How to Solve Divide by Zero Exception in Java
- Divide by Integer Zero Exception in Java
- Resolve Divide by Integer Zero Exception in Java Using Conditional Statements
-
Resolve Divide by Integer Zero Exception in Java Using
Try-Catch
- Resolve Divide by Integer Zero Exception in Java Using Ternary Operator
- Divide by Floating Point Zero Exception in Java
- Resolve Divide by Float Zero Exception in Java Using Conditional Statements
-
Resolve Divide by Float Zero Exception in Java Using
Try-Catch
- Resolve Divide by Float Zero Exception in Java Using Ternary Operator
- Conclusion
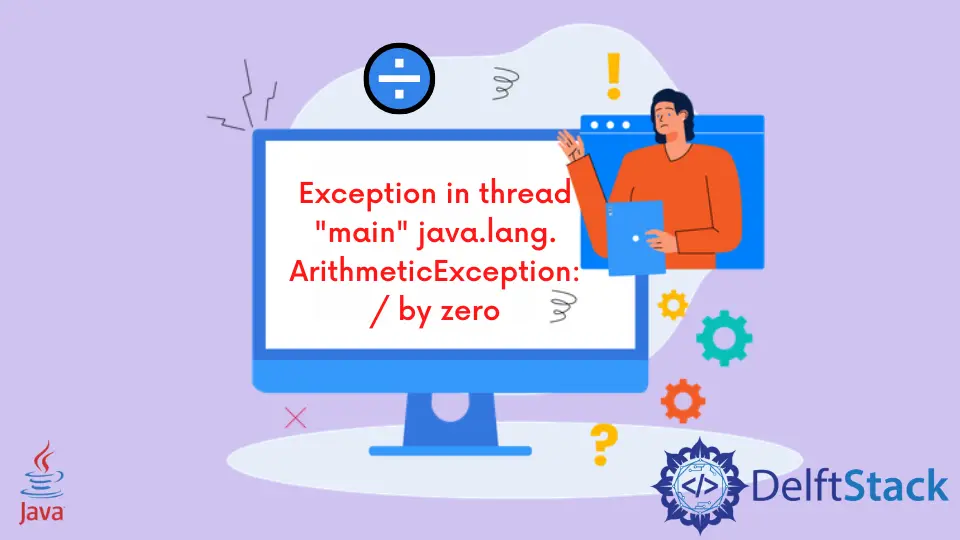
This article will demonstrate what happens in a Java program when dividing by zero or float zero and how to resolve its errors.
In Java, an ArithmeticException
is a type of runtime exception that occurs when an arithmetic operation is attempted, but the result is undefined or not representable. Handling ArithmeticException
is crucial to prevent unexpected program termination and to provide a more controlled response to arithmetic errors during runtime.
Therefore, it becomes imperative for Java developers to implement robust error-handling mechanisms, especially when dealing with mathematical operations that involve division.
Divide by Integer Zero Exception in Java
ArithmeticException
in Java is thrown when an illegal arithmetic operation occurs, such as dividing an integer by zero. It signals errors in arithmetic calculations, preventing unexpected program termination by providing a controlled response to arithmetic errors during runtime.
Common scenarios leading to an ArithmeticException
include dividing an integer by zero (/ 0
) or attempting to calculate the remainder of a division by zero. Essentially, it indicates an error in arithmetic calculations, signaling that the operation is not valid in the given context.
Certainly, here’s an example code that performs a division by zero without catching the ArithmeticException
:
public class ArithmeticExceptionExample {
public static void main(String[] args) {
int numerator = 10;
int denominator = 0;
// Division by zero (ArithmeticException will occur)
int result = numerator / denominator;
// This line will not be reached due to the exception
System.out.println("Result: " + result);
}
}
In this code snippet, the program attempts to divide numerator
by zero (denominator = 0
). As a result, an ArithmeticException
is thrown during runtime.
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero
at ArithmeticExceptionExample.main(ArithmeticExceptionExample.java:7)
Since there is no try-catch
block to handle this exception, the program will terminate abruptly, and the line to print the result will not be executed. It’s crucial to implement proper exception handling to prevent such unexpected terminations in a real-world application.
Resolve Divide by Integer Zero Exception in Java Using Conditional Statements
Conditional statements are crucial in resolving divide-by-zero scenarios as they provide a mechanism to check whether the denominator is zero before attempting the division operation. Without these checks, attempting to divide an integer by zero would lead to an ArithmeticException
, resulting in runtime errors and potential program crashes.
Using conditional statements, developers can proactively prevent such exceptions, handle edge cases, and provide graceful error messages or alternative actions, contributing to more robust and reliable software.
Consider a straightforward scenario where a user inputs two integers, and our program attempts to perform division. To preemptively handle the possibility of division by zero, we’ll employ conditional checks.
Below is the complete working code example:
import java.util.Scanner;
public class DivideByZeroHandler {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the numerator: ");
int numerator = scanner.nextInt();
System.out.println("Enter the denominator: ");
int denominator = scanner.nextInt();
if (denominator != 0) {
int result = divideNumbers(numerator, denominator);
System.out.println("Result: " + result);
} else {
System.out.println("Error: Cannot divide by zero");
}
}
private static int divideNumbers(int numerator, int denominator) {
return numerator / denominator;
}
}
In the code, we begin by importing the Scanner
class to enable user input. The main
method acts as the program’s entry point, and a Scanner
object is created to facilitate user input.
The user is prompted to input values for the numerator and denominator.
The code includes a conditional check using an if
statement to ensure that the denominator is not equal to zero before performing the division operation. If the condition is true, the division logic is encapsulated within a separate method to enhance code modularity.
This approach allows for a clear and organized structure, promoting readability and maintainability in the code.
In this scenario, the user input consists of a numerator of 10 and a denominator of 0. This creates a division by zero situation, which can lead to a runtime error.
Output:
Enter the numerator:
10
Enter the denominator:
0
Error: Cannot divide by zero
By incorporating conditional checks, we’ve implemented a defensive programming strategy to handle potential divide by zero scenarios. This approach allows us to gracefully manage the division operation and provide informative error messages when necessary.
As expected, the program detects the zero denominator through the conditional check and outputs a meaningful error message, demonstrating the effectiveness of using conditional checks to handle divide by zero scenarios in Java.
Resolve Divide by Integer Zero Exception in Java Using Try-Catch
Using a single try-catch
block is important in resolving divide-by-zero scenarios because it allows for centralized exception handling. When performing a division operation, placing it within a try
block enables the program to catch the potential ArithmeticException
that may arise when dividing by zero.
This approach helps prevent abrupt program termination and allows developers to handle the exception in a controlled manner.
By encapsulating the division operation within a try-catch
block, developers can implement specific error-handling logic or provide meaningful error messages to users. It promotes cleaner code by consolidating exception handling in one location, making it easier to maintain and understand.
Additionally, this approach enhances the overall reliability of the program by ensuring that division by zero does not lead to unexpected crashes, offering a more graceful and controlled response to errors.
Let’s consider a simple scenario where a user inputs two integers, and our program attempts to perform division. To handle the possibility of division by zero, we’ll use a try-catch
block.
Below is the complete working code example:
import java.util.Scanner;
public class DivideByZeroHandler {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the numerator: ");
int numerator = scanner.nextInt();
System.out.println("Enter the denominator: ");
int denominator = scanner.nextInt();
try {
int result = divideNumbers(numerator, denominator);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: Cannot divide by zero");
}
}
private static int divideNumbers(int numerator, int denominator) {
return numerator / denominator;
}
}
We begin by importing the Scanner
class to facilitate user input. The program starts with the main
method, serving as the entry point.
A Scanner
object is created to read user input, and the user is prompted to enter values for the numerator and denominator. The critical division operation is encapsulated within a try-catch
block, providing a mechanism to catch and handle ArithmeticException
if a division by zero occurs.
Additionally, the division logic is encapsulated in a separate method for improved modularity, contributing to a clear and organized code structure.
This design enhances readability and maintainability, promoting effective error handling in the program.
In this scenario, the user input consists of a numerator of 10 and a denominator of 0. This creates a division by zero situation, which can lead to a runtime error.
Output:
Enter the numerator:
10
Enter the denominator:
0
Error: Cannot divide by zero
By employing the try-catch
method, we’ve created a robust program that gracefully handles the potential divide by zero scenario. The catch
block ensures that even if an exception occurs, the program doesn’t crash abruptly, providing a more user-friendly experience.
As expected, the program catches the exception and outputs a meaningful error message, preventing the division by zero from causing a runtime failure. This illustrates the effectiveness of using try-catch
for handling divide by zero scenarios in Java.
Resolve Divide by Integer Zero Exception in Java Using Ternary Operator
The ternary operator in Java can be useful in resolving divide-by-zero scenarios by providing a concise and readable way to handle conditions. Specifically, when checking if the denominator is zero before performing a division operation, the ternary operator allows developers to express this condition in a single line of code.
This can lead to cleaner and more maintainable code, especially when dealing with straightforward conditions like checking for division by zero.
Let’s consider a scenario where a user inputs two integers, and our program aims to perform division while gracefully handling the possibility of division by zero. The ternary operator, a compact conditional expression, provides a concise way to achieve this.
Below is the complete working code example:
import java.util.Scanner;
public class DivideByZeroHandler {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the numerator: ");
int numerator = scanner.nextInt();
System.out.println("Enter the denominator: ");
int denominator = scanner.nextInt();
int result = (denominator != 0) ? divideNumbers(numerator, denominator) : 0;
System.out.println((denominator != 0) ? "Result: " + result : "Error: Cannot divide by zero");
}
private static int divideNumbers(int numerator, int denominator) {
return numerator / denominator;
}
}
We begin by importing the Scanner
class for handling user input in our program. The main
method serves as the entry point, and a Scanner
object is created to collect user input.
Users are prompted to input values for the numerator and denominator. The ternary operator is then employed to conditionally perform the division only when the denominator is not zero.
If the condition is true, the divideNumbers
method is called; otherwise, the result is set to zero. Similarly, the ternary operator is used for output, printing the result if the denominator is not zero; otherwise, an error message is displayed.
The division logic is encapsulated in a separate method, promoting code modularity for a clearer and more organized structure.
In this scenario, the user input consists of a numerator of 10 and a denominator of 0. This creates a division by zero situation, which can lead to a runtime error.
Output:
Enter the numerator:
10
Enter the denominator:
0
Error: Cannot divide by zero
As expected, the program utilizes the ternary operator to gracefully handle the division by zero scenarios, providing a clear error message without compromising code conciseness. This demonstrates the effectiveness of using the ternary operator to handle divide by zero scenarios in Java.
The use of the ternary operator provides a concise and readable solution for handling divide by zero scenarios. It condenses the conditional checks and expressions into a single line, making the code more elegant and expressive.
Divide by Floating Point Zero Exception in Java
In Java, the ability to divide by float zero is allowed according to the IEEE 754 floating-point standard, which specifies the behavior of floating-point arithmetic. The standard defines two special values related to division by zero: positive and negative infinity.
When you divide a non-zero floating-point number by zero, Java represents the result as positive or negative infinity, depending on the sign of the numerator. For example:
import java.util.*;
public class Main {
public static void main(String[] args) {
float numerator = 1.0f;
float denominator = 0.0f;
float result = numerator / denominator;
System.out.println("Result: " + result); // Output: Result: Infinity
}
}
In this case, the result
will be positive infinity.
Output:
Result: Infinity
If you explicitly attempt to divide zero by zero, the result is NaN
(Not a Number), indicating an undefined or indeterminate value:
import java.util.*;
public class Main {
public static void main(String[] args) {
float numerator = 0.0f;
float denominator = 0.0f;
float result = numerator / denominator;
System.out.println("Result: " + result); // Output: Result: NaN
}
}
Output:
Result: NaN
While Java allows these operations to conform to the IEEE 754 standard, it’s essential to handle the results appropriately in your code, considering cases where infinity or NaN
may be encountered.
Resolve Divide by Float Zero Exception in Java Using Conditional Statements
Conditional statements, like an if
check, ensure that the denominator is not zero before attempting division, avoiding ArithmeticException
. This proactive approach safeguards the program from unexpected crashes, providing a controlled response to potential errors.
It enhances code reliability by allowing developers to handle edge cases gracefully, ensuring smooth program execution even in challenging scenarios.
Consider a scenario where a program prompts a user to input two floating-point numbers and performs division. The challenge lies in handling the potential division by floating-point zero.
Let’s delve into the code example to understand how conditional checks can effectively address this issue.
import java.util.Scanner;
public class FloatDivisionHandler {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// User input for numerator
System.out.println("Enter the numerator: ");
float numerator = scanner.nextFloat();
// User input for denominator
System.out.println("Enter the denominator: ");
float denominator = scanner.nextFloat();
// Conditional check to handle division by floating-point zero
if (denominator != 0.0f) {
float result = divideNumbers(numerator, denominator);
System.out.println("Result: " + result);
} else {
System.out.println("Error: Cannot divide by floating-point zero");
}
}
// Method to perform division
private static float divideNumbers(float numerator, float denominator) {
return numerator / denominator;
}
}
The program initiates by prompting the user to input two floating-point numbers, namely the numerator and denominator, utilizing the Scanner
class for data collection.
Subsequently, a pivotal conditional check is implemented using an if
statement to ensure the denominator is not zero, thus preventing division by floating-point zero. If the condition holds true, the program proceeds to execute the division operation; otherwise, it displays an error message.
In order to enhance modularity and code organization, the division logic is encapsulated within a dedicated method called divideNumbers
.
In this scenario, the user inputs a numerator of 10.0 and a denominator of 0.0, resulting in a division by zero situation.
Output:
Enter the numerator:
10.0
Enter the denominator:
0.0
Error: Cannot divide by floating-point zero
Handling division by floating-point zero in Java using conditional checks ensures a robust and controlled approach to potential errors. The code exemplifies a user-friendly way to deal with such scenarios, providing informative error messages and preventing unexpected program crashes.
This method promotes code reliability, making it a valuable technique in Java programming.
The program effectively catches the division by floating-point zero through the conditional check, providing a clear and informative error message. This demonstrates the efficacy of using conditional checks to handle such scenarios in Java.
Resolve Divide by Float Zero Exception in Java Using Try-Catch
Resolving divide by float zero using a single try-catch
block in Java is essential for streamlined error handling. It simplifies code, centralizing exception management.
The try-catch
structure efficiently catches and handles potential ArithmeticException
due to division by zero, preventing program crashes. This approach enhances code readability and maintainability by consolidating error-handling logic, resulting in more concise and organized code.
Let’s consider a practical example where a Java program prompts a user to input two floating-point numbers and performs division. The focus is on using a single try-catch
block to manage the potential division by floating-point zero.
import java.util.Scanner;
public class FloatDivisionHandler {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// User input for numerator
System.out.println("Enter the numerator: ");
float numerator = scanner.nextFloat();
// User input for denominator
System.out.println("Enter the denominator: ");
float denominator = scanner.nextFloat();
try {
// Attempt to perform division
float result = divideNumbers(numerator, denominator);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
// Catch and handle ArithmeticException (division by zero)
System.out.println("Error: " + e.getMessage());
}
}
// Method to perform division
private static float divideNumbers(float numerator, float denominator) {
// Check for division by zero and throw ArithmeticException
if (denominator == 0.0f) {
throw new ArithmeticException("Cannot divide by floating-point zero");
}
return numerator / denominator;
}
}
In the initial step of the program, users are prompted to input two floating-point numbers: the numerator and the denominator.
The Scanner
class is employed to efficiently collect these values for subsequent processing.
The distinctive aspect of the approach lies in the utilization of a single try-catch
block, replacing traditional conditional checks. This singular block encapsulates the division operation, streamlining the code and consolidating error handling into a centralized structure.
Within the dedicated division method (divideNumbers
), a check is implemented to ensure the denominator is not zero.
In the event of a zero denominator, an ArithmeticException
is promptly thrown, accompanied by a customized error message. This methodical approach enhances code readability and maintains a concise structure.
In this scenario, the user inputs a numerator of 10.0 and a denominator of 0.0, resulting in a division by zero situation.
Output:
Enter the numerator:
10.0
Enter the denominator:
0.0
Error: Cannot divide by floating-point zero
Employing a single try-catch
block to handle division by floating-point zero in Java brings clarity and conciseness to error management. This method simplifies the code structure, making it more readable and maintainable.
By throwing and catching an ArithmeticException
, developers can efficiently manage division-related errors, enhancing the robustness of their applications.
The program effectively catches the division by floating-point zero using the single try-catch
block, providing a clear and informative error message. This demonstrates the effectiveness of using this approach to handle such scenarios in Java.
Resolve Divide by Float Zero Exception in Java Using Ternary Operator
The ternary operator allows developers to handle the division scenario elegantly in a single line, improving code efficiency. It provides a clear and compact representation of the condition, making the code more expressive.
This approach enhances code maintainability and readability by succinctly addressing potential errors, ensuring a streamlined and effective way to manage division by floating-point zero.
Let’s delve into a practical example where a Java program prompts users to input two floating-point numbers and performs division. The focus here is on employing the ternary operator to efficiently handle the possibility of division by floating-point zero.
import java.util.Scanner;
public class FloatDivisionHandler {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// User input for numerator
System.out.println("Enter the numerator: ");
float numerator = scanner.nextFloat();
// User input for denominator
System.out.println("Enter the denominator: ");
float denominator = scanner.nextFloat();
// Using ternary operator to handle division by floating-point zero
float result = (denominator != 0.0f) ? divideNumbers(numerator, denominator) : 0.0f;
// Displaying the result
System.out.println("Result: " + result);
}
// Method to perform division
private static float divideNumbers(float numerator, float denominator) {
return numerator / denominator;
}
}
In the program, users are prompted to input two floating-point numbers, the numerator and the denominator, using the Scanner
class.
The core of the approach involves using the ternary operator in a single line of code to check if the denominator is not equal to zero. If the condition holds true, the program proceeds with the division operation; otherwise, it assigns a default value of 0.0f
to the result.
The division logic is encapsulated in the divideNumbers
method, ensuring a modular and organized structure for executing the division based on the provided values.
In this scenario, the user inputs a numerator of 10.0 and a denominator of 0.0, resulting in a division by zero situation.
Output:
Enter the numerator:
10.0
Enter the denominator:
0.0
Result: 0.0
Using the ternary operator to handle division by floating-point zero in Java introduces an elegant and concise approach. This method not only simplifies the code but also enhances readability and maintains a streamlined structure.
The single line of code encapsulates the essence of error handling, making the program more robust.
The program effectively utilizes the ternary operator to handle division by floating-point zero, providing a clear and concise output. This demonstrates the effectiveness of leveraging the ternary operator in Java to manage potential errors in division scenarios.
Conclusion
Handling arithmetic exceptions, specifically division by zero is crucial for maintaining the stability and reliability of Java programs.
Using conditional statements, developers can proactively check and prevent division by zero, ensuring smooth execution and preventing unexpected crashes.
Alternatively, employing a try-catch
block allows for centralized error handling, catching arithmetic exceptions when they occur, and providing an opportunity to handle them gracefully.
Ternary operators offer a concise and expressive way to handle such scenarios in a single line of code, contributing to code readability and efficiency.
Each method—conditional statements, try-catch
blocks, and ternary operators—provides a valuable approach to resolving divide by zero issues, allowing developers to choose the most suitable strategy based on their coding preferences and the specific requirements of their applications.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn