Difference Between StringBuilder and StringBuffer in Java
-
the
StringBuffer
in Java -
the
StringBuilder
in Java -
Differences Between
StringBuilder
andStringBuffer
in Java
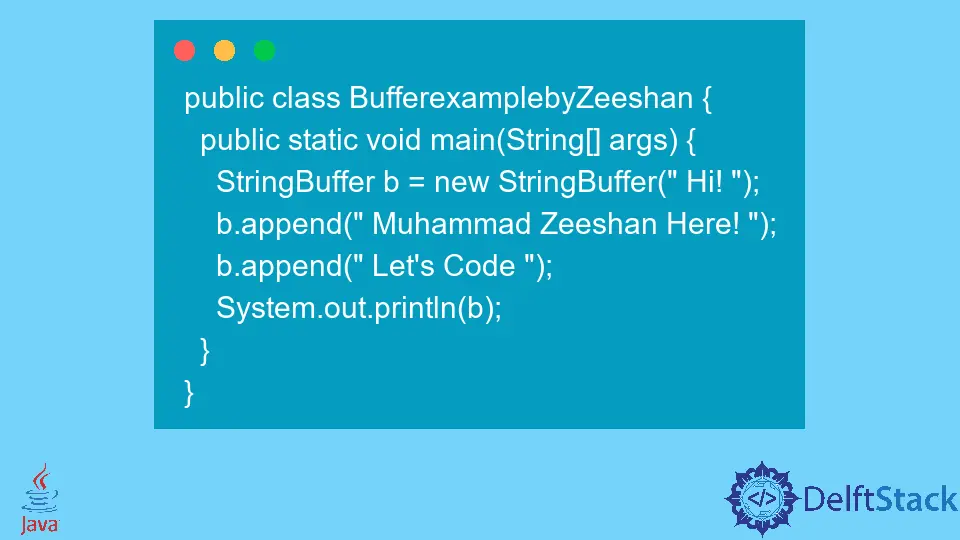
This tutorial will discuss the differences between Java’s StringBuilder
and StringBuffer
classes. So let’s get started!
the StringBuffer
in Java
The StringBuffer
class gives us a means by which we can use mutable string data in Java. These strings may be utilized in an unconcerned manner by numerous threads at the same time without risk.
Syntax:
StringBuffer b = new StringBuffer("Shanii");
Example:
public class BufferexamplebyZeeshan {
public static void main(String[] args) {
StringBuffer b = new StringBuffer(" Hi! ");
b.append(" Muhammad Zeeshan Here! ");
b.append(" Let's Code ");
System.out.println(b);
}
}
Output:
Hi! Muhammad Zeeshan Here! Let's Code
the StringBuilder
in Java
StringBuilder
also gives us access to mutable strings, though this implementation has no thread safety. Numerous threads can’t utilize it at the same time.
Because this class does not apply this additional functionality, it is much quicker than StringBuffer
.
Syntax:
StringBuilder s1 = new StringBuilder("Shani");
Example:
public class BuilderexamplebyZeeshan {
public static void main(String[] args) {
StringBuilder s = new StringBuilder(" Hey!");
s.append("I am a Programmer");
System.out.println(s);
}
}
Output:
Hey!I am a Programmer
Differences Between StringBuilder
and StringBuffer
in Java
Let’s see the differences between StringBuilder
and StringBuffer
by the below statements:
No. | StringBuilder |
StringBuffer |
---|---|---|
1 | The StringBuilder function is not synchronized, meaning it is not thread-safe. This indicates that two different threads can simultaneously call the methods of the StringBuilder class. |
The StringBuffer object is synchronized, which means it is thread-safe. It signifies that the methods of StringBuffer cannot be called concurrently by two different threads. |
2 | It is generated in the heap memory of the computer. |
It is also generated in the heap memory. |
3 | Offers us strings that are Mutable . |
We can change string without constructing an object. |
4 | It can store a default of 16 characters. | Like StringBuilder , it can store a default of 16 characters. |
5 | The quickest of them all since it supports mutability while preventing many threads from working simultaneously. | It is slower than the StringBuilder class since it enables several threads to perform actions simultaneously. |
6 | The concatenation is done using the append() function. |
Its concatenation is also done using the append() function. |
Example Using StringBuilder
and StringBuffer
StringBuilder
is quicker than StringBuffer
since it’s not synchronized. Let’s have a look at a basic example to compare both StringBuilder
and StringBuffer
:
public class Main {
public static void main(String[] args) {
int RandomNumber = 5677839;
long time;
StringBuffer s = new StringBuffer();
time = System.currentTimeMillis();
for (int i = RandomNumber; i-- > 0;) {
s.append("");
}
System.out.println(System.currentTimeMillis() - time);
StringBuilder b = new StringBuilder();
time = System.currentTimeMillis();
for (int i = RandomNumber; i > 0; i--) {
b.append("");
}
System.out.println(System.currentTimeMillis() - time);
}
}
Output:
161
17
The above example provides the numerical comparison of 161
milliseconds for StringBuffer
and 17
milliseconds for StringBuilder
, which makes it quite evident that StringBuilder
is the more efficient option.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn