How to Delete an Object in Java
-
Delete an
Object
in Java Usingnull
Reference - Delete an Object in Java by Assigning to Another Object
- Delete an Object in Java by Exiting a Method or Scope
-
Delete an Object in Java Using
System.gc()
(Garbage Collection) - Delete an Object in Java by Clearing References in Data Structures
- Conclusion
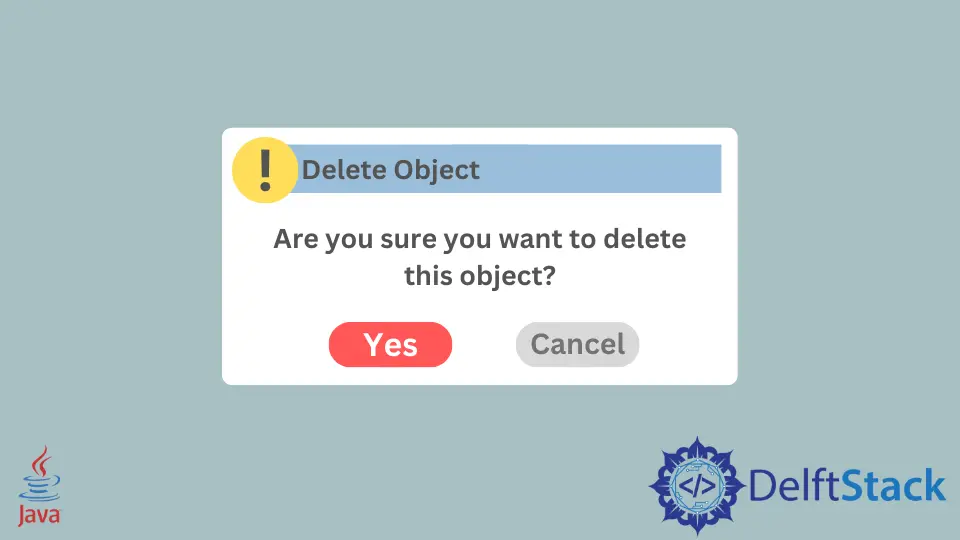
Java is well-known for its robust memory management capabilities, providing developers with a variety of methods to efficiently delete objects. In the complex life cycle of Java applications, effective memory management is crucial for achieving peak performance.
This article looks at five different ways to delete things, explaining how they work and when to use them. From the basic null
assignment to the more advanced garbage collection, we’ll dive into each method and see how it affects memory.
The aim here is to help developers choose the best way for their program’s specific needs, making sure the code stays neat and easy to manage.
Delete an Object
in Java Using null
Reference
In this section, we’ll explore the concept of deleting an object using the null
reference and understand the implications of such an operation.
In Java, the null
reference is a special value that can be assigned to an object variable.
When an object variable is assigned the value null
, it means that the variable no longer points to any object in memory. Essentially, it indicates the absence of an object.
To delete an object in Java using the null
reference, you simply assign null
to the variable that references the object.
Syntax:
myObject = null;
myObject
: The object to be deleted.null
: Assigning this to an object indicates that the object it is assigned to no longer exists, in this casemyObject
.
To understand better, let us look at an example code that utilizes the null
reference to delete an object in Java.
public class ObjectDeletionExample {
public static void main(String[] args) {
// Creating an object
MyClass myObject = new MyClass("Sample Object");
// Displaying the object before deletion
System.out.println("Object before deletion: " + myObject);
// Deleting the object using null
myObject = null;
// Displaying the object after deletion
System.out.println("Object after deletion: " + myObject);
}
static class MyClass {
String name;
MyClass(String name) {
this.name = name;
}
@Override
public String toString() {
return "MyClass{"
+ "name='" + name + '\'' + '}';
}
}
}
Let’s break down the code to understand how deleting an object using null
works.
We first create an instance of the MyClass
class named myObject
and initialize it with the name Sample Object
. Before deleting the object, we print its state using the toString
method of the MyClass
class.
Next, the crucial step - setting the reference myObject
to null
. This essentially detaches the reference from the actual object, making the object eligible for garbage collection.
After setting the reference to null
, we print the state of the object again. Since the reference is now null
, the object effectively no longer exists from a reference standpoint.
The output demonstrates that after setting the reference to null
, the object is effectively removed, and attempting to access it results in null
.
Output:
Object before deletion: MyClass{name='Sample Object'}
Object after deletion: null
Deleting an object using null
in Java is a simple yet powerful technique. It allows developers to explicitly release an object, signaling to the garbage collector that the memory occupied by the object can be reclaimed.
However, it’s essential to use this technique judiciously, as overusing it can lead to null pointer exceptions. In most cases, relying on Java’s automatic garbage collection is sufficient, but understanding explicit deletion can be beneficial in specific scenarios.
Delete an Object in Java by Assigning to Another Object
This section explores the technique of deleting an object in Java by assigning it to another object. Understanding this concept is beneficial for developers seeking fine-grained control over memory resources in situations where immediate garbage collection is necessary.
The concept of deleting an object in Java by assigning it to another object typically involves reassigning the reference variable to a different object or null
.
Syntax:
firstObject = secondObject;
firstObject
: This is the object to be deleted.secondObject
: This object is assigned to thefirstObject
to delete it.
Let’s create a simple example to demonstrate deleting an object in Java by assigning it to another object:
public class ObjectDeletionExample {
public static void main(String[] args) {
// Creating two objects
MyClass firstObject = new MyClass("Object 1");
MyClass secondObject = new MyClass("Object 2");
// Displaying the state before deletion
System.out.println("Before Deletion:");
System.out.println("First Object: " + firstObject.getName());
System.out.println("Second Object: " + secondObject.getName());
secondObject = null;
// Deleting the first object by assigning the second object
firstObject = secondObject;
// Displaying the state after deletion
System.out.println("\nAfter Deletion:");
// Now, firstObject points to the same object as secondObject
System.out.println("First Object: " + firstObject);
System.out.println("Second Object: " + secondObject);
}
}
class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
In this example, we have a simple MyClass
representing objects with a name.
Then, the two objects (firstObject
and secondObject
) are created. The state of both objects is displayed before deletion.
The firstObject
is deleted by assigning it the value of secondObject
which is null
. The state of the objects is displayed after deletion, showing that firstObject
now points to the same object as secondObject
(null
).
Output:
Before Deletion:
First Object: Object 1
Second Object: Object 2
After Deletion:
First Object: null
Second Object: null
Keep in mind that this operation doesn’t immediately free up memory; the actual cleanup occurs when the Java garbage collector runs and identifies unreferenced objects.
Delete an Object in Java by Exiting a Method or Scope
This section focuses on deleting an object by exiting a method or scope. This is a convenient way as local variables are automatically eligible for garbage collection when their scope ends.
Let’s consider a simple scenario where we have a resource-intensive object that we want to release as soon as it’s no longer needed. Here’s a complete working example:
public class ObjectDeletionExample {
public static void main(String[] args) {
processObject();
System.out.println("Main method finished");
}
private static void processObject() {
// Create a resource-intensive object
HeavyResourceObject resourceObject = new HeavyResourceObject();
// Perform some operations with the object
System.out.println("Processing the object...");
// Explicitly delete the object by exiting the method
return; // This effectively ends the method, and the object becomes eligible for garbage
// collection
}
private static class HeavyResourceObject {
// Simulating a resource-intensive object
// ...
}
}
In the ObjectDeletionExample
class, we have a main
method that calls the processObject
method. Inside processObject
, we create an instance of the HeavyResourceObject
, which simulates a resource-intensive object.
After performing some operations with the object, we want to release it before the method completes. By using the return
statement without any value, we exit the processObject
method prematurely, making the resourceObject
eligible for garbage collection.
This approach is particularly useful when the heavy resource object consumes substantial memory, and we want to release it as soon as it’s no longer needed, rather than waiting for the method or scope to naturally complete.
Output:
Processing the object...
Main method finished
This output indicates that the processObject
method was exited prematurely, and the subsequent message in the main
method is printed. The premature exit of the processObject
method triggers the object deletion, demonstrating the effectiveness of this approach in managing memory efficiently.
In conclusion, the technique of deleting an object by exiting a method or scope in Java provides a practical way to release resources promptly. It’s crucial for scenarios where memory optimization is essential, and objects need to be explicitly deallocated.
By incorporating this concept into your Java programming practices, you can enhance the efficiency of your applications and ensure better resource management.
Delete an Object in Java Using System.gc()
(Garbage Collection)
The System.gc()
method, though not a guarantee of immediate garbage collection, can be employed to suggest to the JVM that it’s an opportune time to perform garbage collection.
Before we dive into using System.gc()
, let’s understand the significance of garbage collection in Java. When objects are created during the execution of a program, they occupy memory.
Over time, some of these objects may no longer be in use, but their memory is still reserved. The Garbage Collector
identifies and removes these unused objects, freeing up memory and preventing memory leaks.
However, Java’s Garbage Collector
operates on its schedule, and developers might encounter situations where they want to expedite the removal of an object. This is where System.gc()
comes into play.
Consider a scenario where we have a class Book
that represents a book with a title and an author. We want to demonstrate how to use System.gc()
to suggest garbage collection when a book is no longer needed.
Example:
public class Book {
private String title;
private String author;
public Book(String title, String author) {
this.title = title;
this.author = author;
}
public void displayInfo() {
System.out.println("Title: " + title);
System.out.println("Author: " + author);
}
public static void main(String[] args) {
// Create a Book object
Book myBook = new Book("Java Programming", "John Doe");
// Display book information
myBook.displayInfo();
// Suggest garbage collection using System.gc()
System.gc();
}
}
In this code snippet, we define a simple Book
class with private fields for title and author. A constructor initializes the title and author when a new Book
object is created, then the displayInfo()
method prints the book information.
Inside the main
method, we create an instance of the Book
class named myBook
. We then call the displayInfo()
method to print the book information.
After displaying the book information, we invoke System.gc()
to suggest to the JVM that it may be a good time to perform garbage collection.
Output:
Title: Java Programming
Author: John Doe
The reason why the output is like that is because calling System.gc()
only suggests garbage collection; it doesn’t guarantee immediate execution. The Garbage Collector
will decide when to run based on various factors, such as available memory and system load.
In Java, the Garbage Collector
automates memory management, but there are scenarios where developers may want to provide hints for efficient memory usage. The System.gc()
method is a tool for suggesting garbage collection, offering developers a level of control over memory resources.
However, it’s essential to understand that the Garbage Collector
operates autonomously, and the use of System.gc()
should be approached with caution, considering the potential impact on performance.
In practice, relying on the automatic garbage collection mechanism is generally sufficient for most applications. Explicitly invoking System.gc()
is a nuanced decision and should be done judiciously, taking into account the specific requirements and performance considerations of the application.
Delete an Object in Java by Clearing References in Data Structures
In this section, we’ll explore the concept of deleting an object in Java by explicitly clearing references in data structures.
Deleting an object in Java by clearing references in data structures involves removing all references to the object from any data structures (such as collections or arrays) that are holding it. When there are no more references to an object, it becomes unreachable and eligible for garbage collection.
The syntax for this operation depends on the specific data structure being used.
Example:
import java.util.ArrayList;
import java.util.List;
public class ObjectDeletionExample {
public static void main(String[] args) {
// Create a list of objects
List<String> objectList = new ArrayList<>();
objectList.add("Object 1");
objectList.add("Object 2");
objectList.add("Object 3");
// Display the list before deletion
System.out.println("Objects in the list before deletion: " + objectList);
// Delete an object by clearing its reference
clearObjectReference(objectList, "Object 2");
// Display the list after deletion
System.out.println("Objects in the list after deletion: " + objectList);
}
private static void clearObjectReference(List<String> list, String objectToDelete) {
// Iterate through the list to find and remove the specified object
for (int i = 0; i < list.size(); i++) {
if (list.get(i).equals(objectToDelete)) {
list.set(i, null); // Clear the reference to the object
break;
}
}
}
}
Let’s break down the code step by step to understand the process of deleting an object by clearing references.
We start by creating a List
of String
objects named objectList
using the ArrayList
implementation. Then, three strings (Object 1
, Object 2
, and Object 3
) are added to the list to simulate a collection of objects.
We print the contents of the list before any object deletion to visualize its initial state.
Then, the clearObjectReference
method takes the list and the object to be deleted as parameters. It iterates through the list and identifies the specified object; once found, it sets the reference to null
, effectively clearing the reference to the object.
We print the contents of the list after deleting the specified object to observe the change.
Output:
Objects in the list before deletion: [Object 1, Object 2, Object 3]
Objects in the list after deletion: [Object 1, null, Object 3]
The output of the code demonstrates the removal of the specified object.
Clearing references in data structures is a valuable technique in Java for managing memory efficiently. By explicitly setting references to null
when an object is no longer needed, we contribute to the timely release of memory resources.
This practice is particularly crucial in large-scale applications where memory management is a critical aspect of performance optimization. Adopting such practices can help developers build robust and resource-efficient Java applications.
Conclusion
In Java development, it’s important to know various ways to delete objects for efficient applications.
Each method discussed in this article has its advantages, and the best one depends on the project’s needs. With this knowledge, developers can make smart choices, balancing performance and easy-to-manage code.
Java’s flexibility goes beyond just its rules and features; it helps manage memory and gives developers many options to make applications work better.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn