How to Get Year From Date in Java
-
Using
java.time.LocalDate
to Get the Current Year From Date in Java -
Using
java.text.SimpleDateFormat
to Get the Current Year From Date in Java -
Using
java.util.Calendar
to Get the Current Year From Date in Java -
Using the
String.split()
Method to Get the Current Year From Date in Java -
Using
java.util.GregorianCalendar
to Get the Current Year From Date in Java - Conclusion
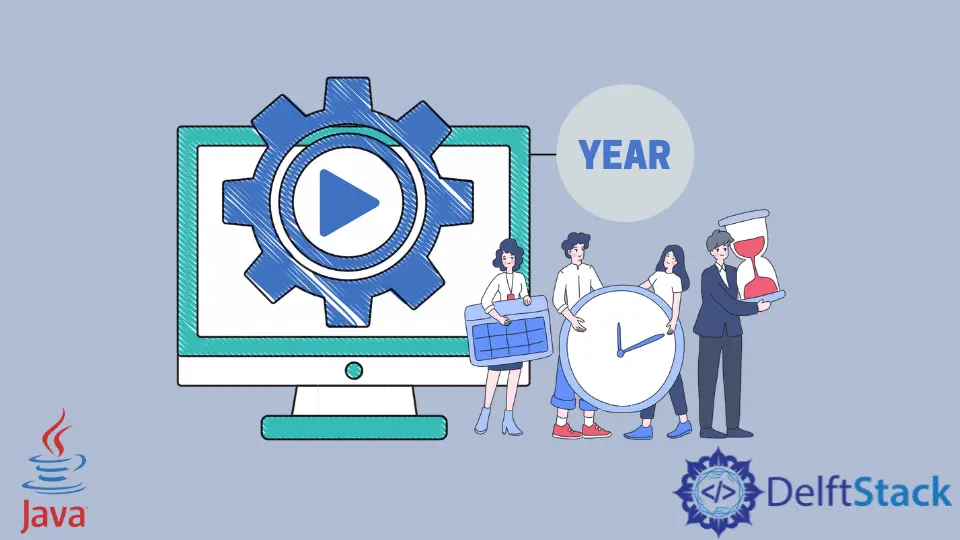
In Java programming, retrieving the current year from a date is a task frequently encountered in applications dealing with temporal data. Whether you are working with legacy code or utilizing the latest features introduced in Java 8 and beyond, there are multiple approaches to achieve this common goal.
This article will explore various methods to extract the current year from a date in Java, ranging from modern solutions like the java.time.LocalDate
class to traditional options like java.util.Calendar
and even unconventional approaches such as using String.split()
.
Using java.time.LocalDate
to Get the Current Year From Date in Java
In Java, the java.time.LocalDate
class provides a modern and efficient way to work with dates. The LocalDate
class, part of the java.time
package introduced in Java 8, represents a date without a time component.
To get the current year, we first need to create a LocalDate
instance using the static now()
method. This method retrieves the current date based on the system clock.
Next, we extract the year from the LocalDate
instance using the getYear()
method. Unlike the deprecated getYear()
method in the older java.util.Date
class, this method returns the actual year without any need for adjustments.
Code Example: Using java.time.LocalDate
to Get the Current Year From Date
Here’s a simple Java program that demonstrates how to obtain the current year from a date using java.time.LocalDate
:
import java.time.LocalDate;
public class CurrentYearFromDate {
public static void main(String[] args) {
LocalDate currentDate = LocalDate.now();
int currentYear = currentDate.getYear();
System.out.println("Current Year: " + currentYear);
}
}
In this example, we start by importing the java.time.LocalDate
class. In the main
method, we create a LocalDate
instance named currentDate
using LocalDate.now()
, which automatically captures the current date based on the system clock.
Next, we extract the current year from the currentDate
instance using the getYear()
method, storing the result in the currentYear
variable.
Output:
This indicates that the code successfully obtained and displayed the current year from the LocalDate
instance.
This approach is straightforward and avoids the pitfalls associated with older date classes. The java.time
package provides a more robust and user-friendly API for handling date and time operations.
Using java.text.SimpleDateFormat
to Get the Current Year From Date in Java
While the java.time.LocalDate
class provides a modern approach for handling dates in Java, some scenarios may still require the use of older classes. The SimpleDateFormat
class, part of the java.text
package, allows us to format and parse dates according to a specified pattern.
To obtain the current year, we create a SimpleDateFormat
instance with the pattern yyyy
, which represents the four-digit year. We then call the format()
method on this instance, passing the Date
object as an argument.
The result is a formatted string containing the current year.
Code Example: Using java.text.SimpleDateFormat
to Get the Current Year From Date
Here’s a Java program demonstrating how to obtain the current year from a date using SimpleDateFormat
:
import java.text.SimpleDateFormat;
import java.util.Date;
public class CurrentYearFromDate {
public static void main(String[] args) {
Date currentDate = new Date();
SimpleDateFormat yearFormat = new SimpleDateFormat("yyyy");
String currentYear = yearFormat.format(currentDate);
System.out.println("Current Year: " + currentYear);
}
}
In this example, we begin by importing the necessary classes: java.text.SimpleDateFormat
and java.util.Date
. In the main
method, we create a Date
instance named currentDate
using new Date()
, representing the current date and time.
Next, we create a SimpleDateFormat
instance named yearFormat
with the pattern yyyy
. This pattern specifies that only the four-digit year should be included in the formatted output.
We then call the format()
method on the yearFormat
instance, passing the currentDate
object as an argument. This operation formats the date into a string containing only the current year, which we store in the currentYear
variable.
Output:
This indicates that the code successfully obtained and displayed the current year using the SimpleDateFormat
class.
While this method is effective, it’s worth noting that the java.time.LocalDate
class remains the recommended choice for modern date and time handling in Java.
Using java.util.Calendar
to Get the Current Year From Date in Java
In situations where more traditional date handling is required, the java.util.Calendar
class can be employed to extract the current year from a Date
object in Java.
The Calendar
class is a versatile utility in Java for working with dates and times. To obtain the current year, we create a Calendar
instance using Calendar.getInstance()
.
We then set the time of the Calendar
object by calling calendar.setTime(date)
, where date
is the Date
object representing the current date and time. Finally, we retrieve the current year using calendar.get(Calendar.YEAR)
.
Code Example: Using java.util.Calendar
to Get the Current Year From Date
Here’s a simple Java program that demonstrates how to obtain the current year from a date using java.util.Calendar
:
import java.util.Calendar;
import java.util.Date;
public class CurrentYearFromDate {
public static void main(String[] args) {
Date currentDate = new Date();
Calendar calendar = Calendar.getInstance();
calendar.setTime(currentDate);
int currentYear = calendar.get(Calendar.YEAR);
System.out.println("Current Year: " + currentYear);
}
}
In this example, we start by importing the necessary classes: java.util.Calendar
and java.util.Date
. In the main
method, we create a Date
instance named currentDate
using new Date()
, representing the current date and time.
Next, we create a Calendar
instance named calendar
using Calendar.getInstance()
. This instance is then configured to represent the same date and time as the currentDate
object by calling calendar.setTime(currentDate)
.
We proceed to extract the current year from the calendar
instance using calendar.get(Calendar.YEAR)
, storing the result in the currentYear
variable.
Output:
This indicates that the code successfully obtained and displayed the current year using the Calendar
class.
While the Calendar
class is an older approach to handling dates, it remains a valid choice in scenarios where more advanced features of the java.time
package are not needed.
Using the String.split()
Method to Get the Current Year From Date in Java
In scenarios where external libraries are restricted and you need a lightweight solution, the String.split()
method can be utilized to extract the current year from a Date
object in Java.
The String.split()
method in Java is typically used to split a string into an array of substrings based on a specified delimiter. The basic syntax is:
String[] result = inputString.split(delimiter);
Here, inputString
is the original string to be split, and delimiter
is the character or regular expression pattern used to determine where to split the string. The method returns an array of substrings.
In this case, we can leverage the fact that the default toString()
method of the Date
class returns a string containing the current date and time.
By splitting this string using the delimiter -
, we can isolate the year component as it appears before the first hyphen. The obtained substring represents the current year.
Code Example: Using String.split()
to Get the Current Year From Date
Let’s consider the following example:
public class YearExtractor {
public static int getCurrentYear(String dateString) {
String[] dateComponents = dateString.split("-");
String yearString = dateComponents[2];
int currentYear = Integer.parseInt(yearString);
return currentYear;
}
public static void main(String[] args) {
String exampleDate = "22-01-2024";
int year = getCurrentYear(exampleDate);
System.out.println("Current Year: " + year);
}
}
In this example, we’ve encapsulated the functionality in a class named YearExtractor
.
The getCurrentYear
method takes a date string as a parameter and extracts the current year using the String.split("-")
expression. This splits the date string into an array of substrings, with the assumption that the year is at the third index (considering the format dd-MM-yyyy
).
The extracted year, represented as a string, is then converted to an integer using Integer.parseInt()
. The method returns the current year as an integer.
In the main
method, an example date string, 22-01-2024
, is used to demonstrate the functionality. The getCurrentYear
method is called, and the result is printed, showcasing the simplicity and efficiency of this approach.
Output:
This indicates that the code successfully obtained and displayed the current year using the String.split()
method.
While this method is less common and not as robust as using dedicated date classes, it provides a simple solution in cases where other options are limited. Keep in mind that this approach is suitable for lightweight scenarios and may not be as reliable in more complex date-handling situations.
Using java.util.GregorianCalendar
to Get the Current Year From Date in Java
For scenarios where fine-grained control over date and time calculations is needed, the java.util.GregorianCalendar
class in Java can be employed.
The GregorianCalendar
class is a more feature-rich implementation than the basic Calendar
class. It provides extensive functionality for date and time calculations.
To obtain the current year, we create a GregorianCalendar
instance using GregorianCalendar.getInstance()
. By setting its time using the calendar.setTime(date)
method, where date
is the Date
object representing the current date and time, we establish a connection between the GregorianCalendar
and the Date
instance.
Finally, we retrieve the current year using calendar.get(Calendar.YEAR)
.
Code Example: Using java.util.GregorianCalendar
to Get the Current Year From Date
Let’s consider an example where we want to extract the current year from the system date using GregorianCalendar
.
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
public class CurrentYearFromDate {
public static void main(String[] args) {
Date currentDate = new Date();
GregorianCalendar calendar = (GregorianCalendar) GregorianCalendar.getInstance();
calendar.setTime(currentDate);
int currentYear = calendar.get(Calendar.YEAR);
System.out.println("Current Year: " + currentYear);
}
}
In this example, a Date
instance named currentDate
is created using new Date()
, representing the current date and time.
Next, we create a GregorianCalendar
instance named calendar
using GregorianCalendar.getInstance()
. By casting it to GregorianCalendar
, we obtain access to the extended functionality of this class.
We then set the time of the calendar
instance to match the currentDate
object by calling calendar.setTime(currentDate)
. We proceed to extract the current year from the calendar
instance using calendar.get(Calendar.YEAR)
, storing the result in the currentYear
variable.
Output:
This indicates that the code successfully obtained and displayed the current year using the GregorianCalendar
class.
While the GregorianCalendar
class is more elaborate, it provides additional features for advanced date and time manipulations. However, for simpler use cases, the java.time.LocalDate
or java.util.Calendar
classes might be more appropriate.
Conclusion
Extracting the current year from a date in Java can be achieved through various approaches, each catering to different requirements and scenarios.
The modern java.time.LocalDate
class offers simplicity and efficiency, making it a recommended choice for contemporary date handling. For scenarios where external libraries are restricted, the java.text.SimpleDateFormat
class and the String.split()
method provide lightweight alternatives.
Traditionalists may still find value in using the java.util.Calendar
class or its more feature-rich counterpart, java.util.GregorianCalendar
, allowing for fine-grained control over date and time calculations.
The choice of method depends on the specific needs of the project, the desired level of simplicity, and the Java version in use. However, you are encouraged to leverage the robust features introduced in Java 8 and later, such as the java.time
package, for modern and efficient date manipulation.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn