Currency Format in Java
- Convert the Currency Using Logical Functions in Java
-
Convert Currency Using the
NumberFormat
Class in Java
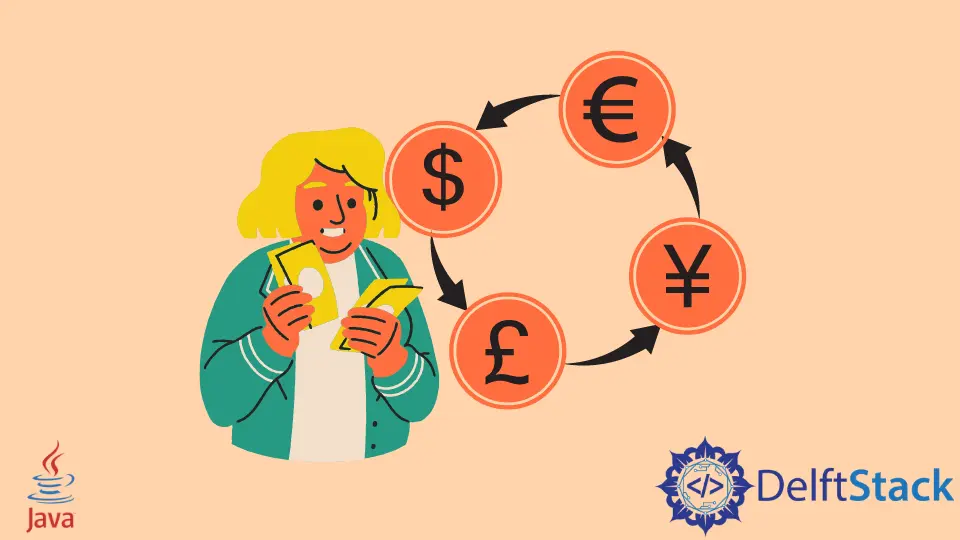
Below are some ways in which we can convert a currency up to desired values in Java.
Convert the Currency Using Logical Functions in Java
public class CurrencyConversion {
public static void main(String[] args) {
double currency_value = 10.9897;
float epsilon = 0.004f;
if (Math.abs(Math.round(currency_value) - currency_value) < epsilon) {
System.out.printf("%10.0f", currency_value);
} else {
System.out.printf("%10.2f", currency_value);
}
}
}
In the above CurrencyConversion
code, there is a variable currency_value
, which holds currency values in decimal format. The epsilon
is another variable that holds a different value. The value can be any float number that performs some logical operations over its difference. The if statement logic is defined that takes the absolute value of the difference between the rounded and actual number. The absolute value gets compared with the epsilon value. The program uses the function Math.abs()
that converts the actual difference of the decimal value to zero or greater than zero.
Based on the output of the conditional statement, it prints in the format as either 10.0f
or 10.2f
. The given two format specifier says, an integral part as 10
, which means that the whole string contains ten characters. And the fractional part specifies the format till 2
decimal places.
In the below console log, it prints an absolute converted value up to 2 decimal places.
10.99 //10.9897
10 //10
10 //10.0008
In the first case, when the currency value is 10.9897
, it gets rounded to 10.99
. Also, When the currency value gets changed to 10
, the output still returns 10
, as nothing rounds off in the input currency. And when the currency value gets changed to 10.0008
, the amount is rounded off to 10
value.
Convert Currency Using the NumberFormat
Class in Java
import java.text.NumberFormat;
public class CurrencyConversion {
public static void main(String[] args) {
double money = 100.1;
NumberFormat formatter = NumberFormat.getCurrencyInstance();
String moneyString = formatter.format(money);
System.out.println(moneyString);
}
}
The above code block uses the NumberFormat
class that calls a static method the getCurrencyInstance
function. The method returns the format’s currency format in the default locale. It also takes a locale value to specify a region. And the currency instances can be in the Rupees
Dollar
format based on the area or region defined. Now the formatter instance is used to call a format method. The format
method takes a parameter double money
and returns a String
value. The function can throw ArithmeticException
when the rounding happens improperly.
The above code creates the following output.
Rs.100.10
But it can be different when the locale value is different. The output can be ¥
when the locale value is Locale.CHINA
. Or it returns $
when the Locale is Locale.CANADA
.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn