How to Declare a Constant String in Java
- Importance of Constants in Java
-
Declaring Constants in Java Using
static final
Modifiers - Declaring Constants in Java Using Interfaces
-
Declaring Constants in Java Using
Enums
- Best Practices in Declaring Constants
- Conclusion
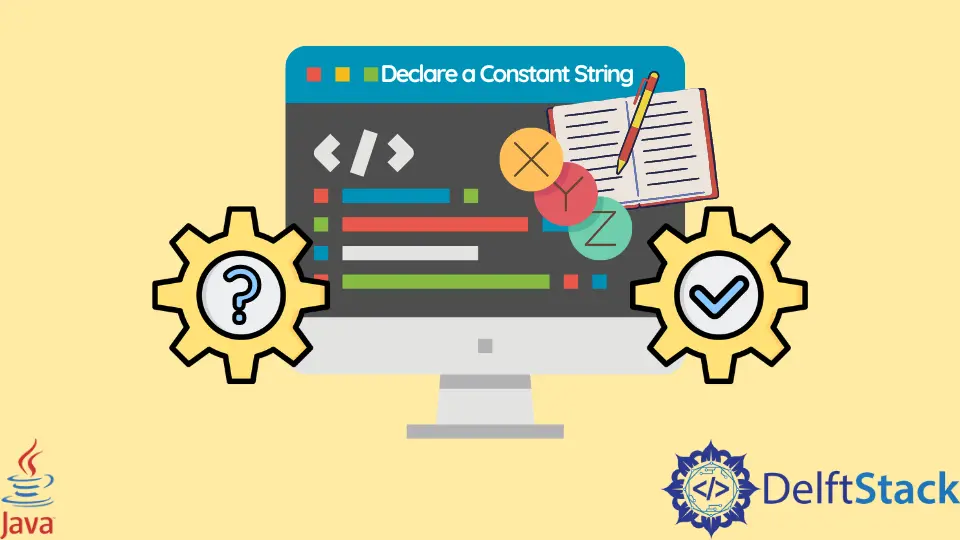
This article provides a comprehensive overview of the significance of constants in Java, explores diverse methods for their declaration, and outlines key best practices to ensure optimal code organization and maintainability.
Importance of Constants in Java
Constants in Java provide stable, unchanging values crucial for code clarity, readability, and maintenance. They enhance program understanding, minimize errors, and enable easy updates by centralizing variable values.
Constants, serving as named references, contribute to efficient and sustainable Java programming.
Declaring Constants in Java Using static final
Modifiers
Declaring constants using the static final
modifier ensures their immutability and shared accessibility, promoting a consistent value throughout the program. The static final
combination ensures that a variable is both a class variable (shared among all instances of the class) and a constant (unchangeable once assigned).
This approach enhances code clarity, prevents inadvertent modifications, and aligns with best practices for constant declaration in Java.
Consider a scenario where we need to represent mathematical constants like Pi and Euler’s number. We will use a class named MathConstants
to demonstrate the static final
method.
public class ConstantsUsingStaticFinal {
public class MathConstants {
public static final double PI = 3.141592653589793;
public static final double EULER_NUMBER = 2.718281828459045;
}
public static void main(String[] args) {
// Accessing constants through the class
System.out.println("Mathematical Constants:");
System.out.println("Pi: " + MathConstants.PI);
System.out.println("Euler's Number: " + MathConstants.EULER_NUMBER);
}
}
In the class declaration section, a class called MathConstants
is introduced to encapsulate mathematical constants. Utilizing the public static final
modifiers, constants such as PI
and EULER_NUMBER
are declared, emphasizing their immutability.
On to the main method, practical usage of accessing constants from the MathConstants
class is demonstrated. This involves using the class name followed by the constant name.
Subsequently, the values of PI
and EULER_NUMBER
are printed, showcasing the straightforward and effective utilization of the static final
modifiers for constant declaration in Java.
Running the program produces the following output:
Mathematical Constants:
Pi: 3.141592653589793
Euler's Number: 2.718281828459045
The static final
method provides a straightforward and widely adopted approach to declare constants in Java. It ensures that the constants are shared among all instances of the class while remaining unchangeable throughout the program.
In this example, we illustrated how to use this method to declare mathematical constants, showcasing its simplicity and effectiveness. Consider employing the static final
modifiers when defining constants in your Java projects to enhance code clarity and maintainability.
Declaring Constants in Java Using Interfaces
Declaring constants using interfaces in Java enhances code organization by grouping related values. It ensures a clear separation of concerns, promoting a clean and maintainable code structure.
Interfaces provide a flexible and consistent way to represent constants, facilitating readability and easy modifications. This approach stands out for its simplicity and adaptability, offering an effective alternative to other methods.
Let’s consider a simple scenario where we want to declare constants representing colors. We will use an interface named ColorConstants
for this purpose.
public class ConstantsUsingInterface {
public interface ColorConstants {
String RED = "#FF0000";
String GREEN = "#00FF00";
String BLUE = "#0000FF";
String BLACK = "#000000";
String WHITE = "#FFFFFF";
}
public static void main(String[] args) {
// Accessing constants through the interface
System.out.println("Color Constants:");
System.out.println("Red: " + ColorConstants.RED);
System.out.println("Green: " + ColorConstants.GREEN);
System.out.println("Blue: " + ColorConstants.BLUE);
System.out.println("Black: " + ColorConstants.BLACK);
System.out.println("White: " + ColorConstants.WHITE);
}
}
In the interface declaration section, we establish an interface called ColorConstants
to encapsulate constant values representing colors. Within this interface, each color is defined as a constant field.
Shifting our focus to the main method, we provide a practical example of accessing the constants declared in the interface. By employing the interface name (ColorConstants
) followed by the specific constant name, we showcase how each color’s value can be accessed.
The resulting values of these constants are then printed, demonstrating a straightforward approach to utilizing interface-based constants in Java.
Running the program produces the following output:
Color Constants:
Red: #FF0000
Green: #00FF00
Blue: #0000FF
Black: #000000
White: #FFFFFF
Declaring constants in Java using interfaces provides a simple and effective way to group related constant values. While interfaces are commonly associated with abstract methods, their ability to hold constants adds versatility to their usage.
In this example, we illustrated how to use an interface to declare color constants, showcasing the clarity and ease of access that this approach offers. Consider employing this method when you have a set of related constants in your Java projects to enhance code organization and maintainability.
Declaring Constants in Java Using Enums
Enums
in Java are more than just a collection of constant values; they are special data types that can encapsulate a set of related constants and methods. Leveraging enums
for constants provides several benefits, such as type safety, code clarity, and the ability to associate additional information with each constant.
Let’s consider a scenario where we need to represent days of the week as constants in a Java program. We will use an enum
called DaysOfWeek
for this purpose.
public class ConstantsUsingEnum {
public enum DaysOfWeek {
SUNDAY("Sun"),
MONDAY("Mon"),
TUESDAY("Tue"),
WEDNESDAY("Wed"),
THURSDAY("Thu"),
FRIDAY("Fri"),
SATURDAY("Sat");
private final String abbreviation;
DaysOfWeek(String abbreviation) {
this.abbreviation = abbreviation;
}
public String getAbbreviation() {
return abbreviation;
}
}
public static void main(String[] args) {
// Accessing enum constants
System.out.println("Days of the Week:");
for (DaysOfWeek day : DaysOfWeek.values()) {
System.out.println(day + ": " + day.getAbbreviation());
}
}
}
In the enum
declaration section, we create an enum
named DaysOfWeek
to represent the days of the week. Each day is defined as a constant accompanied by its corresponding abbreviation.
To store these abbreviations, a private field called abbreviation
is utilized within the enum
. A constructor is employed to initialize the abbreviation
for each constant.
Additionally, we introduce an extra method, getAbbreviation()
, which facilitates retrieving the abbreviation for a specific day.
Moving on to the main method, we illustrate how to access and leverage the enum
constants. By utilizing the values()
method, we obtain an array containing all enum
constants.
Through a loop, each day is iterated, and its name, along with the associated abbreviation, is printed. This provides a clear demonstration of how enums
can be effectively utilized in Java to represent and manipulate constant values.
Running the program produces the following output:
Days of the Week:
SUNDAY: Sun
MONDAY: Mon
TUESDAY: Tue
WEDNESDAY: Wed
THURSDAY: Thu
FRIDAY: Fri
SATURDAY: Sat
Using enums
to declare constants in Java offers a clean and organized way to represent a set of related values. Enums
provide not only the benefit of type safety but also the flexibility to include additional methods, making them a powerful tool for constant declarations.
In this example, we showcased how to use enums
to represent days of the week, emphasizing their clarity and ease of use in a real-world scenario. Consider employing enums
for your constant values to enhance code readability and maintainability in your Java projects.
Best Practices in Declaring Constants
Descriptive Naming
Choose meaningful and descriptive names for constants that convey their purpose. This enhances code readability and understanding.
Grouping in Interfaces
Group related constants within interfaces, creating a cohesive structure that reflects their semantic connections.
static final
Modifiers
Prefer the static final
modifiers when declaring constants within classes for immutability and shared accessibility.
Enumerations for Related Values
Use enums
for sets of related constants, providing type safety and a structured representation of closely associated values.
Avoiding Magic Numbers
Refrain from using magic numbers
directly in code; instead, declare them as named constants to enhance code clarity and maintenance.
Consistent Capitalization
Adopt consistent capitalization conventions (e.g., all uppercase) for constant names to improve uniformity and adherence to coding standards.
Conclusion
Constants play a pivotal role in Java programming, contributing to code clarity, readability, and maintainability. This article explored various methods for declaring constants, including the use of static final
modifiers, enums
, and interfaces.
Emphasizing best practices such as meaningful naming, grouping in interfaces, and consistent capitalization enhances the effectiveness of constants. Whether declared within classes, enums
, or interfaces, constants serve as stable, unchanging values that promote robust and understandable Java code, aligning with best practices for efficient and sustainable software development.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook