How to Concatenate Strings in Java
-
+
Operator Can Concatenate Values of Multiple Types, Whileconcat()
Can Only ConcatenateString
Values -
+
Generates a NewString
if the Value Is Empty, Whileconcat()
Returns the Same Value -
+
Will Concatenatenull
, Whileconcat()
Will Throw an Exception -
+
Can Concatenate Multiple Values, Whileconcat()
Can Take Only One Value
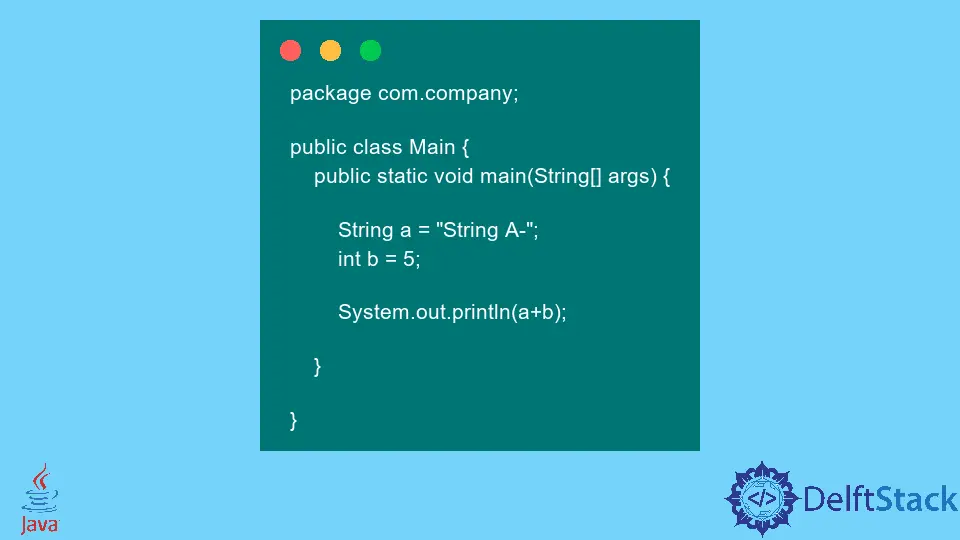
Today, we will be looking at the differences between concat()
and the +
operator. Both of them are used to concatenate Strings
, but we are here to find what makes them different from each other.
+
Operator Can Concatenate Values of Multiple Types, While concat()
Can Only Concatenate String
Values
The first big difference between +
and concat()
is that we can concatenate multiple data types with a String
using the +
operator, but the concat()
method is restricted to take only a String
type value.
If we look under the hood, every value of any data type is converted to a String
using the toString()
method when we join values using +
.
The below example shows that we can join an int b
to a String a
. But if we try this on concat()
, it will give a runtime error as it cannot take an int
type value.
package com.company;
public class Main {
public static void main(String[] args) {
String a = "String A-";
int b = 5;
System.out.println(a + b);
}
}
Output:
String A-5
+
Generates a New String
if the Value Is Empty, While concat()
Returns the Same Value
The next big difference to note down is that the +
will create a new String
every time it gets a value, even if its length is zero. But concat()
generates a new string only when it encounters that the value’s length is greater than zero.
This can change the result a lot if we want to compare two strings, as we have done below. The first comparison is done when the string is concatenated using concat()
, while the second comparison shows the result of comparing two strings concatenated by +
.
package com.company;
public class Main {
public static void main(String[] args) {
String a = "String A";
String b = "";
String d = a.concat(b);
if (d == a) {
System.out.println("String d and a are equal using concat");
} else {
System.out.println("String d and a are NOT equal using concat");
}
String e = a + b;
if (e == a) {
System.out.println("String e and a are equal using plus operator");
} else {
System.out.println("String e and a are NOT equal plus operator");
}
}
}
Output:
String d and a are equal using concat
String e and a are NOT equal plus operator
+
Will Concatenate null
, While concat()
Will Throw an Exception
In the below example, we can see that if we initialize the variable b
with null
it will still concatenate without any error. It happens because the +
operator converts every value to String
and then joins them.
package com.company;
public class Main {
public static void main(String[] args) {
String a = "String A-";
String b = null;
System.out.println(a + b);
}
}
Output:
String A-null
Unlike +
when we concatenate b
, which has null
in it to a
, it will throw a NullPointerException
, which is the right output in a general way.
package com.company;
public class Main {
public static void main(String[] args) {
String a = "String A-";
String b = null;
System.out.println(a.concat(b));
}
}
Output:
Exception in thread "main" java.lang.NullPointerException
at java.base/java.lang.String.concat(String.java:1937)
at com.company.Main.main(Main.java:14)
+
Can Concatenate Multiple Values, While concat()
Can Take Only One Value
The +
operator can join multiple values like we are doing in the following example. Still, as the concat()
function takes only one argument, it cannot concatenate more than two values.
package com.company;
public class Main {
public static void main(String[] args) {
String a = "String A-";
String b = "String B-";
String c = "String C";
System.out.println(a + b + c);
System.out.println(a.concat(b));
}
}
Output:
String A-String B-String C
String A-String B-
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn