How to Compare Strings Alphabetically in Java
-
Compare Strings Alphabetically Using the
compareTo()
Function - Compare Strings Alphabetically Using the Traditional Way
- Compare Strings Alphabetically Using Custom Comparator
- Conclusion
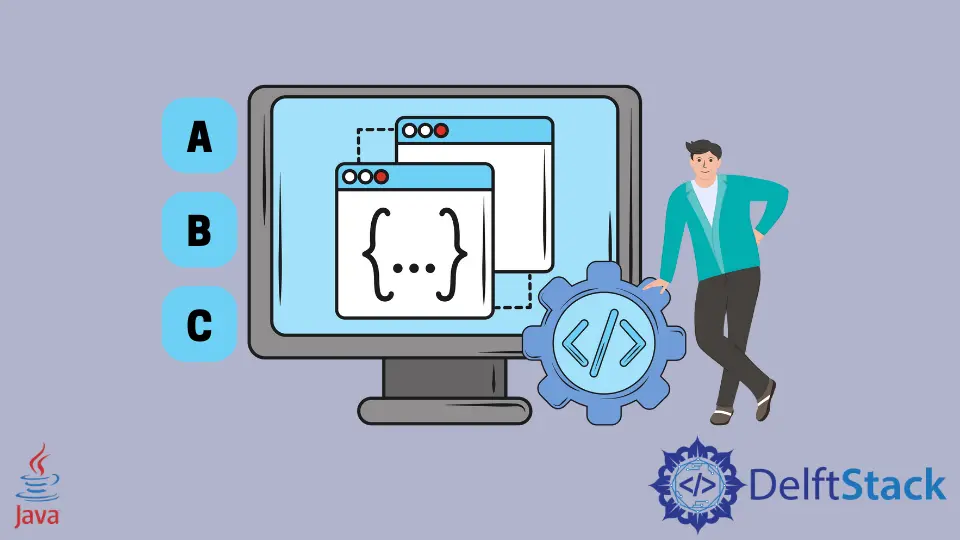
There are several ways to compare two or more strings in Java, but if you want to compare the strings lexicographically (alphabetically), here’s the article for you. In Java, the lexicographical order, or lexical order, refers to the alphabetic order or the sequence of ordered symbols in dictionaries or elements of an ordered set.
Below, we have examples that show three ways of comparing strings alphabetically in Java.
Compare Strings Alphabetically Using the compareTo()
Function
The compareTo()
method compares two strings lexicographically based on their Unicode character values. It returns an integer value that indicates whether one string is less than, equal to, or greater than the other string.
Basic Syntax:
int comparedResult = string1.compareTo(string2);
Parameters:
string1
: This is the string on which you call thecompareTo()
method. It is the first string in the comparison.string2
: This is the string that you want to compare withstring1
. It is the second string in the comparison.
The compareTo()
method then returns an integer value (comparedResult
) that indicates the result of the comparison. This value will be negative, positive, or zero, as described in the previous responses, depending on the lexicographical order of the two strings.
Let’s give an example:
class CompareStrings {
public static void main(String args[]) {
String s1 = "apple";
String s2 = "orange";
compareStrings(s1, s2);
String s3 = "apple";
String s4 = "Orange";
compareStrings(s3, s4);
String s5 = "sole";
String s6 = "soul";
compareStrings(s5, s6);
String s7 = "john";
String s8 = "johnson";
compareStrings(s7, s8);
String s9 = "one";
String s10 = "one";
compareStrings(s9, s10);
}
public static void compareStrings(String s1, String s2) {
int comparedResult = s1.compareTo(s2);
if (comparedResult > 0) {
System.out.println(s1 + " comes after " + s2);
} else if (comparedResult < 0) {
System.out.println(s1 + " comes before " + s2);
} else {
System.out.println(s1 + " is equal to " + s2);
}
}
}
In the code, we have a class named CompareStrings
. Inside the main
method, we declared several pairs of strings and compared them alphabetically using the compareTo()
method.
We see that s1
is compared to s2
, s3
to s4
, s5
to s6
, s7
to s8
, and s9
to s10
. These pairs of strings represent various scenarios, such as different words, case differences, and identical words.
The compareStrings
method takes two string parameters, s1
and s2
, and calculates the result of their alphabetical comparison. We used the compareTo()
method to obtain an integer value, comparedResult
.
Depending on this value, it prints out one of three possibilities.
If comparedResult
is positive, it means that the first string (in this case, s1
) comes after the second string (here, s2
), so the output states that "s1 comes after s2"
.
Next, if comparedResult
is negative, it means that the first string (s1
) comes before the second string (s2
), so the output states that "s1 comes before s2"
.
Lastly, if comparedResult
is zero, it indicates that the two strings are equal, and the output mentions that "s1 is equal to s2"
.
Output:
apple comes before orange
apple comes after Orange
sole comes before soul
john comes before johnson
one is equal to one
This output reflects the comparisons and provides clear information about the alphabetical relationships between the given pairs of strings. It demonstrates how the compareTo
method can be used to determine the relative order of strings in Java.
Compare Strings Alphabetically Using the Traditional Way
In this traditional way of comparing strings, we don’t use the built-in compareTo()
method but instead create our comparison logic. The following syntax is the custom comparison method that we’ll use in the following code example.
The below syntax is the central part of the code where we define the custom comparison method. Inside the method, we can implement our logic for comparing the two strings based on our specific requirements.
Basic Syntax:
public static int customCompare(String s1, String s2) {
// Custom comparison logic
// Return a positive value if s1 comes after s2
// Return a negative value if s1 comes before s2
// Return 0 if s1 and s2 are equal
}
Parameters:
s1
: This represents the first string you want to compare.s2
: This represents the second string you want to compare.
We can perform your custom comparison logic within the method, which should return an integer value based on the comparison results. The integer value’s sign indicates whether s1
comes before, after, or is equal to s2
in the alphabetical order.
Let’s give an example:
class CompareStrings {
public static void main(String[] args) {
String s1 = "apple";
String s2 = "orange";
int getValue1 = compareStrings(s1, s2);
String s3 = "apple";
String s4 = "Orange";
int getValue2 = compareStrings(s3, s4);
String s5 = "sole";
String s6 = "soul";
int getValue3 = compareStrings(s5, s6);
String s7 = "john";
String s8 = "johnson";
int getValue4 = compareStrings(s7, s8);
String s9 = "one";
String s10 = "one";
int getValue5 = compareStrings(s9, s10);
getComparisonResult(getValue1, s1, s2);
getComparisonResult(getValue2, s3, s4);
getComparisonResult(getValue3, s5, s6);
getComparisonResult(getValue4, s7, s8);
getComparisonResult(getValue5, s9, s10);
}
public static int compareStrings(String s1, String s2) {
for (int i = 0; i < s1.length() && i < s2.length(); i++) {
if ((int) s1.charAt(i) == (int) s2.charAt(i)) {
continue;
} else {
return (int) s1.charAt(i) - (int) s2.charAt(i);
}
}
if (s1.length() < s2.length()) {
return (s1.length() - s2.length());
} else if (s1.length() > s2.length()) {
return (s1.length() - s2.length());
} else {
return 0;
}
}
private static void getComparisonResult(int value, String s1, String s2) {
if (value > 0) {
System.out.println(s1 + " comes after " + s2);
} else if (value < 0) {
System.out.println(s1 + " comes before " + s2);
} else {
System.out.println(s1 + " and " + s2 + " are equal");
}
}
}
In the code, we declared several pairs of strings, such as s1
and s2
, s3
and s4
, and so on. These pairs represent different scenarios, including differences in case and identical words.
For each pair of strings, we call the compareStrings()
method to perform the alphabetical comparison. The method iterates through the characters of both strings, comparing their ASCII values, and if a character difference is found, the method returns the difference in ASCII values.
If no character difference is found while iterating, the method checks the length of the two strings. If one string is shorter, it returns a negative value based on the length difference.
If one string is longer, it returns a positive value based on the length difference. If the lengths are the same, it returns 0
, indicating equality.
We call the getComparisonResult()
method with the comparison result and the two strings being compared. This method prints the comparison result depending on the value
.
If the value
is positive, it means the first string comes after the second, so it prints "s1 comes after s2"
.
Then, if the value
is negative, it means the first string comes before the second, so it prints "s1 comes before s2"
.
Lastly, if the value
is zero, it indicates that the two strings are equal, and it prints "s1 and s2 are equal"
.
Output:
apple comes before orange
apple comes after Orange
sole comes before soul
john comes before johnson
one and one are equal
The output of the code displays the results of these comparisons, providing clear information about the alphabetical relationships between the given pairs of strings. It reflects the custom approach to string comparison, which does not rely on the compareTo()
method but rather calculates the order based on character values and lengths.
Compare Strings Alphabetically Using Custom Comparator
If we want to compare strings alphabetically using a custom comparator, we can create our comparator class and specify the custom comparison logic. We will use the following syntax in our next code example:
import java.util.Comparator;
public class CustomStringComparator implements Comparator<String> {
@Override
public int compare(String s1, String s2) {
// Custom comparison logic
// Return a positive value if s1 comes after s2
// Return a negative value if s1 comes before s2
// Return 0 if s1 and s2 are equal
}
}
Parameters:
s1
: This represents the first string to be compared.s2
: This represents the second string to be compared.
This basic syntax defines the structure of a custom comparator class for comparing strings. We can use this structure to create our custom string comparators with specific comparison logic.
Let’s give an example:
import java.util.Comparator;
public class AlphabeticalComparison {
public static void main(String[] args) {
String s1 = "apple";
String s2 = "banana";
String s3 = "cherry";
String s4 = "apple";
int comparedResult1 = compareStringsAlphabetically(s1, s2);
int comparedResult2 = compareStringsAlphabetically(s1, s3);
int comparedResult3 = compareStringsAlphabetically(s1, s4);
printComparisonResults(s1, s2, comparedResult1);
printComparisonResults(s1, s3, comparedResult2);
printComparisonResults(s1, s4, comparedResult3);
}
public static int compareStringsAlphabetically(String s1, String s2) {
return s1.compareTo(s2);
}
public static void printComparisonResults(String s1, String s2, int comparedResult) {
if (comparedResult < 0) {
System.out.println(s1 + " comes before " + s2);
} else if (comparedResult > 0) {
System.out.println(s1 + " comes after " + s2);
} else {
System.out.println(s1 + " is equal to " + s2);
}
}
}
In this code, we start by importing the java.util.Comparator
class to work with custom comparators.
We then have a main
method where we initialize several string variables (s1, s2, s3, and s4
) with different values. These strings represent words in alphabetical order, and we want to compare them.
The compareStringsAlphabetically()
method is used to perform the alphabetical comparison between two strings (s1 and s2
). This method utilizes the compareTo()
method, which is built into the String
class, to compare the two strings and return an integer value indicating their order.
To make the code more modular and reusable, we have a printComparisonResults()
method that takes the two strings being compared (s1 and s2
) and the comparison result as the parameters. This method prints out the comparison result based on the integer value returned by the compareStringsAlphabetically()
method.
Finally, in the main
method, we call compareStringsAlphabetically()
to compare s1
with s2
, s1
with s3
, and s1
with s4
. We also call the printComparisonResults()
method to display the results of these comparisons.
Output:
apple comes before banana
apple comes before cherry
apple is equal to apple
The output above displays the comparisons between the strings, showing whether one string comes before, after, or is equal to the other. It utilizes the custom comparator logic to determine the alphabetical order.
Conclusion
This article explores different methods for alphabetically comparing strings in Java. It covers the usage of the built-in compareTo()
method, which relies on Unicode character values for comparison.
Additionally, the article introduces a traditional approach to string comparison, where custom comparison logic is implemented, bypassing the use of compareTo()
. Furthermore, the article looks into the concept of custom comparators, explaining the structure of a custom string comparator class and the significance of the comparison method.
Whether you prefer the built-in compareTo()
method, a traditional custom comparison method, or a custom comparator, Java offers versatile options to meet your specific string comparison needs.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn