How to Compare Two Integers in Java
- Importance of Comparing Integer Values in Java
- Compare Two Integer Values in Java
- Best Practices in Comparing Integer Values in Java
- Conclusion
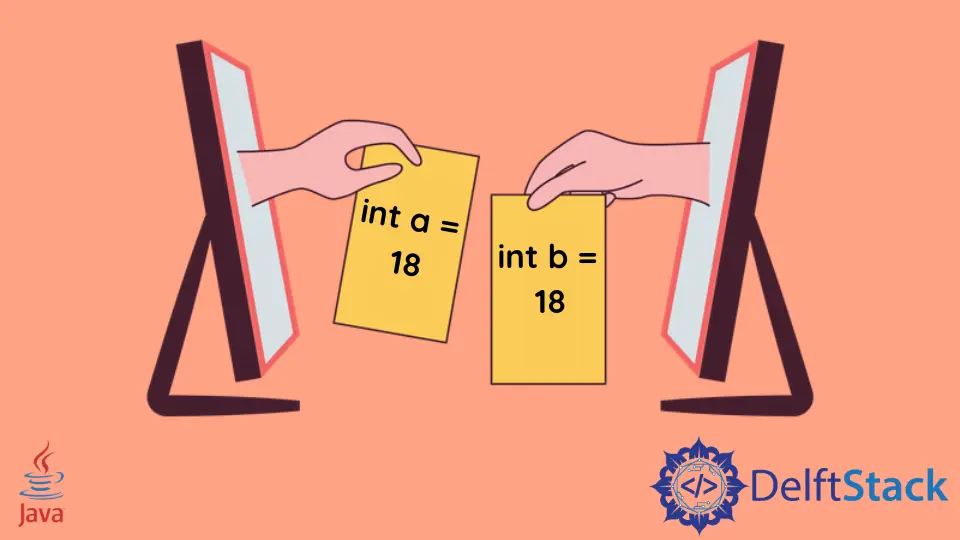
In this article, we will explore the significance of comparing integers in Java, delve into various methods such as relational operators, equals
, and compare
, and discuss best practices to ensure accurate and effective integer comparisons in programming.
While comparing the integer values, it is up to the developers to choose between the comparison methods. Let’s see some examples.
Importance of Comparing Integer Values in Java
Comparing integer values in Java is crucial for decision-making and control flow in programs. It allows developers to establish relationships between numbers, make logical decisions, and implement conditional behaviors.
Comparisons are fundamental for tasks such as sorting, searching, and filtering data. They form the basis for creating efficient and effective algorithms, enabling programs to respond dynamically to different numeric scenarios and optimize their execution based on specific conditions.
In essence, integer comparisons are essential for creating logic and structure in Java programs, enhancing their functionality and adaptability.
Compare Two Integer Values in Java
Using Relational Operators
In Java programming, the comparison of integers is a fundamental aspect, serving as the basis for decision-making and logical operations. One common approach is to use relational operators, such as <
, <=
, >
, >=
, ==
, and !=
, to establish relationships between numbers.
This method is particularly useful when dealing with Integer
objects, allowing for straightforward and concise comparisons.
Let’s delve into a practical example illustrating the comparison of two Integer
objects using relational operators:
public class IntegerComparisonExample {
public static void main(String[] args) {
// Declare and initialize two Integer objects
Integer num1 = 10;
Integer num2 = 5;
// Compare the two Integer objects using relational operators
if (num1 > num2) {
System.out.println("num1 is greater than num2");
} else if (num1 < num2) {
System.out.println("num1 is less than num2");
} else {
System.out.println("num1 is equal to num2");
}
}
}
In this example, we initiate the comparison of two Integer
objects, num1
and num2
, each assigned values 10 and 5, respectively. The use of Integer
objects, as opposed to primitive types, enables us to employ relational operators effectively.
The heart of this comparison lies within the if-else
construct. Initially, the code checks whether num1
is greater than num2
using the >
(greater than) operator. If this condition is true, the program outputs num1 is greater than num2
.
The subsequent else if
block addresses the scenario where num1
is less than num2
, as determined by the <
(less than) operator. If this condition holds, the output is num1 is less than num2
.
Lastly, the else
block handles the situation where neither of the preceding conditions is met, implying that num1
is equal to num2
. In this case, the program outputs num1 is equal to num2
.
Upon running this program, the output will be:
num1 is greater than num2
This output effectively demonstrates the power of using relational operators to compare two Integer
objects. It provides a clear and concise indication of the relationship between the two integers, showcasing the simplicity and effectiveness of this method.
Using the compareTo
Method
In Java programming, comparing integers is a fundamental operation for decision-making and ordering elements in various applications.
While relational operators like <
and >
are commonly used for basic comparisons, the compareTo
method provides a more nuanced approach. This method is particularly useful when dealing with objects of the Integer
class, allowing for a more comprehensive evaluation of the relationship between two integers.
Let’s explore a practical example demonstrating how to compare two integers using the compareTo
method:
public class IntegerComparisonExample {
public static void main(String[] args) {
// Declare and initialize two Integer objects
Integer num1 = 10;
Integer num2 = 5;
// Use the compareTo method to compare the two integers
int result = num1.compareTo(num2);
// Interpret the result and print the appropriate message
if (result > 0) {
System.out.println("num1 is greater than num2");
} else if (result < 0) {
System.out.println("num1 is less than num2");
} else {
System.out.println("num1 is equal to num2");
}
}
}
In this example, we initiate the comparison of two Integer
objects, num1
and num2
, each assigned values 10 and 5, respectively. Utilizing Integer
objects, rather than primitive types, facilitates the use of the compareTo
method.
The pivotal aspect of this illustration is the utilization of the compareTo
method.
This method is invoked on num1
, with num2
as its argument. The ensuing result, an integer value, serves as an indicator of the relative ordering of the two integers.
Subsequently, we capture this result in the variable result
and interpret it within an if-else
construct. If result
is greater than 0, it signifies that num1
is greater than num2
, leading to the output num1 is greater than num2
.
Conversely, if result
is less than 0, it indicates that num1
is less than num2
, resulting in the output 1num1 is less than num2
. Lastly, if result
is 0, the integers are deemed equal, prompting the output num1 is equal to num2
.
Upon running this program, the output will be:
num1 is greater than num2
This output demonstrates how the compareTo
method provides a detailed comparison result, offering information beyond simple equality. It allows for a more nuanced understanding of the relationship between the two integers, making it a powerful tool in scenarios where precise ordering information is required.
The compareTo
method enhances the capabilities of comparing integers in Java, especially when working with Integer
objects. Its versatility and detailed results contribute to more informed decision-making in various programming scenarios.
Using the equals
Method
The equals
method is vital for comparing integers as it ensures accurate value equality. While ==
compares object references, equals
examines content, making it essential for precise integer comparison.
Using equals
is crucial, especially with objects like Integer
, to prevent unintended behavior and guarantee accurate equality checks in Java.
Let’s explore a practical example demonstrating how to compare two integers using the equals
method:
public class IntegerComparisonExample {
public static void main(String[] args) {
// Declare and initialize two Integer objects
Integer num1 = 10;
Integer num2 = 5;
// Use the equals method to compare the two integers
if (num1.equals(num2)) {
System.out.println("num1 is equal to num2");
} else {
System.out.println("num1 is not equal to num2");
}
}
}
In this example, we begin by initializing two Integer
objects, num1
and num2
, with values 10 and 5, respectively. The use of Integer
objects instead of primitive types allows us to employ the equals
method effectively.
The focal point of this illustration is the utilization of the equals
method. We invoke this method on num1
, passing num2
as an argument. The method returns a boolean
value, indicating whether the two integers are equal.
To assess the result of the equals
method, we employ an if-else
construct. If the result is true
, it implies that num1
is equal to num2
, leading to the output num1 is equal to num2
.
Conversely, if the result is false
, we print num1 is not equal to num2
. This approach ensures accurate integer comparison and enhances the clarity of the Java program.
Upon running this program, the output will be:
num1 is not equal to num2
This output demonstrates the effectiveness of the equals
method in comparing two Integer
objects. It provides a clear indication of whether the integers are equal or not, offering a simple and concise approach to integer comparison.
The equals
method is a valuable tool for comparing Integer
objects in Java. It simplifies the process of equality checking, enhancing code readability and providing a convenient way to ascertain the equivalence of two integer values.
Using the compare
Method (Java 7 onwards)
The compare
method is crucial for precise integer comparisons, providing a nuanced result (-1
, 0
, or 1
) indicating the relationship between two integers. It ensures a comprehensive understanding of their ordering, facilitating informed decision-making in Java programs.
Let’s explore a practical example illustrating how to compare two integers using the compare
method:
public class IntegerComparisonExample {
public static void main(String[] args) {
// Declare and initialize two primitive integer variables
int num1 = 10;
int num2 = 5;
// Use the Integer.compare method to compare the two integers
int result = Integer.compare(num1, num2);
// Interpret the result and print the appropriate message
if (result > 0) {
System.out.println("num1 is greater than num2");
} else if (result < 0) {
System.out.println("num1 is less than num2");
} else {
System.out.println("num1 is equal to num2");
}
}
}
In this example, we initialize two primitive integer variables, num1
and num2
, with values 10 and 5, respectively, setting the stage for subsequent comparison. The core of this illustration lies in the use of the Integer.compare
method, a static method invoked on the Integer
class with num1
and num2
as arguments.
The resulting integer value from this method call precisely indicates the relative ordering of the two integers. We interpret this result within an if-else
construct, printing num1 is greater than num2
if the result is greater than 0, num1 is less than num2
if less than 0, and num1 is equal to num2
if the result is 0.
This method ensures a clear and nuanced understanding of the relationship between primitive integers, contributing to effective decision-making in Java programs.
Upon running this program, the output will be:
num1 is greater than num2
This output effectively demonstrates the power of the Integer.compare
method in providing a concise and nuanced result for comparing two integers. It allows developers to easily ascertain the relationship between numeric values and make informed decisions based on the comparison outcome.
The compare
method introduced in Java 7 offers a streamlined and expressive approach to integer comparisons. Its simplicity and efficiency make it a valuable addition to the Java language, providing developers with a powerful tool for comparing primitive integers.
Best Practices in Comparing Integer Values in Java
Use Primitives for Simple Comparisons
When dealing with simple integer values, prefer using primitive data types (int
, long
, etc.) instead of their wrapper classes (Integer
, Long
). This is more efficient and avoids unnecessary object creation.
Relational Operators for Simplicity
For basic integer comparisons, use the standard relational operators (<
, <=
, >
, >=
, ==
, !=
). They are concise and easy to understand.
Careful Handling of Nulls
If dealing with Integer objects and there’s a possibility of null values, ensure appropriate null checks before using methods like compareTo
or equals
to avoid NullPointerExceptions
.
Avoid Overhead with Primitives
When comparing primitive integers, prefer using the ==
operator over methods like equals
. The ==
operator is more performant and suitable for primitive types.
Consistent Use of compareTo
for Objects
When working with Integer
objects, use the compareTo
method for comparisons. It provides a clear indication of whether one integer is less than, equal to, or greater than another.
Use Integer.compare
for Primitives (Java 7+)
For primitive integer types (int
, long
, etc.) and when fine-grained comparison details are not needed, use Integer.compare
for simplicity and efficiency.
Consider Comparator
for Custom Comparisons
For more complex comparisons or custom sorting orders, consider implementing a custom Comparator<Integer>
.
Use switch
Statement for Equality Checks
When comparing against multiple fixed values for equality, consider using a switch
statement. It enhances code readability, especially when dealing with a limited set of possibilities.
Avoid Floating-Point Arithmetic for Equality
Avoid using floating-point arithmetic for integer equality checks due to precision issues. Use integer comparison or consider using the Math.abs
method for a tolerance-based approach.
Document Non-Obvious Comparisons
If your comparison logic is non-trivial or might not be immediately clear to other developers, add comments to explain the rationale behind the comparison.
Testing and Validation
Thoroughly test your comparison logic with various inputs, including edge cases and boundary values, to ensure correctness and reliability.
Conclusion
Comparing integers is a fundamental aspect of Java programming, crucial for decision-making and logical operations. Various methods, such as relational operators, equals
, and compare
, offer versatile approaches to address different needs.
The choice of method depends on the specific requirements of the comparison. Best practices involve considering the data types, using appropriate methods, and ensuring precision.
Whether leveraging the simplicity of relational operators or the nuanced results of equals
and compare
, understanding the importance of accurate integer comparison enhances the effectiveness and clarity of Java programs.