How to Clone Java Arrays
-
Manual Copy Java Array Using the
for
Loop in Java -
Using
Arrays.copyOf()
to Clone a Java Array -
Using
Arrays.copyOfRange()
to Clone a Java Array -
Using
Object.clone()
to Clone a Java Array -
Using
System.arraycopy()
to Clone a Java Array
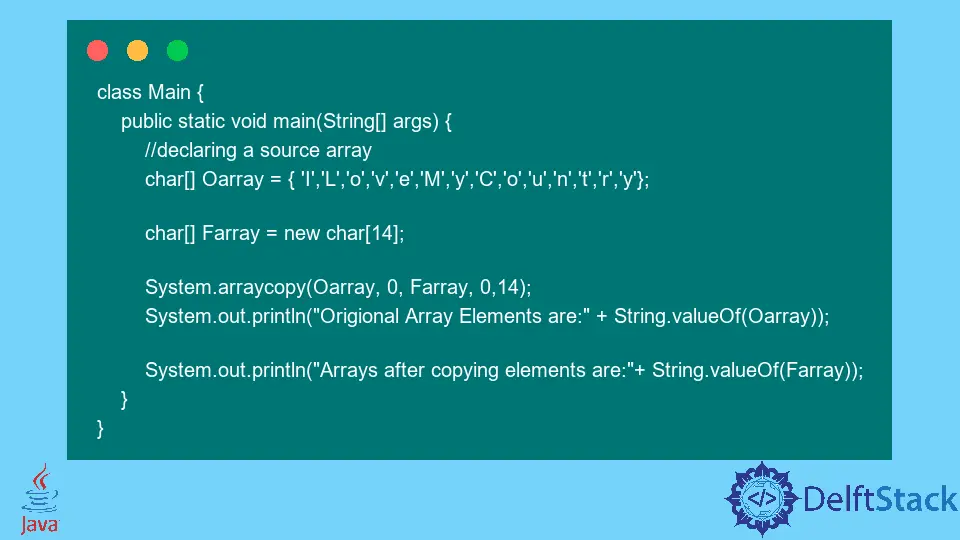
Java arrays can be copied into another array in the following ways.
- Using the variable assignment: This technique has its disadvantages because any change to an array element reflects across both places.
- Create an array that is the same size and copy every element.
- Make use of the clone technique to the array. Clone methods make a brand new array that is a similar size.
- Make use of the
System.arraycopy()
technique.arraycopy
is a method to copy a specific portion from an array.
Manual Copy Java Array Using the for
Loop in Java
Typically, the moment we want to copy variables like a
and b
, we carry out the copying operation in the following manner:
public class Main {
public static void main(String[] args) {
int IArray[] = {24, 45, 56};
System.out.println("Before assignment the values of IArray[] are:");
for (int i = 0; i < IArray.length; i++) System.out.print(IArray[i] + " ");
int CArray[] = new int[IArray.length];
CArray = IArray;
CArray[1]++;
System.out.println("\nElements of IArray[]:");
for (int i = 0; i < IArray.length; i++) System.out.print(IArray[i] + " ");
System.out.println("\nElements of CArray[]:");
for (int i = 0; i < CArray.length; i++) System.out.print(CArray[i] + " ");
}
}
Output:
Before assignment the values of IArray[] are:
24 45 56
Elements of IArray[]:
24 46 56
Elements of CArray[]:
24 46 56
It will not be effective when you apply this same procedure to arrays.
Using Arrays.copyOf()
to Clone a Java Array
This method that is Arrays.copyOf()
is helpful for internal use of the System.arraycopy()
procedure. While it’s not as effective as array copy, it could duplicate a full or part of arrays, similar to the array copy method. The copyOf()
The method is an element in java.util
package, and is part of the Arrays
class. The basic idea behind this method is:
public class Main {
public static void main(String args[]) {
int[] OArray = new int[] {11, 12, 13};
System.out.println("Original Arrays elements are");
for (int i = 0; i < OArray.length; i++) System.out.print(OArray[i] + " ");
int[] CArray = Arrays.copyOf(OArray, 5);
CArray[3] = 22;
CArray[4] = 66;
System.out.println("\nNew array after copying and modification are:");
for (int i = 0; i < copy.length; i++) System.out.print(copy[i] + " ");
}
}
Output:
Original Arrays elements are
11 12 13
New array after copying and modification are
11 12 13 22 66
Here,
original
: The array that will be copied into the newly created array.newLength
: Length of array copied that will be returned.
So, this method creates an exact copy of the array provided as the first argument to the specified size by padding or truncating the length by adding 0 to create the new array. This means that if the size of the array copied is greater than what was originally in the array 0,s are substituted for the remaining elements.
Using Arrays.copyOfRange()
to Clone a Java Array
Method Arrays.copyOfRange()
is specially designed to copy portions of arrays. Similar toCopyOf()
method the method utilizes the System.arraycopy()
technique. The basic idea behind the Arrays.copyOfRange()
procedure can be described as the following:
import java.util.Arrays;
class Main {
public static void main(String args[]) {
int IArray[] = {100, 200, 300, 400, 500};
int[] CArray = Arrays.copyOfRange(IArray, 2, 6);
System.out.println("Array within the index range : " + Arrays.toString(CArray));
int[] CArray1 = Arrays.copyOfRange(IArray, 4, IArray.length + 3);
System.out.println("Array copied within the indexed range: " + Arrays.toString(CArray1));
}
}
Output:
Array within the index range : [300, 400, 500, 0]
Array copied within the indexed range: [500, 0, 0, 0]
Here,
original
: the array of a range being copied.from
: the index for the array to copy inclusive.to
: the Index of the Range that is to be copied, and exclusive.
Using Object.clone()
to Clone a Java Array
Java arrays internally implement a Cloneable interface, so it is effortless to clone Java arrays. It is possible to clone one-dimensional and two-dimensional arrays. If you copy a one-dimensional array, it creates an extensive replica of the array elements that copies the values.
On the other hand, when you copy two-dimensional or multi-dimensional arrays, you get a small copy of the elements is created i.e., the only reference information is copied. This cloning process is accomplished using the clone()
method offered to the arrays.
class Main {
public static void main(String args[]) {
int NArray[] = {50, 100, 150, 200, 250, 300};
int CArray[] = NArray.clone();
System.out.println("Original Num Array are:");
for (int i = 0; i < NArray.length; i++) {
System.out.print(NArray[i] + " ");
}
System.out.println();
System.out.println("Cloned Num Array are:");
for (int i = 0; i < CArray.length; i++) {
System.out.print(CArray[i] + " ");
}
System.out.println("\n");
System.out.print("NArray == CArray = ");
System.out.println(NArray == CArray);
}
}