How to Catch Multiple Exceptions in Java
- Catch Multiple Exceptions Using Multiple Catch Statements in Java
-
Catch Multiple Exceptions Using
instanceOf
in Java -
Catch Multiple Exceptions in a Catch Block Using
|
in Java
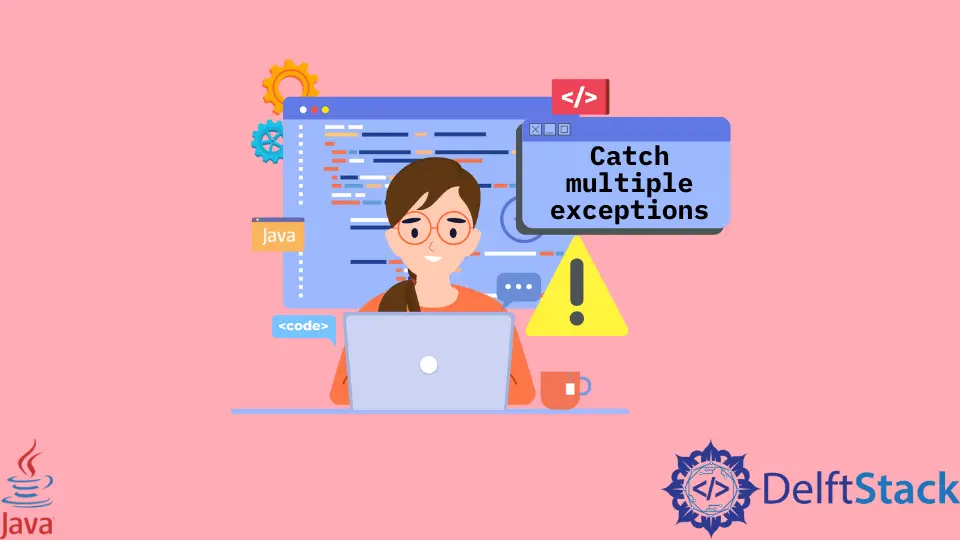
Today we’ll look at the ways we can use and catch multiple exceptions in Java. An exception in Java occurs when an unwanted event happens during runtime. The most common way to see an exception is to use the try-catch keywords. Here, the try
contains the block of code to be checked, and the catch
has the code to show when an exception occurs.
We will check out the three methods to use multiple exceptions in a single catch with the following examples.
Catch Multiple Exceptions Using Multiple Catch Statements in Java
In the example, we take the two int-type inputs from the user and then divide them to show the result. Notice that we have enclosed this code in a try
block; it means that it must have a catch
block too.
Multiple exceptions might be thrown like the InputMismatchException
when the inputted value is not of type int
and ArithmeticException
when num2
gets the value zero because no number is divided by zero. We can throw an Exception
that is the parent of all other exceptions.
To catch all of them, we write three different catch
blocks with their exception types and print out the message to show when that exception occurs. The output shows the different results when there’s no exception and when there are exceptions.
import java.util.InputMismatchException;
import java.util.Scanner;
public class MultiExceptions {
public static void main(String[] args) {
System.out.println("Enter two numbers: ");
Scanner sc = new Scanner(System.in);
try {
System.out.println("num1: ");
int num1 = sc.nextInt();
System.out.println("num2: ");
int num2 = sc.nextInt();
int dividedNum = num1 / num2;
System.out.println("After division result: " + dividedNum);
} catch (InputMismatchException e1) {
System.out.println("Error! - Please enter a number.");
} catch (ArithmeticException e2) {
System.out.println("Error! - You cannot divide any number with 0");
} catch (Exception e3) {
System.out.println("An error occurred please try again!");
}
}
}
Output 1:
Enter two numbers : num1 : 10 num2 : 2 After division result : 5
Output 2:
Enter two numbers : num1 : 50 num2 : hhh Error !-Please enter a number.
Output 3:
Enter two numbers : num1 : 10 num2 : 0 Error !-You cannot divide any number with 0
Catch Multiple Exceptions Using instanceOf
in Java
Another method you can do includes using the instanceOf
function, which checks whether an object is of the given type or not; it returns a boolean value. We use the same example as above, but here we use a single catch
block with multiple if-statements.
As discussed above, the Exception
is the parent class of the most common exceptions. We can use its object to check if the thrown exception is of the given type. Here, we review the two exceptions: InputMismatchException
and ArithmeticException
.
import java.util.InputMismatchException;
import java.util.Scanner;
public class MultiExceptions {
public static void main(String[] args) {
System.out.println("Enter two numbers: ");
Scanner sc = new Scanner(System.in);
try {
System.out.println("num1: ");
int num1 = sc.nextInt();
System.out.println("num2: ");
int num2 = sc.nextInt();
int dividedNum = num1 / num2;
System.out.println("After division result: " + dividedNum);
} catch (Exception e) {
if (e instanceof InputMismatchException) {
System.out.println("Error! - Please enter a number.");
} else if (e instanceof ArithmeticException) {
System.out.println("Error! - You cannot divide any number with 0");
} else {
System.out.println("An error occurred please try again!");
}
}
}
}
Output:
Enter two numbers:
num1:
d
Error! - Please enter a number.
Catch Multiple Exceptions in a Catch Block Using |
in Java
In Java 7 and later, we can use the pipe symbol or bitwise OR
to write multiple exceptions in a single catch. In the example, we divide the two inputted numbers and then check if any one of the two exceptions is true. If it’s true, the error message will be printed.
import java.util.InputMismatchException;
import java.util.Scanner;
public class MultiExceptions {
public static void main(String[] args) {
System.out.println("Enter two numbers: ");
Scanner sc = new Scanner(System.in);
try {
System.out.println("num1: ");
int num1 = sc.nextInt();
System.out.println("num2: ");
int num2 = sc.nextInt();
int dividedNum = num1 / num2;
System.out.println("After division result: " + dividedNum);
} catch (InputMismatchException | ArithmeticException e) {
System.out.println("An error occurred please try again");
}
}
}
Output:
Enter two numbers:
num1:
f
An error occurred please try again
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn