The Arrow Operator -> in Java
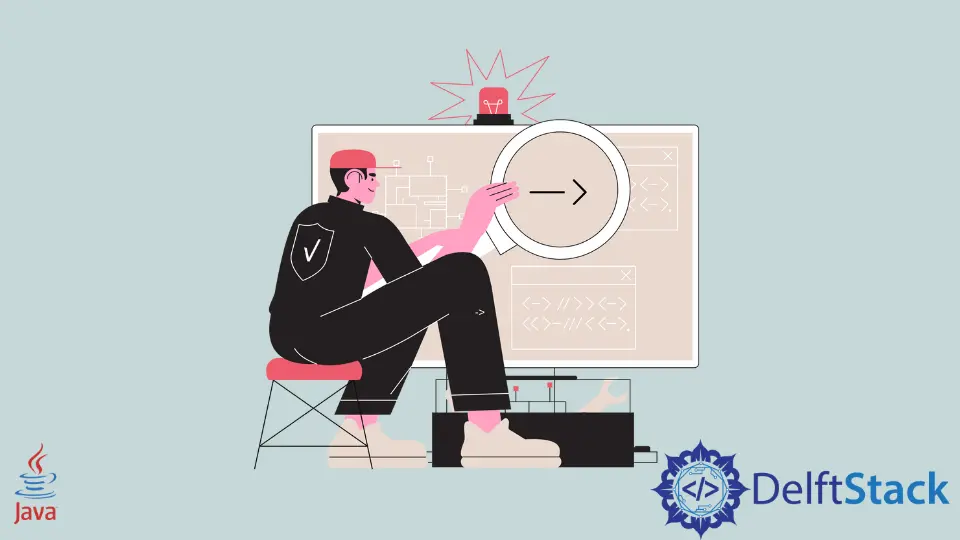
This article explores the arrow operator in Java, delving into its usage across various scenarios, including functional interfaces, collections, streams, sorting, concurrency, optional handling, and event-driven programming.
Arrow Operator in Java
In Java, the arrow operator (->
) is part of lambda expressions introduced in Java 8. It separates the input parameters from the body of a lambda expression, providing a concise syntax for defining anonymous functions.
The left side of the arrow specifies the lambda’s parameters, and the right side contains the code or expression to be executed. It is a shorthand notation for creating instances of functional interfaces in a more readable and compact way.
The syntax of a lambda function in Java is concise and follows a specific structure. The basic syntax is as follows:
(parameters) -> expression
or
(parameters) -> {
statements;
}
In a lambda expression in Java, the parameters
refer to the input variables, and you can have none, one, or more parameters.
If there’s only one parameter, you can skip the parentheses. If there are no parameters, you still need to use empty parentheses ()
.
The ->
arrow operator is used to separate the parameters from the body of the lambda expression. The expression
or { statements; }
on the right side of the arrow represents the body of the lambda.
If the body is a single expression, you can use a single expression without braces. However, if the body consists of multiple statements, you need to use curly braces {}
to define a block of code.
This concise syntax allows for the creation of anonymous functions in a readable and efficient manner, especially when working with functional interfaces.
Example | Code Snippet Example |
---|---|
Lambda expression with no parameters and a single expression | () -> "Hello, World!" |
Lambda expression with one parameter and a single expression | (x) -> x * x |
Lambda expression with multiple parameters and a block of statements | (a, b) -> { int sum = a + b; System.out.println("Sum: " + sum); return sum; } |
The table summarizes examples of lambda expressions in Java, demonstrating different scenarios with varying numbers of parameters and expressions. This concise syntax is particularly advantageous when working with functional interfaces, as seen in the java.util.function
package.
Various Scenarios to Use Arrow Operator in Java
Java 8 brought a significant evolution to the language with the introduction of lambda expressions. At the heart of this enhancement is the arrow operator (->
), a compact notation that simplifies the creation of anonymous functions.
Lambda expressions, empowered by the arrow operator, find extensive use in functional interfaces, collections, streams, comparator sorting, concurrency, optional handling, and event-driven programming.
Each file will contain the code relevant to a specific scenario. Below are the separate files for each scenario:
FunctionalInterfacesDemo
The arrow operator in functional interfaces is crucial as it enables concise expression of anonymous functions in Java. With the arrow operator, developers can create instances of functional interfaces without the need for verbose anonymous class syntax.
This simplifies code and enhances readability, promoting a more functional programming style where behavior can be passed around as data. Ultimately, the arrow operator empowers developers to write cleaner and more expressive code, facilitating the adoption of functional programming paradigms in Java.
public class FunctionalInterfacesDemo {
public static void main(String[] args) {
// Example 1: Functional Interfaces
Runnable myRunnable = () -> System.out.println("Executing...");
// Execute the run method
myRunnable.run();
}
}
In the FunctionalInterfacesDemo
file, we demonstrate the usage of lambda expressions with functional interfaces.
The example creates a Runnable
instance using a lambda expression, showcasing how the arrow operator simplifies the creation of anonymous functions.
CollectionsDemo
The arrow operator in collections simplifies the iteration and processing of elements. With the arrow operator, developers can define concise actions to be performed on each element of a collection, such as printing, filtering, or transforming them.
This eliminates the need for traditional loop structures, reducing boilerplate code and making the code more readable. The arrow operator promotes a functional programming approach, enabling developers to express operations on collections in a clear and concise manner, ultimately enhancing productivity and code maintainability.
import java.util.Arrays;
import java.util.List;
public class CollectionsDemo {
public static void main(String[] args) {
// Example 2: Collections and Iteration
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
}
}
In the CollectionsDemo
file, we showcase the use of lambda expressions for iteration over collections.
The example prints each element of a list of names by using the forEach
method, highlighting the concise syntax enabled by the arrow operator.
StreamsDemo
The arrow operator in streams is essential for streamlining data processing operations in Java. By using the arrow operator, developers can define concise transformations and filters on stream elements, allowing for a more fluent and expressive coding style.
This enables developers to perform complex data manipulations with minimal boilerplate code, leading to clearer and more maintainable codebases. The arrow operator encourages a functional programming paradigm, promoting the separation of concerns and facilitating the composition of operations, ultimately resulting in more efficient and readable stream-based processing pipelines.
import java.util.Arrays;
import java.util.List;
public class StreamsDemo {
public static void main(String[] args) {
// Example 3: Streams
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream().filter(n -> n % 2 == 0).forEach(System.out::println);
}
}
The StreamsDemo
file illustrates the power of the arrow operator in stream processing.
Through a pipeline of operations, the example filters even numbers from a list and prints them, demonstrating how lambda expressions streamline complex data processing tasks.
ComparatorSortingDemo
The arrow operator in comparators simplifies the creation of custom sorting logic in Java. By using the arrow operator, developers can define sorting criteria concisely within lambda expressions without the need for verbose anonymous classes.
This leads to cleaner and more readable code, as sorting logic is expressed in line with the comparator instantiation. The arrow operator promotes a more functional programming style, allowing for flexible and expressive sorting implementations, ultimately enhancing code clarity and maintainability.
import java.util.Arrays;
import java.util.List;
public class ComparatorSortingDemo {
public static void main(String[] args) {
// Example 4: Comparator for Sorting
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.sort((name1, name2) -> name1.length() - name2.length());
// Print sorted list
System.out.println("Sorted Names: " + names);
}
}
In ComparatorSortingDemo
, we demonstrate sorting using lambda expressions and the arrow operator.
The example sorts a list of names based on their lengths, showcasing how lambda expressions can define custom sorting logic concisely.
ConcurrencyDemo
The arrow operator in concurrency simplifies the creation of asynchronous tasks in Java. By using the arrow operator, developers can define tasks or actions to be executed in parallel without the need for verbose anonymous classes.
This streamlines the process of creating threads or submitting tasks to executor services, leading to cleaner and more readable code. The arrow operator promotes a functional programming approach to concurrency, allowing for clearer expression of parallel tasks and promoting better separation of concerns.
Overall, it enhances code readability and maintainability, making concurrent programming more accessible and efficient in Java.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ConcurrencyDemo {
public static void main(String[] args) {
// Example 5: Concurrency
ExecutorService executorService = Executors.newSingleThreadExecutor();
executorService.submit(() -> System.out.println("New thread running"));
executorService.shutdown();
}
}
The ConcurrencyDemo
file exhibits the use of lambda expressions in concurrent programming.
By submitting a task to an executor service, the example creates a new thread, demonstrating how the arrow operator simplifies asynchronous task execution.
OptionalDemo
The arrow operator enhances the handling of optional values in Java. By using the arrow operator, developers can succinctly define actions to be executed when a value is present within an optional object.
This eliminates the need for cumbersome null checks and promotes a more streamlined approach to handling potentially absent values. The arrow operator facilitates a clearer expression of optional value handling, leading to cleaner and more readable code.
Ultimately, it promotes better code organization and reduces the risk of null pointer exceptions, enhancing code reliability and maintainability.
import java.util.Optional;
public class OptionalDemo {
public static void main(String[] args) {
// Example 6: Optional
Optional<String> myOptional = Optional.of("Hello, World!");
myOptional.ifPresent(value -> System.out.println("Value: " + value));
}
}
In OptionalDemo
, we highlight the arrow operator’s role in handling optional values.
The example uses lambda expressions with Optional.ifPresent
to conditionally execute code when a value is present, showcasing how it enhances optional value handling.
EventHandlingDemo
The arrow operator in event handling simplifies the implementation of event-driven programming in Java. By using the arrow operator, developers can define event handlers with concise lambda expressions, eliminating the need for verbose anonymous classes.
This makes the code more readable and reduces boilerplate, as the event-handling logic is expressed inline. The arrow operator promotes a functional programming style in event handling, enabling clearer and more expressive code.
Ultimately, it streamlines the development of GUI applications and facilitates the creation of responsive and interactive user interfaces in Java.
import java.awt.event.ActionEvent;
import javax.swing.JButton;
import javax.swing.JFrame;
public class EventHandlingDemo {
public static void main(String[] args) {
// Example 7: Event Handling with Arrow Operator
JFrame frame = new JFrame("Event Handling Demo");
JButton myButton = new JButton("Click Me");
// Add ActionListener using lambda expression
myButton.addActionListener((ActionEvent e) -> { System.out.println("Button clicked!"); });
// Add button to the frame
frame.getContentPane().add(myButton);
// Set frame properties
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
frame.setVisible(true);
}
}
Lastly, EventHandlingDemo
demonstrates event handling in graphical user interfaces (GUIs) using lambda expressions.
By attaching an action listener to a button, the example responds to button clicks with a lambda expression, showcasing the arrow operator’s elegance in event-driven programming.
Each scenario showcases the arrow operator’s capability to streamline code, enhance readability, and promote a more functional style of programming in Java. By understanding and leveraging the arrow operator, developers can write more expressive and concise code across a wide range of Java applications.
Upon execution, the code produces a cohesive output reflecting the execution of each example. The console will display messages indicating the execution of runnable tasks, the iteration of names, the filtered even numbers, the sorted names, the running of a new thread, and the handling of optional and button events.
In conclusion, the arrow operator in Java brings a level of conciseness and expressiveness to code, enhancing readability and maintainability. Its applications span a variety of scenarios, from functional interfaces to event handling, demonstrating its versatility in modern Java programming.
Conclusion
The arrow operator in Java is a powerful and versatile feature that significantly enhances code expressiveness and conciseness. Throughout this article, we explored various scenarios where the arrow operator finds its utility, ranging from functional interfaces, collections, and streams to sorting, concurrency, optional handling, and event-driven programming.
Its concise syntax, coupled with lambda expressions, empowers developers to write cleaner and more readable code. Embracing the arrow operator opens doors to a more efficient and modern approach to Java programming, providing a valuable tool for enhancing both productivity and code clarity.