How to Get ArrayList of Int Array in Java
-
Create an
ArrayList
-
Add
int Arrays
to theArrayList
- Access an Element or an Entire Int Array
- Add Integer Element to an Array at a Specific Index
-
Remove an Element from the
ArrayList
-
Sort the
ArrayList
based on Array Sums -
ArrayList
of Int Array - Conclusion
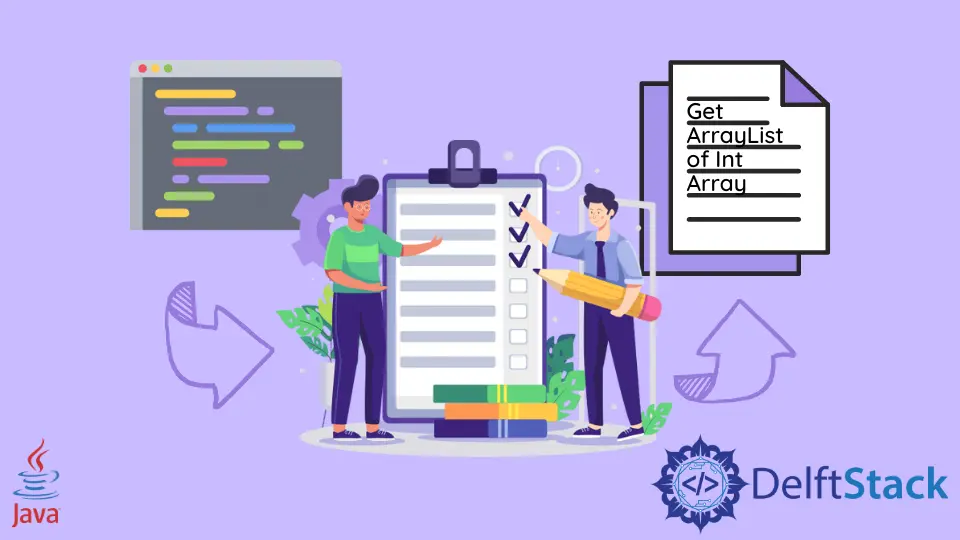
This tutorial introduces how to get ArrayList
of ints in Java and lists some example codes to understand the topic.
In Java programming, working with arrays is a common and essential task. However, there are scenarios where the flexibility of an ArrayList
is preferred over traditional arrays.
This is particularly true when dealing with dynamic data structures or when the size of the array is not known in advance.
In this article, we’ll explore the concept of obtaining an ArrayList
of int
arrays using the ArrayList
class in Java. We’ll cover the basic operations such as adding, accessing, removing, and sorting int
arrays within the ArrayList
.
Create an ArrayList
Creating an ArrayList
in Java is a straightforward process that involves instantiating the ArrayList
class from the java.util
package. To create an ArrayList
, you begin by importing the necessary package with the statement import java.util.ArrayList;
.
Once imported, you can declare an ArrayList
variable and initialize it using the new
keyword, followed by the ArrayList
class constructor.
Example:
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class ArrayListOfIntArray {
public static void main(String[] args) {
// Create an ArrayList of int arrays
ArrayList<int[]> listOfArrays = new ArrayList<>();
// ...
}
}
In this snippet, we start by importing the necessary Java libraries and declare a class named ArrayListOfIntArray
. Inside the main
method, we initialize an ArrayList
named listOfArrays
to hold int arrays.
The diamond operator (<>
) on the right side is a shorthand introduced in Java 7 for type inference, making the code more concise. At this point, the ArrayList
is ready for use, and you can add, remove, or manipulate elements within it.
Add int Arrays
to the ArrayList
You can use the add
method to insert int arrays into the ArrayList
.
Example:
// Add int arrays to the ArrayList
listOfArrays.add(new int[] {5, 2, 8, 1, 7});
listOfArrays.add(new int[] {3, 9, 4, 6, 0});
These lines add two int arrays to the ArrayList
listOfArrays
, demonstrating the ability to store multiple arrays within the ArrayList
.
This process can be repeated to add multiple int arrays, providing a flexible and dynamic structure for storing arrays of integers.
Access an Element or an Entire Int Array
Accessing an element or an int array in an ArrayList
in Java is a straightforward process. Once an int array is added to the ArrayList
, you can use the get
method to retrieve the array or access individual elements.
In Java, to access an array at a specific index and a specific element within an array, you can use the following syntax:
Accessing an Array at a Specific Index:
int[] myArray = arrayListOfIntArrays.get(index);
Here, index
is the position of the int array within the ArrayList
that you want to access. The get
method retrieves the int array at the specified index.
Accessing a Specific Element within an Array:
int myElement = myArray[elementIndex];
In this syntax, elementIndex
represents the index of the specific element you want to access within the int array. The value is then stored in the variable myElement
.
Remember to replace index
, arrayListOfIntArrays
, elementIndex
, and myArray
with the appropriate variable names or values in your specific code.
Example:
// Access an element of the first array
int element = listOfArrays.get(0)[3];
// Access the entire int array
int[] firstArray = listOfArrays.get(0);
These lines demonstrate accessing individual elements and the entire int array at index 0 in the ArrayList
. The accessed element and array are then stored in variables for later display.
Add Integer Element to an Array at a Specific Index
To add an integer element to an array at a specific index within an ArrayList
in Java, you can follow a two-step process.
First, retrieve the int array from the ArrayList
using the get
method and assign it to a variable. Next, you can modify the desired element within the array using standard array notation.
Example:
// Add an element to a specific index in the first array
listOfArrays.get(0)[2] = 42;
This line modifies the first array in the ArrayList
by adding the integer 42
to the specific index 2
.
Remove an Element from the ArrayList
To remove an element at a specific index from an array within an ArrayList
in Java, you can use a custom method, as illustrated in the provided code snippet. The removeElement
method is designed to take an array and the index of the element to be removed, and it returns a new array with the specified element removed.
In this case, the code removes an element at index 1 from the int array at index 0 in the listOfArrays
ArrayList
.
// Remove an element from the first array
int indexToRemove = 1;
listOfArrays.get(0) = removeElement(listOfArrays.get(0), indexToRemove);
The removeElement
method utilizes System.arraycopy
to create a new array with the specified element removed. It copies the elements before the index and after the index into a new array, effectively excluding the element at the specified index.
The result is then assigned back to the original array at index 0 in the listOfArrays
.
Sort the ArrayList
based on Array Sums
Sorting an ArrayList
based on the sum of arrays within it in Java involves using the Collections.sort
method with a custom comparator. The comparator compares the sums of two arrays, ensuring that the ArrayList
is sorted in ascending order according to the array sums.
// Sort the ArrayList based on the sum of each int array
Collections.sort(listOfArrays, (a1, a2) -> {
int sum1 = Arrays.stream(a1).sum();
int sum2 = Arrays.stream(a2).sum();
return Integer.compare(sum1, sum2);
});
// Display the sorted ArrayList
System.out.println("Sorted ArrayList:");
for (int[] array : listOfArrays) {
System.out.println(Arrays.toString(array));
}
By specifying this custom comparator, the Collections.sort
method rearranges the ArrayList
so that arrays with smaller sums come first, and arrays with larger sums come later.
This output reflects the various modifications and operations performed on the ArrayList
of int arrays, showcasing the flexibility and functionality of the code.
ArrayList
of Int Array
Arrays and ArrayLists
are fundamental data structures in Java, providing the means to store and manipulate collections of elements. However, there are scenarios where combining these structures becomes essential.
We will discuss fundamental tasks like adding, accessing, removing, and sorting arrays of integers within the ArrayList
, consolidating the various code snippets provided earlier.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class ArrayListOfIntArray {
public static void main(String[] args) {
// Create an ArrayList of int arrays
ArrayList<int[]> listOfArrays = new ArrayList<>();
// Add int arrays to the ArrayList
listOfArrays.add(new int[] {5, 2, 8, 1, 7});
listOfArrays.add(new int[] {3, 9, 4, 6, 0});
// Add an element to a specific index in the first array
listOfArrays.get(0)[2] = 42;
// Access an element of the first array
int element = listOfArrays.get(0)[3];
// Access the entire int array
int[] firstArray = listOfArrays.get(0);
// Remove an element from the first array
int indexToRemove = 1;
listOfArrays.get(0) = removeElement(listOfArrays.get(0), indexToRemove);
// Get the size of the first array
int size = listOfArrays.get(0).length;
// Sort the ArrayList based on the sum of each int array
Collections.sort(listOfArrays, (a1, a2) -> {
int sum1 = Arrays.stream(a1).sum();
int sum2 = Arrays.stream(a2).sum();
return Integer.compare(sum1, sum2);
});
// Display all outputs
System.out.println("Modified First Array: " + Arrays.toString(listOfArrays.get(0)));
System.out.println("Accessed Element: " + element);
System.out.println("Accessed Array: " + Arrays.toString(firstArray));
System.out.println("Size of First Array: " + size);
System.out.println("Sorted ArrayList:");
for (int[] array : listOfArrays) {
System.out.println(Arrays.toString(array));
}
}
// Method to remove an element from an int array
private static int[] removeElement(int[] array, int index) {
int[] result = new int[array.length - 1];
System.arraycopy(array, 0, result, 0, index);
System.arraycopy(array, index + 1, result, index, array.length - index - 1);
return result;
}
}
We initiate the process by creating an ArrayList
, a versatile container for int arrays, allowing for dynamic and flexible data storage. Subsequently, we enrich the ArrayList
by adding two distinct int arrays, showcasing its capability to accommodate multiple arrays efficiently.
To demonstrate array manipulation, we tailor the first array, introducing an element at a specific index, and then proceed to access individual elements as well as the entire array. The removal of an element from an int array is exemplified through a custom removeElement
method.
Size retrieval of the int array within the ArrayList
is then elucidated, providing insights into dynamic size management.
Finally, we delve into the sorting capability of the ArrayList
, emphasizing its adaptability by sorting the arrays based on the sum of their elements.
Output:
Modified First Array: [5, 2, 42, 1, 7]
Accessed Element: 1
Accessed Array: [5, 2, 42, 1, 7]
Size of First Array: 4
Sorted ArrayList:
[1, 7, 42, 5, 2]
[0, 3, 4, 6, 9]
This array manipulation journey showcases the potency and flexibility of employing an ArrayList
to manage int arrays dynamically.
Conclusion
Combining the flexibility of ArrayLists
with the structure of int arrays provides a powerful toolset for dynamic data manipulation in Java. This approach allows developers to efficiently manage and manipulate arrays in a resizable and convenient manner.
The given example demonstrated how to add, access, modify, and remove int arrays, as well as how to get the size of the ArrayList
and perform sorting based on the contents of the arrays. This technique not only simplifies array manipulation but also enhances the flexibility and functionality of your Java programs.