Obtener ArrayList del array int en Java
-
Creación de
ArrayList
de tipo int -
Agregar elemento entero a un índice en
ArrayList
-
Accediendo al elemento
ArrayList
con el índice - ArrayList de matrices int
-
Accediendo al elemento de array int desde
ArrayList
- ArrayList de ArrayLists
- Resumen
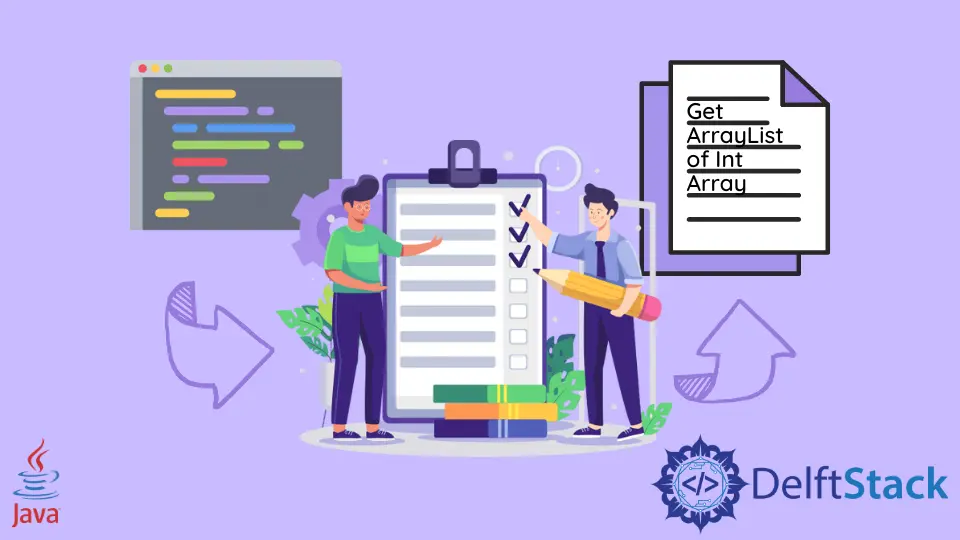
Este tutorial presenta cómo obtener ArrayList
de ints en Java y enumera algunos códigos de ejemplo para comprender el tema.
Una ArrayList
es un array dinámica o redimensionable. Es parte de Collection Framework en Java. ArrayList
se utiliza para superar el problema del tamaño fijo de los arrays normales. En este tutorial, aprenderemos cómo crear ArrayList
de ints.
Creación de ArrayList
de tipo int
ArrayList
o cualquier otra colección no puede almacenar tipos de datos primitivos como int. Si escribimos el código que se muestra a continuación, obtendremos un error de compilación.
public class Demo {
public static void main(String[] args) {
ArrayList<int> arrList;
}
}
Producción :
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Syntax error, insert "Dimensions" to complete ReferenceType
at snippet.Demo.main(Demo.java:7)
En su lugar, usamos clases contenedoras para almacenar primitivas en ArrayList
. Cada tipo de datos primitivo tiene una clase contenedora correspondiente que representa un objeto para el mismo tipo. Para el tipo de datos int, la clase contenedora se llama Integer
. Entonces, para crear una ArrayList
de ints, necesitamos usar la clase contenedora Integer
como su tipo.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
}
}
Ahora podemos agregar enteros a la ArrayList
usando el método add()
de la clase.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
}
}
ArrayList
, al igual que los arrays normales, sigue la indexación basada en cero. Podemos especificar el índice donde queremos agregar un objeto en el método add()
. El elemento presente en ese índice y todos los elementos a su derecha se desplazarán un lugar a la derecha.
Agregar elemento entero a un índice en ArrayList
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
arrList.add(1, 199); // Inserting 199 at index 1.
}
}
Accediendo al elemento ArrayList
con el índice
Podemos buscar elementos individuales de ArrayList
utilizando sus índices. Pase el valor del índice al método get()
para obtener el elemento requerido.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
arrList.add(1, 199); // Inserting 199 at index 1.
System.out.println("Element at index 1: " + arrList.get(1));
System.out.println("Element at index 2: " + arrList.get(2));
}
}
Producción :
Element at index 1: 199
Element at index 2: 7
También podemos imprimir toda la ArrayList
utilizando una única declaración de impresión.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
System.out.println("Printing the ArrayList: " + arrList);
arrList.add(1, 199); // Inserting 199 at index 1.
System.out.println("Printing the ArrayList: " + arrList);
}
}
Producción :
Printing the ArrayList: [5, 7, 12]
Printing the ArrayList: [5, 199, 7, 12]
ArrayList de matrices int
Podemos crear una ArrayList
donde cada elemento en sí mismo es un array. Usamos el tipo de datos y los corchetes para crear una nueva matriz.
Del mismo modo, definimos el tipo de ArrayList
utilizando int[]
. No podemos usar primitivas como int como tipo ArrayList
, pero podemos usar int[]
. Esto se debe a que los arrays en Java son objetos, no primitivas. Y una ArrayList
puede ser creada por cualquier tipo de objeto (matrices en nuestro caso).
ArrayList<int[]> arrList = new ArrayList<int[]>();
Podemos realizar todas las operaciones básicas que comentamos anteriormente. Necesitamos usar el método toString()
de Arrays
para imprimir correctamente el array en la consola.
import java.util.ArrayList;
import java.util.Arrays;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
// Fetching the array from the List
int[] arrAtIdx1 = arrList.get(1);
// Printing the fetched array using the toString() method of Arrays
System.out.println("The Second array in the List is: " + Arrays.toString(arrAtIdx1));
}
}
Producción :
The Second array in the List is: [3, 6, 9]
Accediendo al elemento de array int desde ArrayList
También podemos acceder a los elementos individuales de los arrays int presentes en la ArrayList
. Usaremos índices de array para hacer esto. Por ejemplo, si deseamos acceder al segundo elemento del tercer array, usaremos el siguiente código:
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
// Fetching the second element of the third array
int[] thirdArr = arrList.get(2);
int secondElement = thirdArr[1];
System.out.println("Second Element of the Third Array is: " + secondElement);
}
}
Producción :
Second Element of the Third Array is: 10
Sin embargo, necesitamos código adicional para imprimir toda la ArrayList
de matrices.
import java.util.ArrayList;
import java.util.Arrays;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
for (int i = 0; i < arrList.size(); i++) {
int[] currArr = arrList.get(i);
System.out.println("Array at index " + i + " is: " + Arrays.toString(currArr));
}
}
}
Producción :
Array at index 0 is: [2, 4, 6]
Array at index 1 is: [3, 6, 9]
Array at index 2 is: [5, 10, 15]
ArrayList de ArrayLists
Como se discutió anteriormente, los arrays son de longitud fija, pero las ArrayLists
son dinámicas. En lugar de crear una ArrayList
de matrices int, podemos crear una ArrayList
de Integer
ArrayLists
. De esta manera, no tendremos que preocuparnos por quedarnos sin espacio en nuestra matriz.
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
Podemos usar el método add()
y el método get()
como antes. Sin embargo, necesitamos un bucle para imprimir cada elemento ArrayList
.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
// Creating individual ArrayLists
ArrayList<Integer> arrList1 = new ArrayList<>();
arrList1.add(2);
arrList1.add(4);
arrList1.add(6);
ArrayList<Integer> arrList2 = new ArrayList<>();
arrList2.add(3);
arrList2.add(6);
arrList2.add(9);
ArrayList<Integer> arrList3 = new ArrayList<>();
arrList3.add(5);
arrList3.add(10);
arrList3.add(15);
// Adding ArrayLists to the ArrayList
arrListOfarrLists.add(arrList1);
arrListOfarrLists.add(arrList2);
arrListOfarrLists.add(arrList3);
// Fetching ArrayList
ArrayList<Integer> listAtIdx1 = arrListOfarrLists.get(1);
System.out.println("ArrayList present at index 1 is: " + listAtIdx1 + "\n");
// Printing the entire ArrayList
for (int i = 0; i < arrListOfarrLists.size(); i++)
System.out.println("ArrayList at index " + i + " is " + arrListOfarrLists.get(i));
}
}
Producción :
ArrayList present at index 1 is: [3, 6, 9]
ArrayList at index 0 is [2, 4, 6]
ArrayList at index 1 is [3, 6, 9]
ArrayList at index 2 is [5, 10, 15]
Si desea acceder a elementos individuales de ArrayList
, utilice el método get()
dos veces. Por ejemplo, si desea el segundo elemento del tercer ArrayList
, utilice:
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
// Creating individual ArrayLists
ArrayList<Integer> arrList1 = new ArrayList<>();
arrList1.add(2);
arrList1.add(4);
arrList1.add(6);
ArrayList<Integer> arrList2 = new ArrayList<>();
arrList2.add(3);
arrList2.add(6);
arrList2.add(9);
ArrayList<Integer> arrList3 = new ArrayList<>();
arrList3.add(5);
arrList3.add(10);
arrList3.add(15);
// Adding ArrayLists to the ArrayList
arrListOfarrLists.add(arrList1);
arrListOfarrLists.add(arrList2);
arrListOfarrLists.add(arrList3);
// Fetching second element of the third ArrayList
ArrayList<Integer> thirdList = arrListOfarrLists.get(2);
int secondElement = thirdList.get(1);
System.out.print("second element of the third ArrayList is: " + secondElement);
}
}
Producción :
second element of the third ArrayList is: 10
Resumen
ArrayList
es probablemente la colección más utilizada en Java. Es una estructura de datos simple que se utiliza para almacenar elementos del mismo tipo. No podemos crear una ArrayList
de tipos primitivos como int. Necesitamos usar las clases contenedoras de estas primitivas. La clase ArrayList
proporciona métodos convenientes para agregar y recuperar elementos de la lista. También podemos crear una ArrayList
de matrices o una ArrayList
de ArrayLists
. Se utilizan principalmente para representar datos en un formato de array 2D o un formato tabular. Es mejor usar una ArrayList
de ArrayLists
, ya que no limitará su tamaño.