Obtenha ArrayList do array int em Java
-
Criando
ArrayList
do tipo int -
Adicionando elemento inteiro a um índice em
ArrayList
-
Acessando o elemento
ArrayList
com o índice - ArrayList of int arrays
-
Acessando o elemento do array int de
ArrayList
- ArrayList de ArrayLists
- Resumo
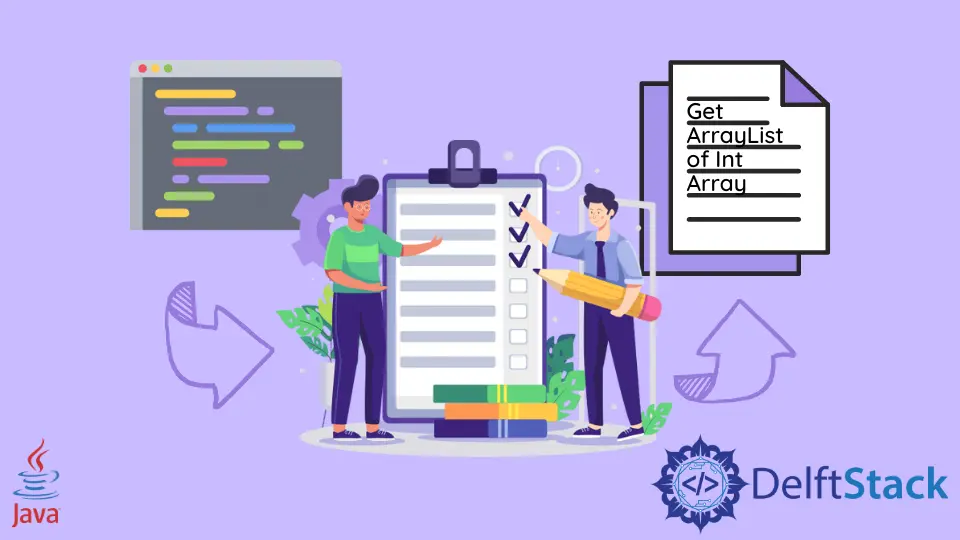
Este tutorial apresenta como obter ArrayList
de ints em Java e lista alguns códigos de exemplo para entender o tópico.
Uma ArrayList
é um array dinâmica ou redimensionável. Faz parte do Collection Framework em Java. ArrayList
é usado para superar o problema do tamanho fixo dos arrays normais. Neste tutorial, aprenderemos como criar ArrayList
de ints.
Criando ArrayList
do tipo int
ArrayList
ou qualquer outra coleção não pode armazenar tipos de dados primitivos, como int. Se escrevermos o código mostrado abaixo, obteremos um erro de compilação.
public class Demo {
public static void main(String[] args) {
ArrayList<int> arrList;
}
}
Produção:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Syntax error, insert "Dimensions" to complete ReferenceType
at snippet.Demo.main(Demo.java:7)
Em vez disso, usamos classes de wrapper para armazenar primitivos em ArrayList
. Cada tipo de dado primitivo possui uma classe de invólucro correspondente que representa um objeto do mesmo tipo. Para o tipo de dados int, a classe wrapper é chamada Integer
. Portanto, para criar uma ArrayList
de ints, precisamos usar a classe wrapper Integer
como seu tipo.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
}
}
Agora podemos adicionar inteiros à ArrayList
usando o método add()
da classe.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
}
}
ArrayList
, assim como arrays normais, segue a indexação baseada em zero. Podemos especificar o índice onde queremos adicionar um objeto no método add()
. O elemento presente nesse índice e todos os elementos à sua direita deslocarão um lugar para a direita.
Adicionando elemento inteiro a um índice em ArrayList
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
arrList.add(1, 199); // Inserting 199 at index 1.
}
}
Acessando o elemento ArrayList
com o índice
Podemos buscar itens individuais ArrayList
usando seus índices. Passe o valor do índice para o método get()
para buscar o elemento necessário.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
arrList.add(1, 199); // Inserting 199 at index 1.
System.out.println("Element at index 1: " + arrList.get(1));
System.out.println("Element at index 2: " + arrList.get(2));
}
}
Produção:
Element at index 1: 199
Element at index 2: 7
Também podemos imprimir a ArrayList
inteira usando uma única instrução de impressão.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<Integer> arrList = new ArrayList<Integer>();
arrList.add(5);
arrList.add(7);
arrList.add(12);
System.out.println("Printing the ArrayList: " + arrList);
arrList.add(1, 199); // Inserting 199 at index 1.
System.out.println("Printing the ArrayList: " + arrList);
}
}
Produção:
Printing the ArrayList: [5, 7, 12]
Printing the ArrayList: [5, 199, 7, 12]
ArrayList of int arrays
Podemos criar um ArrayList
onde cada elemento é um array. Usamos o tipo de dados e colchetes para criar uma nova matriz.
Da mesma forma, definimos o tipo de ArrayList
usando int[]
. Não podemos usar primitivas como int como tipo ArrayList
, mas podemos usar int[]
. Isso ocorre porque os arrays em Java são objetos, não primitivos. E uma ArrayList
pode ser criada por qualquer tipo de objeto (arrays em nosso caso).
ArrayList<int[]> arrList = new ArrayList<int[]>();
Podemos realizar todas as operações básicas que discutimos acima. Precisamos usar o método toString()
de Arrays
para imprimir corretamente o array no console.
import java.util.ArrayList;
import java.util.Arrays;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
// Fetching the array from the List
int[] arrAtIdx1 = arrList.get(1);
// Printing the fetched array using the toString() method of Arrays
System.out.println("The Second array in the List is: " + Arrays.toString(arrAtIdx1));
}
}
Produção:
The Second array in the List is: [3, 6, 9]
Acessando o elemento do array int de ArrayList
Também podemos acessar os elementos individuais das matrizes int presentes na ArrayList
. Usaremos índices de array para fazer isso. Por exemplo, se quisermos acessar o segundo elemento da terceira matriz, usaremos o seguinte código:
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
// Fetching the second element of the third array
int[] thirdArr = arrList.get(2);
int secondElement = thirdArr[1];
System.out.println("Second Element of the Third Array is: " + secondElement);
}
}
Produção:
Second Element of the Third Array is: 10
No entanto, precisamos de código adicional para imprimir toda a ArrayList
de arrayes.
import java.util.ArrayList;
import java.util.Arrays;
public class Demo {
public static void main(String[] args) {
ArrayList<int[]> arrList = new ArrayList<int[]>();
int[] arr1 = {2, 4, 6};
int[] arr2 = {3, 6, 9};
int[] arr3 = {5, 10, 15};
// Adding int arrays to the ArrayList
arrList.add(arr1);
arrList.add(arr2);
arrList.add(arr3);
for (int i = 0; i < arrList.size(); i++) {
int[] currArr = arrList.get(i);
System.out.println("Array at index " + i + " is: " + Arrays.toString(currArr));
}
}
}
Produção:
Array at index 0 is: [2, 4, 6]
Array at index 1 is: [3, 6, 9]
Array at index 2 is: [5, 10, 15]
ArrayList de ArrayLists
Como discutido acima, os arrays têm comprimento fixo, mas ArrayLists
são dinâmicos. Em vez de criar uma ArrayList
de arrays int, podemos criar uma ArrayList
de Integer
ArrayLists
. Dessa forma, não precisamos nos preocupar em ficar sem espaço em nosso array.
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
Podemos usar o método add()
e o método get()
como antes. No entanto, exigimos um loop para imprimir cada elemento ArrayList
.
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
// Creating individual ArrayLists
ArrayList<Integer> arrList1 = new ArrayList<>();
arrList1.add(2);
arrList1.add(4);
arrList1.add(6);
ArrayList<Integer> arrList2 = new ArrayList<>();
arrList2.add(3);
arrList2.add(6);
arrList2.add(9);
ArrayList<Integer> arrList3 = new ArrayList<>();
arrList3.add(5);
arrList3.add(10);
arrList3.add(15);
// Adding ArrayLists to the ArrayList
arrListOfarrLists.add(arrList1);
arrListOfarrLists.add(arrList2);
arrListOfarrLists.add(arrList3);
// Fetching ArrayList
ArrayList<Integer> listAtIdx1 = arrListOfarrLists.get(1);
System.out.println("ArrayList present at index 1 is: " + listAtIdx1 + "\n");
// Printing the entire ArrayList
for (int i = 0; i < arrListOfarrLists.size(); i++)
System.out.println("ArrayList at index " + i + " is " + arrListOfarrLists.get(i));
}
}
Produção:
ArrayList present at index 1 is: [3, 6, 9]
ArrayList at index 0 is [2, 4, 6]
ArrayList at index 1 is [3, 6, 9]
ArrayList at index 2 is [5, 10, 15]
Se você deseja acessar elementos individuais de ArrayList
, use o método get()
duas vezes. Por exemplo, se você quiser o segundo elemento do terceiro ArrayList
, use:
import java.util.ArrayList;
public class Demo {
public static void main(String[] args) {
ArrayList<ArrayList<Integer> > arrListOfarrLists = new ArrayList<ArrayList<Integer> >();
// Creating individual ArrayLists
ArrayList<Integer> arrList1 = new ArrayList<>();
arrList1.add(2);
arrList1.add(4);
arrList1.add(6);
ArrayList<Integer> arrList2 = new ArrayList<>();
arrList2.add(3);
arrList2.add(6);
arrList2.add(9);
ArrayList<Integer> arrList3 = new ArrayList<>();
arrList3.add(5);
arrList3.add(10);
arrList3.add(15);
// Adding ArrayLists to the ArrayList
arrListOfarrLists.add(arrList1);
arrListOfarrLists.add(arrList2);
arrListOfarrLists.add(arrList3);
// Fetching second element of the third ArrayList
ArrayList<Integer> thirdList = arrListOfarrLists.get(2);
int secondElement = thirdList.get(1);
System.out.print("second element of the third ArrayList is: " + secondElement);
}
}
Produção:
second element of the third ArrayList is: 10
Resumo
ArrayList
é provavelmente a coleção mais comumente usada em Java. É uma estrutura de dados simples usada para armazenar elementos do mesmo tipo. Não podemos criar um ArrayList
de tipos primitivos como int. Precisamos usar as classes de wrapper dessas primitivas. A classe ArrayList
fornece métodos convenientes para adicionar e buscar elementos da lista. Também podemos criar um ArrayList
de arrays ou um ArrayList
de ArrayLists
. Eles são usados principalmente para representar dados em um formato de array 2D ou um formato tabular. É melhor usar um ArrayList
de ArrayLists
, pois não limitará seu tamanho.