How to Append Strings in Java Efficiently
-
Use
concat()
from thejava.lang.String
Class to Append Strings in Java -
String Concatenation Using the
+
Operator in Java -
Using the
concat()
Method Fromjava.lang.String
-
Using
StringBuffer
to Concatenating Strings in Java -
Using
StringBuilder
in Concatenating Strings in Java
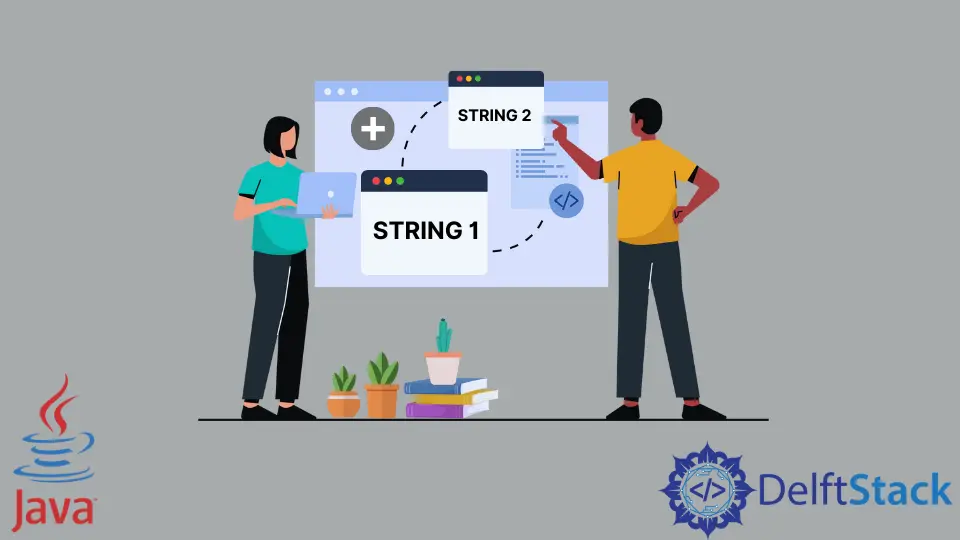
When we talk about string concatenation in Java, the +
operator comes to mind to append strings most efficiently. Since Java does not provide support for overloading, it is special for strings to have such behavior. There are various methods, other than the +
operator, used to append a string in Java that are;
StringBuffer
StringBuilder
Use concat()
from the java.lang.String
Class to Append Strings in Java
Both the StringBuffer
and StringBuilder
classes are used for this purpose. StringBuilder
is given importance because it is the fastest method to concatenate strings. StringBuffer
is given priority secondly due to the synchronized method and because the other methods aside from them are 1000 times slower than these two. Here we will see an example of these four methods.
public class Main {
public static void main(String args[]) {
// 1st method of concat
String str1 = "Most";
str1 = str1.concat(" Welcome");
str1 = str1.concat(" to University");
System.out.println(str1);
// 2nd method of concat
String str2 = "Today";
str2 = str2.concat(" is").concat(" your first").concat(" day");
System.out.println(str2);
}
}
Output:
Most Welcome to University
Today is your first day
String Concatenation Using the +
Operator in Java
This is the simplest method to join strings and numeric values in Java. When the users have two or more primitive values such as short
, char
, or int
at the start of a string concatenation, they must be converted into strings explicitly. For example, System.out.println(300, + 'B'+ "")
will never print 300B, it will print 366 by taking the ASCII
value of B, then adding it to 300. On the other hand, if we use System.out.println('', + 300 +'B')
, then it will print 300B. All +
operator scan be concatenated into various StringBuilder.append()
calls before calling to String()
.
Using the concat()
Method From java.lang.String
The concat(String str)
method concatenates some specific Strings into the end of the string. It allocates the char[]
of length, equal to the combined length of two Strings. This method copies String data into that string and creates a new String object from the private String constructor, which does not copy the input char[]
as given below.
import java.util.Scanner;
public class Main {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the first string: ");
String str1 = sc.next();
System.out.println("Enter the second string: ");
String str2 = sc.next();
String result = str1 + str2;
System.out.println(result);
}
}
Output:
Enter the first string:
United
Enter the second string:
Kingdom
UnitedKingdom
Using StringBuffer
to Concatenating Strings in Java
This is the most appropriate method of concatenating multiple strings, integers, and other parts of Java when StringBuilder
is not available. It works faster than the concat()
and the +
operator methods. The only disadvantage of this approach is that the append methods by StringBuffer
are synchronized. Its working methodology is similar to the StringBuilder
method. It also provides different overloaded append methods to concatenate char
, int
, short
, etc.
public class Main {
public static void main(String args[]) {
StringBuffer sb = new StringBuffer("My ");
sb.append("Country");
System.out.println(sb);
}
}
Output:
My Country
Using StringBuilder
in Concatenating Strings in Java
This is one of the best methods to concatenate strings in Java. Most importantly, when users are concatenating multiple strings. This method is easy and efficient to use when the users are required to append multiple strings. But this method is not commonly used when the users are required to concatenate strings.
public class Main {
public static void main(String args[]) {
StringBuilder sb = new StringBuilder("My ");
sb.append("Country");
System.out.println(sb);
}
}
Output:
My Country