在 Java 中高效地附加字符串
-
concat()
来自java.lang.String
类 -
使用
+
运算符的字符串连接 -
使用
java.lang.String
中的concat()
方法 -
在 Java 中使用
StringBuffer
连接字符串 -
在 Java 中连接字符串时使用
StringBuilder
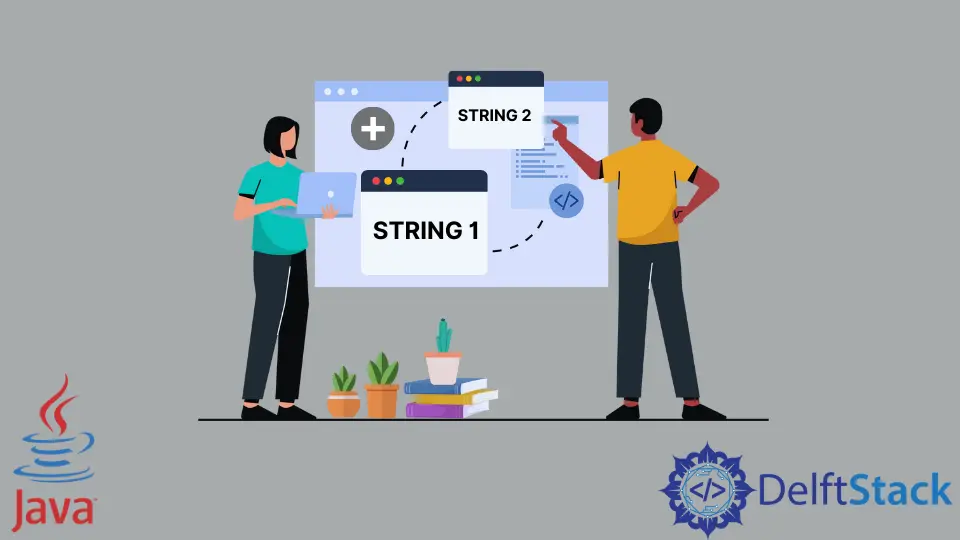
当我们谈论 Java 中的字符串连接时,会想到 +
运算符以最有效地附加字符串。由于 Java 不提供对重载的支持,因此字符串具有这种行为是特殊的。除了 +
操作符之外,还有多种方法用于在 Java 中附加字符串:
StringBuffer
StringBuilder
concat()
来自 java.lang.String
类
StringBuffer
和 StringBuilder
类都用于此目的。StringBuilder
很重要,因为它是连接字符串的最快方法。StringBuffer
由于同步方法而具有第二优先权,并且因为除它们之外的其他方法比这两个方法慢 1000 倍。在这里,我们将看到这四种方法的示例。
public class Main {
public static void main(String args[]) {
// 1st method of concat
String str1 = "Most";
str1 = str1.concat(" Welcome");
str1 = str1.concat(" to University");
System.out.println(str1);
// 2nd method of concat
String str2 = "Today";
str2 = str2.concat(" is").concat(" your first").concat(" day");
System.out.println(str2);
}
}
输出:
Most Welcome to University
Today is your first day
使用 +
运算符的字符串连接
这是在 Java 中连接字符串和数值的最简单方法。当用户在字符串连接的开头有两个或多个原始值(例如 short
、char
或 int
)时,必须将它们显式转换为字符串。例如,System.out.println(300, + 'B'+ "")
永远不会打印 300B,它将通过获取 B 的 ASCII
值,然后将其添加到 300 来打印 366。另一方面, 如果我们使用 System.out.println('', + 300 +'B')
,那么它将打印 300B。在调用 String()
之前,所有 +
运算符扫描都被连接到各种 StringBuilder.append()
调用中。
使用 java.lang.String
中的 concat()
方法
concat(String str)
方法将一些特定的字符串连接到字符串的末尾。它分配长度的 char[]
,等于两个字符串的组合长度。此方法将 String 数据复制到该字符串中,并从私有 String 构造函数创建一个新的 String 对象,该构造函数不会复制输入 char[]
,如下所示。
import java.util.Scanner;
public class Main {
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the first string: ");
String str1 = sc.next();
System.out.println("Enter the second string: ");
String str2 = sc.next();
String result = str1 + str2;
System.out.println(result);
}
}
输出:
Enter the first string:
United
Enter the second string:
Kingdom
UnitedKingdom
在 Java 中使用 StringBuffer
连接字符串
当 StringBuilder
不可用时,这是连接多个字符串、整数和 Java 其他部分的最合适的方法。它比 concat()
和 +
运算符方法工作得更快。这种方法的唯一缺点是 StringBuffer
的 append 方法是同步的。它的工作方法类似于 StringBuilder
方法。它还提供了不同的重载附加方法来连接 char
、int
、short
等。
public class Main {
public static void main(String args[]) {
StringBuffer sb = new StringBuffer("My ");
sb.append("Country");
System.out.println(sb);
}
}
输出:
My Country
在 Java 中连接字符串时使用 StringBuilder
这是在 Java 中连接字符串的最佳方法之一。最重要的是,当用户连接多个字符串时。当用户需要附加多个字符串时,这种方法使用起来简单高效。但是当用户需要连接字符串时,这种方法并不常用。
public class Main {
public static void main(String args[]) {
StringBuilder sb = new StringBuilder("My ");
sb.append("Country");
System.out.println(sb);
}
}
输出:
My Country