How to Iterate Through List in Java
-
Iterate List Elements Using the
for
Loop in Java -
Iterate List Elements Using the
for-each
Loop in Java -
Iterate List Elements Using
while
Loop in Java -
Iterate List Elements Using
forEach()
Method in Java 8 -
Iterate List Elements Using
forEach()
andstream
Method in Java 8 -
Iterate List Elements Using
apache
Library in Java -
Iterate List Elements Using
join()
Method in Java -
Iterate List Elements Using the
map()
Method in Java
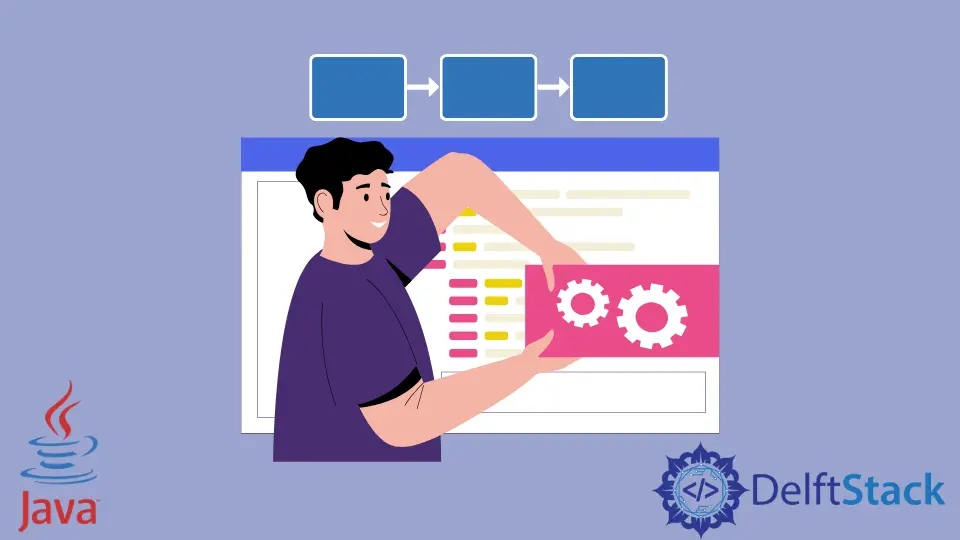
This tutorial introduces how to iterate through the list in Java and lists some example codes to understand the topic.
The list is an interface in Java that has several implementation classes such as ArrayList
, LinkedList
, etc. We can use these classes to store data. The list works as a dynamic array that grows its size when the number of elements increases.
There are several ways to iterate all the elements of a list in Java. For example, the for
loop, the for-each
loop, the forEach()
method with a list or stream, etc. Let’s see some examples.
Iterate List Elements Using the for
Loop in Java
We can use the classic for
loop to iterate each element individually. The for
loop works fine with the objects and primitive values as well. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("India");
list.add("US");
list.add("China");
list.add("Russia");
for (int i = 0; i < list.size(); i++) {
String element = list.get(i);
System.out.println(element);
}
}
}
Output:
India
US
China
Russia
Iterate List Elements Using the for-each
Loop in Java
We can use the for-each loop to print each element individually. The for-each loop works fine with the object’s container like a list, Set
, etc. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("India");
list.add("US");
list.add("China");
list.add("Russia");
for (String country : list) {
System.out.println(country);
}
}
}
Output:
India
US
China
Russia
Iterate List Elements Using while
Loop in Java
As the for
loop, we can use the while
loop to iterate list elements in Java. We fetch elements by using the get()
method and print the elements. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("India");
list.add("US");
list.add("China");
list.add("Russia");
while (!list.isEmpty()) {
String element = list.get(0);
list = list.subList(1, list.size());
System.out.println(element);
}
}
}
Output:
[India, US, China, Russia]
Iterate List Elements Using forEach()
Method in Java 8
If you are using Java 8 or higher version, use the forEach()
method that prints the elements of a list. It takes method reference as a parameter. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("India");
list.add("US");
list.add("China");
list.add("Russia");
list.forEach(System.out::println);
}
}
Output:
India
US
China
Russia
Iterate List Elements Using forEach()
and stream
Method in Java 8
If you are working with stream API, use the forEach()
of stream()
to print out all the list elements. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("India");
list.add("US");
list.add("China");
list.add("Russia");
list.stream().forEach(System.out::println);
}
}
Output:
India
US
China
Russia
Iterate List Elements Using apache
Library in Java
If you are working with the apache library, use the ArrayUtils
class to print out all the list elements. See the example below.
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.lang3.ArrayUtils;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("India");
list.add("US");
list.add("China");
list.add("Russia");
System.out.println(ArrayUtils.toString(list));
}
}
Output:
{India,US,China,Russia}
Iterate List Elements Using join()
Method in Java
If you want to print out all the elements as a single string, you can use the join()
method to join each element of the list and print them using the print()
method. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("India");
list.add("US");
list.add("China");
list.add("Russia");
System.out.print(String.join("\n", list));
}
}
Output:
India
US
China
Russia
Iterate List Elements Using the map()
Method in Java
This is another approach to use the map()
method of stream API with forEach()
. The map()
method can be used to pass a lambda expression to print elements accordingly. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("India");
list.add("US");
list.add("China");
list.add("Russia");
list.stream().map(x -> x).forEach(System.out::println);
}
}
Output:
India
US
China
Russia