How to Instantiate an Object in Java
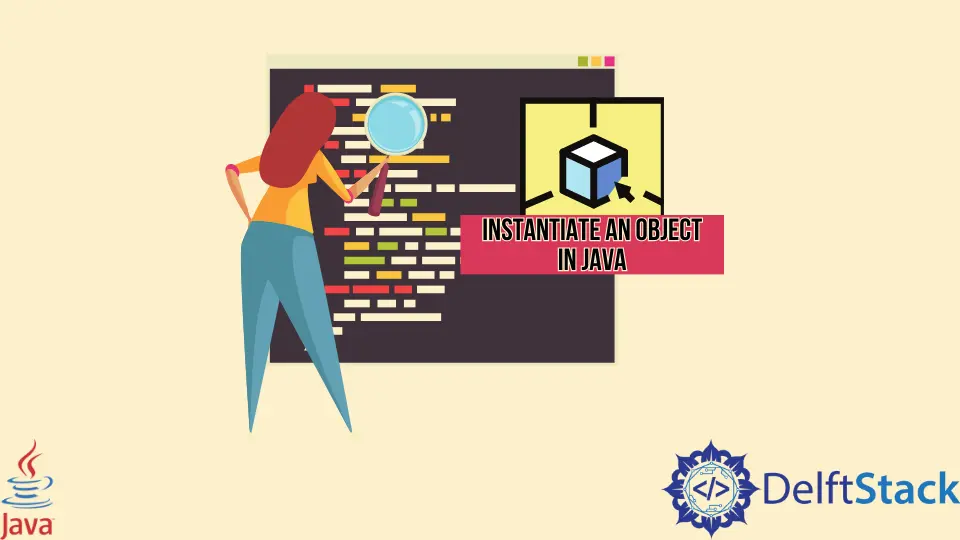
In this tutorial, we will discuss how to instantiate an object in Java, a fundamental concept that every Java developer should master. Understanding object instantiation is crucial for creating and manipulating objects, which are the building blocks of Java applications. Whether you’re a beginner or looking to refresh your knowledge, this guide will walk you through the various methods available for instantiating objects in Java. From using the new
keyword to exploring factory methods, we will cover everything you need to know. So, let’s dive into the world of Java object creation and unlock the power of object-oriented programming.
Using the new
Keyword
The most common way to instantiate an object in Java is by using the new
keyword. This method is straightforward and widely used in various applications. When you declare an object using new
, Java allocates memory for the object and initializes it. Here’s a simple example:
class Dog {
String name;
Dog(String name) {
this.name = name;
}
void bark() {
System.out.println(name + " says Woof!");
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog("Buddy");
myDog.bark();
}
}
Output:
Buddy says Woof!
In this example, we create a class named Dog
with a constructor that takes a String
parameter for the dog’s name. Inside the main
method, we instantiate a Dog
object named myDog
using the new
keyword. This line not only creates the object but also calls the constructor, initializing the name to “Buddy.” Finally, we invoke the bark
method, which prints out a message to the console.
Using the new
keyword is the most direct method for instantiation and is suitable for most scenarios. It’s essential to ensure that the constructor parameters match the object’s requirements to avoid errors during instantiation.
Using Factory Methods
Another popular method for instantiating objects in Java is through factory methods. Instead of creating an object directly with new
, you call a static method that returns an instance of a class. This approach can provide more flexibility and encapsulation. Here’s how it works:
class Cat {
String name;
private Cat(String name) {
this.name = name;
}
public static Cat createCat(String name) {
return new Cat(name);
}
void meow() {
System.out.println(name + " says Meow!");
}
}
public class Main {
public static void main(String[] args) {
Cat myCat = Cat.createCat("Whiskers");
myCat.meow();
}
}
Output:
Whiskers says Meow!
In this example, we define a class Cat
with a private constructor, which prevents direct instantiation. Instead, we provide a static method createCat
that takes a name as a parameter and returns a new Cat
instance. In the main
method, we call Cat.createCat("Whiskers")
to create a Cat
object. This method not only encapsulates the instantiation logic but also allows for additional processing or validation before creating the object.
Factory methods are particularly useful in scenarios where you need to control the instantiation process or when you want to return an instance of a subclass based on certain conditions.
Using the Clone Method
Java also allows object instantiation through the cloning process, which can be particularly useful when you want to create a copy of an existing object. To use cloning, the class must implement the Cloneable
interface and override the clone
method. Here’s an example:
class Bird implements Cloneable {
String name;
Bird(String name) {
this.name = name;
}
@Override
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
void chirp() {
System.out.println(name + " says Chirp!");
}
}
public class Main {
public static void main(String[] args) {
try {
Bird originalBird = new Bird("Tweety");
Bird clonedBird = (Bird) originalBird.clone();
clonedBird.chirp();
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
}
}
Output:
Tweety says Chirp!
In this example, we create a Bird
class that implements the Cloneable
interface. The clone
method is overridden to allow cloning. In the main
method, we first create an instance of Bird
named originalBird
. We then clone this object using the clone
method, resulting in a new Bird
object named clonedBird
. When we call the chirp
method on the cloned object, it outputs the name of the original bird.
Cloning is beneficial when you need a duplicate of an object without creating a new instance from scratch. However, it’s essential to handle CloneNotSupportedException
to ensure that the cloning process runs smoothly.
Conclusion
Instantiating objects in Java is a fundamental skill that every Java programmer should master. Whether you choose to use the new
keyword, factory methods, or the clone method, understanding these techniques will empower you to create and manipulate objects effectively. Each method has its advantages and specific use cases, so it’s essential to choose the right one based on your application’s requirements. Keep practicing these concepts, and you’ll find that object-oriented programming in Java becomes second nature.
FAQ
-
What is object instantiation in Java?
Object instantiation in Java refers to the process of creating an instance of a class, which allows you to use the class’s properties and methods. -
Can I instantiate an object without using the
new
keyword?
Yes, you can instantiate an object using factory methods or by cloning an existing object. -
What is the difference between a constructor and a factory method?
A constructor is a special method used to initialize a new object, while a factory method is a static method that returns an instance of a class, allowing for more flexibility in object creation. -
What is the purpose of the
Cloneable
interface?
TheCloneable
interface allows an object to be cloned, enabling the creation of a copy of an existing object. -
Are there any performance impacts when using factory methods?
Factory methods can introduce a small overhead due to the additional method call, but they can also improve code maintainability and readability.