How to Ignore Upper Case and Lower Case in Java
-
Ignore Case Using
toUppercase()
Method in Java -
Ignore Case Using
toLowerCase()
Method in Java -
Ignore Case Using
equalsIgnoreCase()
Method in Java -
Ignore Case Using
compareToIgnoreCase()
Method in Java
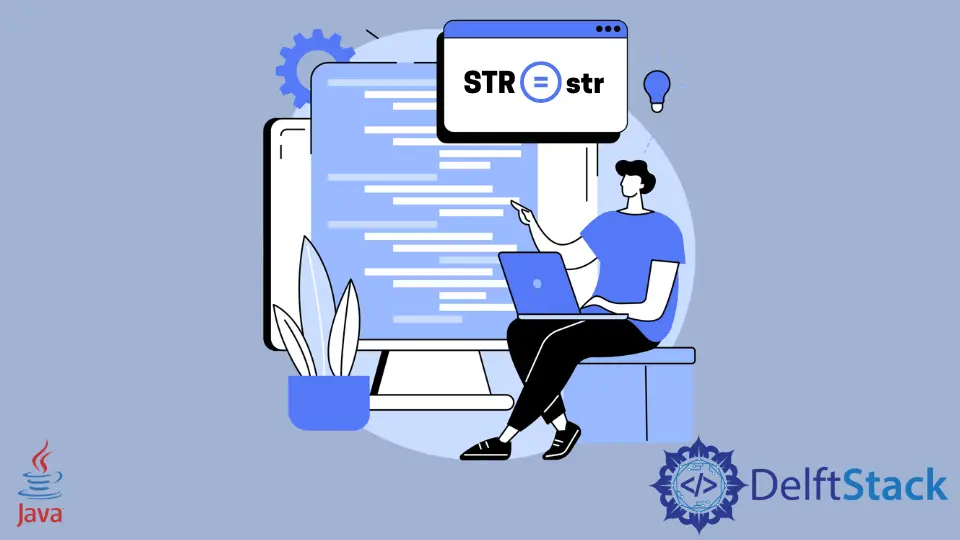
This tutorial introduces how to ignore uppercase and lower case of string in Java.
A string is a sequence of characters, and in Java, it is a class also containing several utility methods. A string can be in lowercase or uppercase or a mix of both, which is fine, but sometimes it creates an issue when comparing strings as each character has a unique Unicode value.
In this article, we will learn to handle the string while ignoring the case. We are familiar with comparing two strings using the equals()
method. This method returns true
when both the strings are equal, including their cases.
For example, look at the example below.
import java.lang.Object;
import java.util.*;
public class SimpleTesting {
public static void main(String args[]) {
String a = "SimpleTesting";
String b = "SimpleTesting";
String c = "SimpleTesting";
a.equals(b); // return false
a.equals(c); // returns true
}
}
This makes us wonder if there is any way to compare two strings while ignoring their cases.
Well, Java provides us with various approaches to achieve this task. Here, we will talk about these approaches in detail:
- Ignore case using
toUppercase()
method - Ignore case using
toLowerCase()
method - Ignore case using
equalsIgnoreCase()
method - Ignore case using
compareToIgnoreCase()
method
Ignore Case Using toUppercase()
Method in Java
We first convert both the strings to uppercase in this approach and then call the equals()
method. The signature for this method is:
public String toUpperCase()
This method converts all the string characters to uppercase based on the default locale. It means that icecream
will be converted to ICECREAM
only if the default locale is Locale.ENGLISH
or Locale.US
.
We might get unpredictable results if the default local is set to another country or language that doesn’t recognize these characters. We can pass the locale to the function call to avoid this.
Let’s now compare two strings using toUpperCase()
and equals()
method in Java. Look at the code below:
import java.lang.Object;
import java.util.*;
public class SimpleTesting {
public static void main(String args[]) {
String desert1 = "icecream";
String desert2 = "IceCream";
// converting to both the strings to upper case
String desert1_converted = desert1.toUpperCase();
String desert2_converted = desert2.toUpperCase();
// comparing both the desert
boolean same_or_not1 = desert1_converted.equals(desert2_converted);
boolean same_or_not2 = desert1.equals(desert2);
System.out.println("Comparison with conversion: " + same_or_not1);
System.out.println("Comparison without conversion: " + same_or_not2);
}
}
Output:
Comparison with conversion: true
Comparison without conversion: false
In the above example, the toUpperCase()
works fine without passing a locale because our default locale is English.
Ignore Case Using toLowerCase()
Method in Java
This method is just like the previous one except that it converts all characters of both the strings to lower case. The method signature is:
public String toLowerCase()
According to the default locale, this procedure changes all of the characters in the string to lowercase.
It means if the default locale is Locale.ENGLISH
or Locale.US
, then ICECREAM
will be converted to icecream
. We might get unpredictable results if the default local is set to another country or language that doesn’t recognize these characters.
We can pass the locale as an argument to the function call to avoid this. Let’s take a closer look at how this feature operates.
Let’s now compare two strings using toLowerCase()
and equals()
method in Java. Look at the code below:
import java.lang.Object;
import java.util.*;
public class SimpleTesting {
public static void main(String args[]) {
String desert1 = "icecream";
String desert2 = "IceCream";
// converting to both the strings to lower case
String desert1_converted = desert1.toLowerCase();
String desert2_converted = desert2.toLowerCase();
// comparing both the desert
boolean same_or_not1 = desert1_converted.equals(desert2_converted);
boolean same_or_not2 = desert1.equals(desert2);
System.out.println("Comparison with conversion: " + same_or_not1);
System.out.println("Comparison without conversion: " + same_or_not2);
}
}
Output:
Comparison with conversion: true
Comparision without conversion: false
Ignore Case Using equalsIgnoreCase()
Method in Java
This method is just like the equals()
method, except that it ignores the case of the strings. Let’s first look at the method signature.
public boolean equalsIgnoreCase(String anotherString)
This method returns true
if two strings are equal after ignoring their cases.
If the length of two strings are the same and the corresponding characters in the two strings are the same, they are regarded as equal, ignoring the case. According to this method, ICECREAM
and icecream
are the same; hence, true
will be returned.
Look at the code below to understand how it works.
import java.lang.Object;
import java.util.*;
public class SimpleTesting {
public static void main(String args[]) {
String desert1 = "icecream";
String desert2 = "IceCream";
// comparing both the deserts
boolean same_or_not1 = desert1.equalsIgnoreCase(desert2);
System.out.println("Comparison : " + same_or_not1);
}
}
Output:
Comparison : true
Ignore Case Using compareToIgnoreCase()
Method in Java
This approach lexicographically compares two strings, disregarding case differences. This method returns an integer equal to the difference between the first two non-equal characters.
Its signature is:
public int compareToIgnoreCase(String str)
If both strings are equivalent, 0
will be returned. Look at the example code below:
import java.lang.Object;
import java.util.*;
public class SimpleTesting {
public static void main(String args[]) {
String desert1 = "icecream";
String desert2 = "IceCream";
// comparing both the deserts
int same_or_not1 = desert1.compareToIgnoreCase(desert2);
System.out.println("Comparision : " + same_or_not1);
}
}
Output:
Comparision : 0