How to Split String to Array in Java
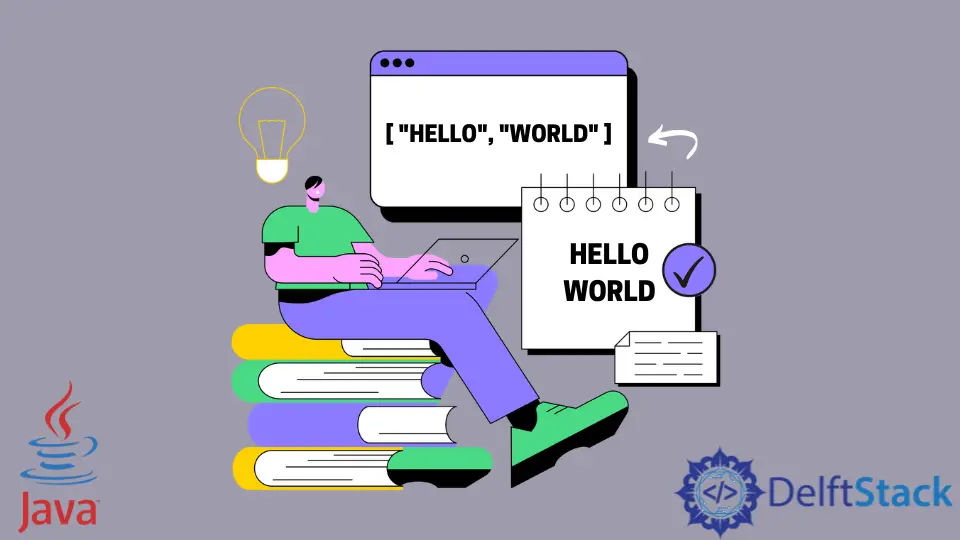
In the world of programming, manipulating strings is a fundamental task, especially when working with data. One common operation is splitting a string into an array. This can be particularly useful when you need to parse data, such as reading CSV files or processing user input. In Java, there are several methods to achieve this, each with its own advantages.
This article will guide you through the different ways to split a string into an array in Java, providing clear examples and explanations to help you understand the process. Whether you are a beginner or looking to refine your skills, this guide will enhance your understanding of string manipulation in Java.
Using the split()
Method
One of the most straightforward ways to split a string into an array in Java is by using the built-in split()
method from the String
class. This method takes a regular expression as an argument and divides the string into substrings based on the specified delimiter.
Here’s a simple example:
public class SplitStringExample {
public static void main(String[] args) {
String str = "Java,Python,C++,JavaScript";
String[] languages = str.split(",");
for (String language : languages) {
System.out.println(language);
}
}
}
Output:
Java
Python
C++
JavaScript
In this example, we have a string that contains programming languages separated by commas. The split(",")
method call splits the string at each comma, resulting in an array of languages. The for
loop then iterates through the array and prints each language on a new line. This method is efficient for simple cases where the delimiter is consistent.
The split()
method also allows you to specify a limit on the number of substrings to return. For instance, if you want to split the string but only get the first two elements, you can pass a limit parameter:
String[] languages = str.split(",", 2);
This would result in an array containing the first language and a second element that includes all remaining languages. This flexibility makes the split()
method a powerful tool for string manipulation in Java.
Using StringTokenizer
Another approach to splitting a string into an array in Java is by using the StringTokenizer
class. This class is part of the java.util
package and provides a simple way to break a string into tokens based on specified delimiters.
Here’s how you can use StringTokenizer
:
import java.util.StringTokenizer;
public class TokenizerExample {
public static void main(String[] args) {
String str = "Java;Python;C++;JavaScript";
StringTokenizer tokenizer = new StringTokenizer(str, ";");
String[] languages = new String[tokenizer.countTokens()];
int index = 0;
while (tokenizer.hasMoreTokens()) {
languages[index++] = tokenizer.nextToken();
}
for (String language : languages) {
System.out.println(language);
}
}
}
Output:
Java
Python
C++
JavaScript
In this example, we use a semicolon as the delimiter. The StringTokenizer
object is created with the input string and the delimiter. We then create an array to hold the tokens and use a loop to extract each token until there are no more tokens left. This method is particularly useful when you want more control over the tokenization process, such as when working with multiple delimiters or requiring more complex parsing logic.
However, it’s worth noting that StringTokenizer
is considered a legacy class. For new projects, it’s generally recommended to use the split()
method or other modern alternatives, such as Scanner
.
Using Scanner to Split a String
The Scanner
class provides another effective way to split strings in Java. It can be particularly useful when reading input from various sources, including files and user input. The Scanner
class allows you to specify delimiters and has built-in methods to handle different data types.
Here’s a practical example using Scanner
:
import java.util.Scanner;
public class ScannerExample {
public static void main(String[] args) {
String str = "Java|Python|C++|JavaScript";
Scanner scanner = new Scanner(str);
scanner.useDelimiter("\\|");
while (scanner.hasNext()) {
System.out.println(scanner.next());
}
scanner.close();
}
}
Output:
Java
Python
C++
JavaScript
In this example, we define a string with languages separated by a pipe (|
). We create a Scanner
object and set the delimiter using the useDelimiter()
method. The loop continues to extract and print each token until there are no more tokens left. This method is particularly powerful when dealing with complex input, as Scanner
can handle various data types and formats seamlessly.
One of the advantages of using Scanner
is its versatility. It can read from different sources, including files and user input, making it a great choice for applications that require dynamic input processing.
Conclusion
Splitting a string into an array in Java is a fundamental skill that can greatly enhance your programming toolkit. Whether you choose to use the split()
method, StringTokenizer
, or Scanner
, each method offers unique advantages depending on your specific needs. As you become more familiar with these techniques, you’ll find that string manipulation becomes a breeze, allowing you to handle data more efficiently. Remember to choose the method that best suits your requirements, and happy coding!
FAQ
-
What is the difference between split() and StringTokenizer?
split() uses regular expressions and is more powerful, while StringTokenizer is simpler but less flexible. -
Can I use multiple delimiters with the
split()
method?
Yes, you can use regular expressions to specify multiple delimiters in thesplit()
method. -
Is StringTokenizer deprecated?
StringTokenizer is not deprecated, but it is considered a legacy class; using split() or Scanner is generally preferred. -
How can I limit the number of splits using split()?
You can pass a limit parameter to thesplit()
method to control the number of substrings returned. -
Can Scanner read from files as well?
Yes, the Scanner class can read from files, user input, and strings, making it very versatile.