How to Split a String in Java
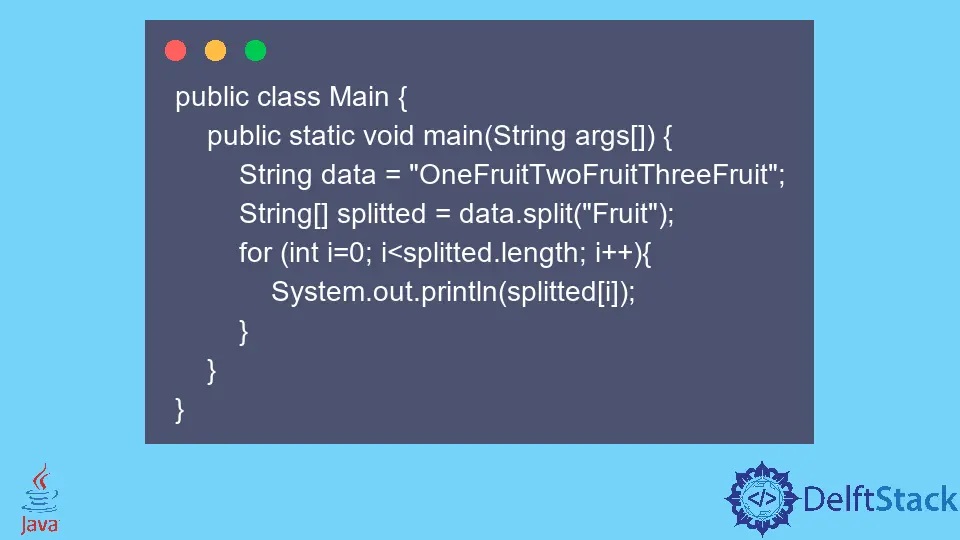
This tutorial discusses how to split a string in Java.
String
in Java is one of the non-primitive datatypes and is used to store a sequence of characters. We can declare a string variable simply as follows:
String string = "I am a string";
String string1 = "I am another string";
We might need to perform several string processing tasks, depending on what we are trying to achieve. Let’s consider the case where we have a string which represents data in a CSV format - all separated by a comma.
String data = "Apple,Banana,Orange,Peach";
We want all the individual strings from this; hence we need to split the string based on the comma to separate the data to get the following output.
> Apple
> Banana
> Orange
> Peach
Java Strings have a split function by default String#split()
which takes a regular expression as input and returns an array of resulting strings after splitting on the given input.
The below example illustrates how to use this method to split a given string in Java.
public class Main {
public static void main(String args[]) {
String data = "Apple,Banana,Orange,Peach";
String[] splitted = data.split(",");
for (int i = 0; i < splitted.length; i++) {
System.out.println(splitted[i]);
}
}
}
The above code outputs the following.
Apple
Banana
Orange
Peach
Let us try this method out to split a string on a different parameter.
Split on a Dot
This time we have a string like this: "One.Two.Three.Four"
. Let us use the same method again to split it.
public class Main {
public static void main(String args[]) {
String data = "One.Two.Three.Four";
String[] splitted = data.split(".");
for (int i = 0; i < splitted.length; i++) {
System.out.println(splitted[i]);
}
}
}
The above code will output nothing! Remember, we mentioned that the split
function takes a regular expression
pattern as input. Therefore, we need to take extra measures when we want to split on some special characters.
There are 12 characters with special meanings:
- the backslash
\
- the caret
^
- the dollar sign
$
- the period or dot
.
- the vertical bar or pipe symbol
|
- the question mark
?
- the asterisk or star
*
- the plus sign
+
- the opening parenthesis
(
- the closing parenthesis
)
- and the opening square bracket
[
- the opening curly brace
{
These special characters are often called metacharacters
.
If we want to split our string on any of the above characters, we can use backslash \
to escape these special characters, so use data.split('\\.')
instead of data.split('.')
.
public class Main {
public static void main(String args[]) {
String data = "One.Two.Three.Four";
String[] splitted = data.split("\\.");
for (int i = 0; i < splitted.length; i++) {
System.out.println(splitted[i]);
}
}
}
The above code now correctly outputs:
One
Two
Three
Four
Let us try one more example.
Split on a String
This time we have a string like this: "OneFruitTwoFruitThreeFruit"
. Let us use the same method again to split it based on the string Fruit
.
public class Main {
public static void main(String args[]) {
String data = "OneFruitTwoFruitThreeFruit";
String[] splitted = data.split("Fruit");
for (int i = 0; i < splitted.length; i++) {
System.out.println(splitted[i]);
}
}
}
The above code outputs:
One
Two
Three
Now we know how to split a string in Java on any given parameter.