How to Sort a Map by Value in Java
-
Sort a
Map<key, value>
Usingsort()
Method in Java -
Sort a
Map<key, value>
Usingsorted()
Method in Java -
Sort a
Map<key, value>
Usingsort()
Method WithComparator
in Java -
Sort a
Map<key, value>
Usingsorted()
andtoMap()
Method in Java -
Sort a
Map<key, value>
Using Custom Code in Java
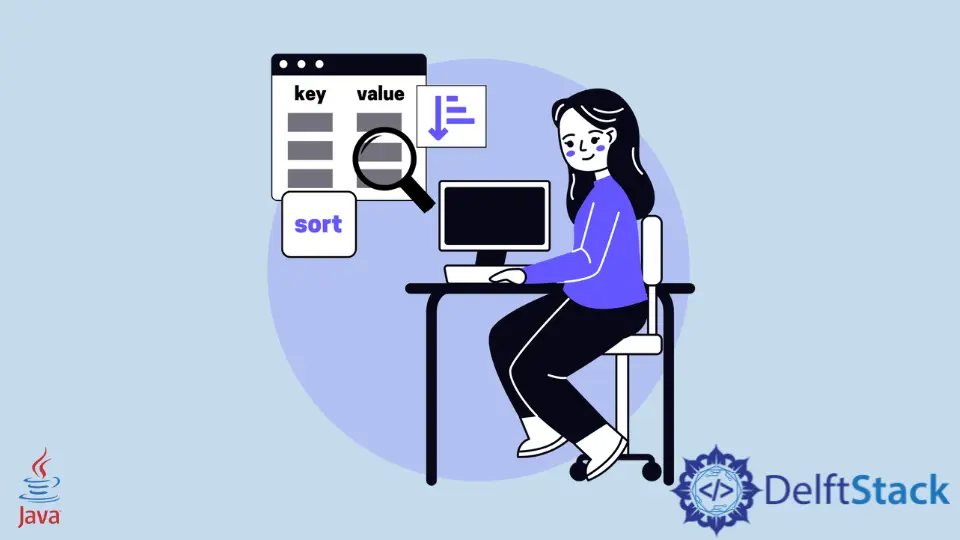
This tutorial introduces how to sort a Map<key, value>
by value in Java and lists some example codes to understand it.
There are several ways to sort a Map<key, value>
. Here we use the sort()
, sorted()
method and comparator interface, etc. Let’s see the examples.
Sort a Map<key, value>
Using sort()
Method in Java
We can use the sort()
method of the List
interface to sort the elements of Map. The sort()
method sorts the elements into ascending order and we specified the sort by value by using the comparingByValue()
method. See the example below.
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
map.forEach((k, v) -> System.out.println(k + "=" + v));
System.out.println("After Sorting by value");
List<Entry<Integer, Integer>> list = new ArrayList<>(map.entrySet());
list.sort(Entry.comparingByValue());
list.forEach(System.out::println);
}
}
Output:
1=100
2=1020
3=300
5=500
After Sorting
1=100
3=300
5=500
2=1020
Sort a Map<key, value>
Using sorted()
Method in Java
If you are working with streams, you can use the sorted()
method that sorts the elements in ascending order. We pass Map.Entry.comparingByValue()
as an argument to the sorted()
method to sort the Map<key, value>
by values.
import java.util.HashMap;
import java.util.Map;
import java.util.stream.Stream;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
map.forEach((k, v) -> System.out.println(k + "=" + v));
System.out.println("After Sorting by value");
Stream<Map.Entry<Integer, Integer>> sorted =
map.entrySet().stream().sorted(Map.Entry.comparingByValue());
sorted.forEach(System.out::println);
}
}
Output:
1=100
2=1020
3=300
5=500
After Sorting by value
1=100
3=300
5=500
2=1020
Sort a Map<key, value>
Using sort()
Method With Comparator
in Java
In this example, we use the compareTo()
method to compare values of Map<key, value>
inside the sort()
method as an argument. You can see that we created an anonymous inner class of the Comparator
interface and defined the compare()
method to compare the values.
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
map.forEach((k, v) -> System.out.println(k + "=" + v));
System.out.println("After Sorting by value");
List<Entry<Integer, Integer>> list = new LinkedList<>(map.entrySet());
Collections.sort(list, new Comparator<Object>() {
@SuppressWarnings("unchecked")
public int compare(Object o1, Object o2) {
return ((Comparable<Integer>) ((Map.Entry<Integer, Integer>) (o1)).getValue())
.compareTo(((Map.Entry<Integer, Integer>) (o2)).getValue());
}
});
Map<Integer, Integer> result = new LinkedHashMap<>();
for (Iterator<Entry<Integer, Integer>> it = list.iterator(); it.hasNext();) {
Map.Entry<Integer, Integer> entry = (Map.Entry<Integer, Integer>) it.next();
result.put(entry.getKey(), entry.getValue());
}
result.forEach((k, v) -> System.out.println(k + "=" + v));
}
}
Output:
1=100
2=1020
3=300
5=500
After Sorting by value
1=100
3=300
5=500
2=1020
Sort a Map<key, value>
Using sorted()
and toMap()
Method in Java
In this example, we are using the sorted()
method to sort the Map<key, value>
and collect the result into LinkedHashMap
using the toMap()
method. Here, we used the method reference concept to create a LinkedHashMap
object.
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.stream.Collectors;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
map.forEach((k, v) -> System.out.println(k + "=" + v));
System.out.println("After Sorting by value");
Map<Integer, Integer> result = map.entrySet()
.stream()
.sorted(Entry.comparingByValue())
.collect(Collectors.toMap(Entry::getKey, Entry::getValue,
(e1, e2) -> e1, LinkedHashMap::new));
result.forEach((k, v) -> System.out.println(k + "=" + v));
}
}
Output:
1=100
2=1020
3=300
5=500
After Sorting by value
1=100
3=300
5=500
2=1020
Sort a Map<key, value>
Using Custom Code in Java
Here, we created a user-defined class that implements the Comparator
interface and passed its object to TreeMap
to get sorted Map<key, value>
by value.
import java.util.Comparator;
import java.util.HashMap;
import java.util.Map;
import java.util.TreeMap;
class UserComparator implements Comparator<Object> {
Map<Integer, Integer> map;
public UserComparator(Map<Integer, Integer> map) {
this.map = map;
}
public int compare(Object o1, Object o2) {
if (map.get(o2) == map.get(o1))
return 1;
else
return ((Integer) map.get(o1)).compareTo((Integer) map.get(o2));
}
}
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
map.forEach((k, v) -> System.out.println(k + "=" + v));
System.out.println("After Sorting by value");
UserComparator comparator = new UserComparator(map);
Map<Integer, Integer> result = new TreeMap<Integer, Integer>(comparator);
result.putAll(map);
result.forEach((k, v) -> System.out.println(k + "=" + v));
}
}
Output:
1=100
2=1020
3=300
5=500
After Sorting by value
1=100
3=300
5=500
2=1020