Anonymous Comparator in Java
- Anonymous Comparator Using Anonymous Class in Java
- Anonymous Comparator Using Lambda Expression in Java
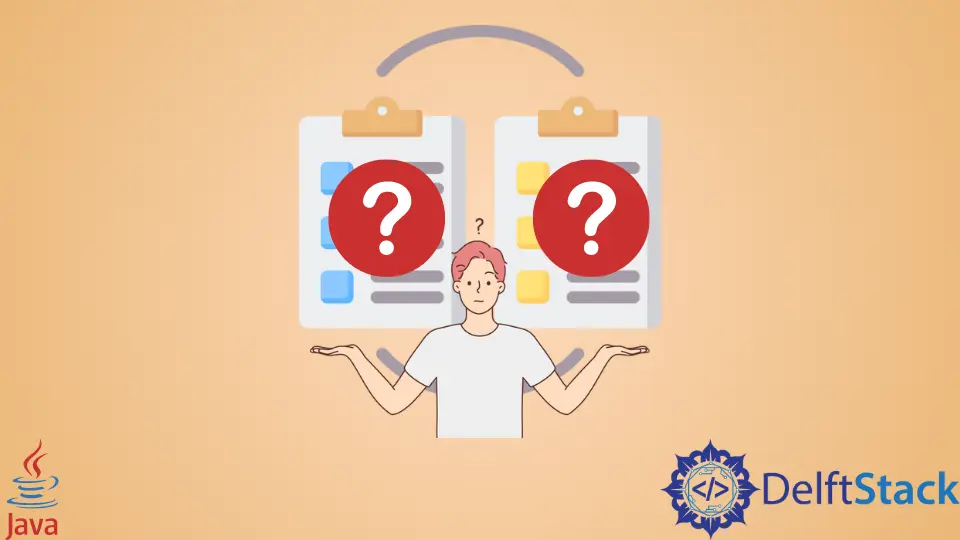
In Java, we can create an anonymous comparator in two ways: one uses the anonymous class, and the other uses Lambda expressions.
This tutorial demonstrates how to create an anonymous comparator in Java.
Anonymous Comparator Using Anonymous Class in Java
To create an anonymous comparator with an anonymous class, follow the steps below.
-
First of all, create a user-defined class.
-
Then, create another class that will be used for the comparator object. This class will include a
collection
class with thesort
method. -
Now, using the anonymous class, create the comparator object and then implement the compare method with the help of the comparator object.
-
The
Sort
method from theCollections
class will be called by passing the object. -
Print or use the sorted information upon your need.
Let’s try an example in Java based on the above steps.
package delftstack;
import java.util.*;
class Employee {
String name;
double salary;
Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
}
public class Anonymous_Comparator {
public static void main(String args[]) {
ArrayList<Employee> Employee_List = new ArrayList<Employee>();
Employee_List.add(new Employee("Mike", 3000));
Employee_List.add(new Employee("Michelle", 2500));
Employee_List.add(new Employee("Tina", 4000));
Employee_List.add(new Employee("Shawn", 5000));
Employee_List.add(new Employee("Jack", 4500));
Employee_List.add(new Employee("Jenny", 3500));
Collections.sort(Employee_List, new Comparator<Employee>() {
public int compare(Employee employee1, Employee employee2) {
if (employee1.salary < employee2.salary) {
return 1;
}
return -1;
}
});
System.out.println("Name\tSalary");
System.out.println("---------------------");
for (Employee Employee : Employee_List) {
System.out.println(Employee.name + " = " + Employee.salary);
}
}
}
The code above creates an anonymous comparator for the class Employee
and sorts the Employee
list according to the salary.
Output:
Name Salary
---------------------
Shawn = 5000.0
Jack = 4500.0
Tina = 4000.0
Jenny = 3500.0
Mike = 3000.0
Michelle = 2500.0
Anonymous Comparator Using Lambda Expression in Java
To create an anonymous comparator with lambda expressions, follow the steps below.
-
First of all, create a user-defined class.
-
Then, create another class that will be used for the comparator object. This class will include a
collection
class with thesort
method. -
Now, using the lambda expressions, create the comparator object interface and then implement the
compare
method with the help of the comparator interface. -
The
Sort
method from theCollections
class will be called by passing the object. -
Print or use the sorted information upon your need.
Let’s try an example in Java based on the above steps.
package delftstack;
import java.util.*;
class Employee {
String name;
double salary;
Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
}
public class Anonymous_Comparator {
public static void main(String args[]) {
ArrayList<Employee> Employee_List = new ArrayList<Employee>();
Employee_List.add(new Employee("Mike", 3000));
Employee_List.add(new Employee("Michelle", 2500));
Employee_List.add(new Employee("Tina", 4000));
Employee_List.add(new Employee("Shawn", 5000));
Employee_List.add(new Employee("Jack", 4500));
Employee_List.add(new Employee("Jenny", 3500));
Collections.sort(Employee_List, (employee1, employee2) -> { // Using Lambda Expressions
if (employee1.salary < employee2.salary) {
return 1;
}
return -1;
});
System.out.println("Name\tSalary");
System.out.println("---------------------");
for (Employee Employee : Employee_List) {
System.out.println(Employee.name + " = " + Employee.salary);
}
}
}
The code above will sort the Employee
using lambda expressions in an anonymous comparator. See output:
Name Salary
---------------------
Shawn = 5000.0
Jack = 4500.0
Tina = 4000.0
Jenny = 3500.0
Mike = 3000.0
Michelle = 2500.0
We created our data classes to understand the problem, but these comparators can also be easily applied to built-in types.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook