How to Sort Comparator in Java
-
Use the
DepartmentComparator
to Sort Elements in Java -
Modify the Program Above Using the
lambda
Function in Java 8
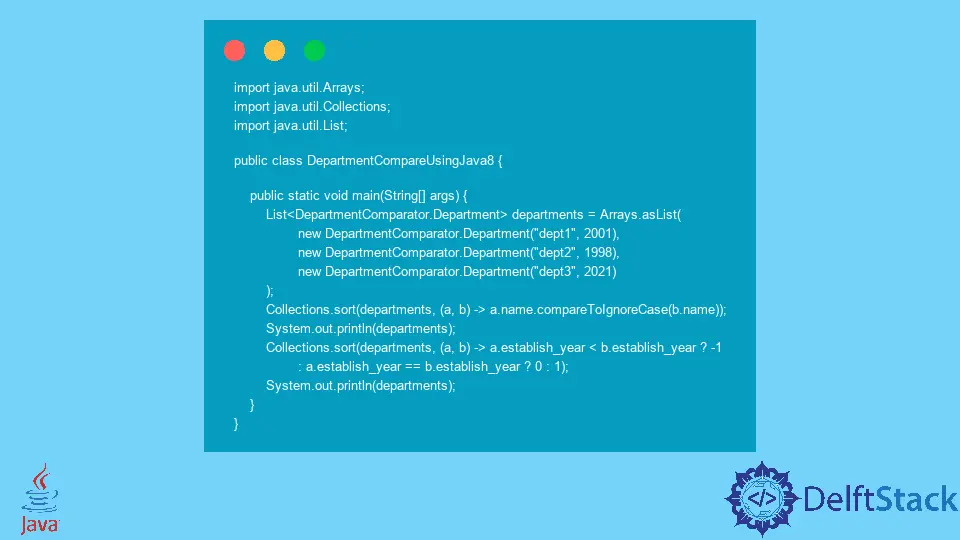
This article defines what a sort comparator in Java is and demonstrates how you can use it in processes. We’ve included programs you can follow to help you understand this concept better.
Use the DepartmentComparator
to Sort Elements in Java
Sorting is the process of arranging the data structures like a list array in a definite sequential order. The process works by comparing the data elements, hence, defining the new positions. There are various types of sort algorithms defined in Java that are useful based on the complexity of the structure.
Below is the code block that defines overriding the comparator interface to give our implementation for sorting the elements.
import java.util.*;
public class DepartmentComparator {
public static void main(String[] args) {
List<Department> departments = Arrays.asList(new Department("dept1", 2001),
new Department("dept2", 1998), new Department("dept3", 2021));
Collections.sort(departments, new LexicographicComparator());
System.out.println("Sorting on the basis of name: " + departments);
Collections.sort(departments, new YearComparator());
System.out.println("Sorting on the basis of year: " + departments);
}
static class LexicographicComparator implements Comparator<Department> {
@Override
public int compare(Department a, Department b) {
return a.name.compareToIgnoreCase(b.name);
}
}
static class YearComparator implements Comparator<Department> {
@Override
public int compare(Department a, Department b) {
return a.establish_year < b.establish_year ? -1
: a.establish_year == b.establish_year ? 0
: 1;
}
}
static class Department {
String name;
int establish_year;
Department(String n, int a) {
name = n;
establish_year = a;
}
@Override
public String toString() {
return String.format("{name=%s, Establish Year=%d}", name, establish_year);
}
}
}
In the program above, the DepartmentComparator
class is a public class that holds the main method and acts as the driver code. Other than the main class, the code has non-public classes that got added to show the functionality. The Department
class is a POJO that holds a field name and the establish_year
variable and overridden toString()
function. Aside from the bean class, a LexicographicComparator
class and a YearComparator
class implement the Comparator
interface.
In the static method, a List
gets initialized with three elements. The Array.asList
is a static method that returns a fixed size List
. The function takes instances of the class to transform into the list. So the department class
instance is created using a new keyword and is now called the parameterized
constructor. This constructor initializes the name and establishes a year from the parameters passed.
Once the list gets created, the sort
method gets invoked of the Collections
class. It sorts the defined list or collection based on the comparator given. The method takes a manual comparator and returns void but modifies the collection passed as an argument. The method throws a ClassCastException
when the elements are of a different type. The method takes a list collection as a first argument and a manual comparator as a second argument.
The LexicographicComparator
and the YearComparator
classes get created for manual comparison. The classes implement a functional interface
that is Comparator
with a single method compare
that returns an int
value based on the comparison. The method gets overridden in the user-defined class where the user-specified implementation can get defined.
In the LexicographicComparator
class, the method specifies a condition based on the name, compares the passed arguments, and returns -1
, 0
, or 1
based on whether the input is lesser, greater than, or equal to each other. Similarly, the YearComparator
method is overridden to compare the year passed as an argument.
Below is the output arranged by names, in ascending order, and by year.
Sorting on the basis of name: [{name=dept1, Establish Year=2001}, {name=dept2, Establish Year=1998}, {name=dept3, Establish Year=2021}]
Sorting on the basis of year: [{name=dept2, Establish Year=1998}, {name=dept1, Establish Year=2001}, {name=dept3, Establish Year=2021}]
Modify the Program Above Using the lambda
Function in Java 8
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class DepartmentCompareUsingJava8 {
public static void main(String[] args) {
List<DepartmentComparator.Department> departments =
Arrays.asList(new DepartmentComparator.Department("dept1", 2001),
new DepartmentComparator.Department("dept2", 1998),
new DepartmentComparator.Department("dept3", 2021));
Collections.sort(departments, (a, b) -> a.name.compareToIgnoreCase(b.name));
System.out.println(departments);
Collections.sort(departments,
(a, b)
-> a.establish_year < b.establish_year ? -1
: a.establish_year == b.establish_year ? 0
: 1);
System.out.println(departments);
}
}
The difference in this program is that instead of defining the new classes that implement the Comparator interface, the Java 8 functional interface
helps reduce the overhead of processing in a new Class each time. The Functional Interface
has a single unimplemented method or abstract
method. It reduces the overhead of creating a class that implements interfaces and gives its own method version.
It uses lambda ()->
functions to call the method directly. Lambdas treat the functions as an argument and do not require any class for the instantiation. The function takes parameters and gives the implementation in the same line instead of in a separate class.
The output of the program above is the same as that of the one in the first code.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn