PriorityQueue Comparator in Java
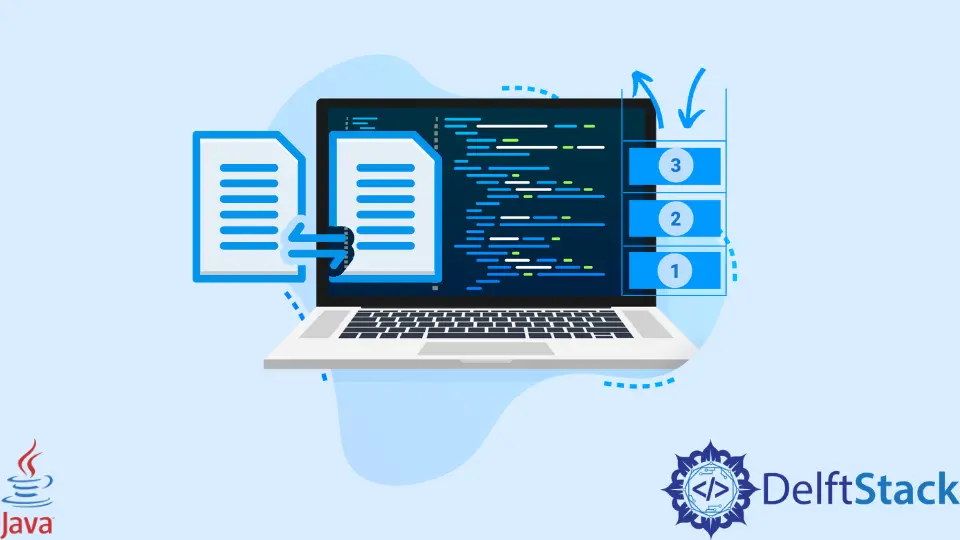
In the priority queue, each element is handled with a particular priority associated with it. That priority is defined in a comparator function associated with the priority queue.
In default, the priority queue is ordered naturally; the comparator is used to give a particular order to the priority queue. This demonstrates the use of a comparator in the priority queue.
Create a Custom Comparator in Java PriorityQueue
Let’s create a custom comparator to sort a PriorityQueue
in descending order.
See example:
package delftstack;
import java.util.Comparator;
import java.util.PriorityQueue;
public class PQ_Comparator {
public static void main(String[] args) {
// Create a priority queue
PriorityQueue<Integer> Demo_PQ = new PriorityQueue<>(new Sort_Comparator());
Demo_PQ.add(3);
Demo_PQ.add(4);
Demo_PQ.add(6);
Demo_PQ.add(5);
Demo_PQ.add(1);
System.out.print("Sorted PriorityQueue According to the comparator: " + Demo_PQ);
}
}
// Comparator class
class Sort_Comparator implements Comparator<Integer> {
@Override
public int compare(Integer x, Integer y) {
if (x < y) {
return 1;
}
if (x > y) {
return -1;
}
return 0;
}
}
The code above creates a custom comparator in the class Sort_Comparator
and uses it in the priority queue to sort it in descending order.
Output:
Sorted PriorityQueue According to the comparator: [6, 5, 4, 3, 1]
Create a Comparator Directly in Java PriorityQueue
We can also create a comparator directly in the priority queue. Let’s sort the priority queue in descending order for the same task.
See example:
package delftstack;
import java.util.*;
public class PQ_Comparator {
public static void main(String[] args) {
// Create a priority queue
PriorityQueue<Integer> Demo_PQ = new PriorityQueue<Integer>(Collections.reverseOrder());
// PriorityQueue<Integer> Demo_PQ = new PriorityQueue<Integer>((a,b) -> b - a);
// PriorityQueue<Integer> Demo_PQ = new PriorityQueue<Integer>((a,b) -> b.compareTo(a));
Demo_PQ.add(3);
Demo_PQ.add(4);
Demo_PQ.add(6);
Demo_PQ.add(5);
Demo_PQ.add(1);
System.out.print("Sorted PriorityQueue According to the comparator: " + Demo_PQ);
}
}
The code above uses the built-in function Collections.reverseOrder
to sort the priority in descending order. The other two comparators given in the comments also perform the same operation.
Output:
Sorted PriorityQueue According to the comparator: [6, 5, 4, 3, 1]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook