PriorityQueue in Java
- Declaration of PriorityQueue in Java
- Adding Element to PriorityQueue
- Removing Elements From PriorityQueue
- Accessing PriorityQueue Elements
- Iterating the PriorityQueue
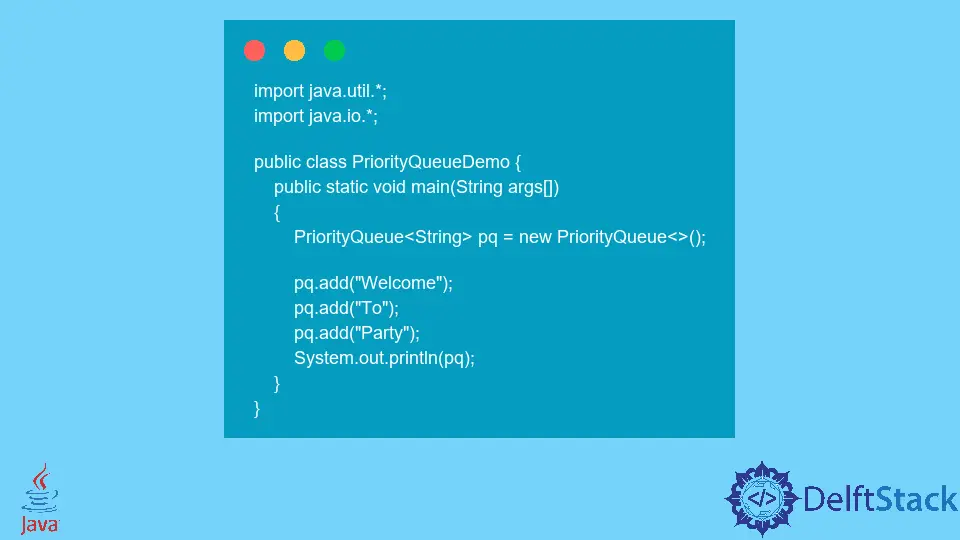
A PriorityQueue is used when the objects have to proceed based on the priority. It is also based on the First-In-First-Out algorithm, but sometimes the elements are required to be proceeded based on priority. That is why PriorityQueue
comes into play. PriorityQueue
is based on a priority heap. The elements in the PriorityQueue
are arranged according to natural ordering, and at queue
construction time, it depends upon which constructor has been used.
Declaration of PriorityQueue in Java
public class PriorityQueue<E> extends AbstractQueue<E> implements Serializable
Here, E is the type of elements held in this queue.
Let’s discuss how the different operations can be performed on the PriorityQueue
class.
Adding Element to PriorityQueue
To include an item to the PriorityQueue
, it is possible to use the add()
method or addition()
method. The order of insertion isn’t saved within the PriorityQueue
. These elements will be stored following the priority order, which will ascend in default.
Example:
import java.io.*;
import java.util.*;
public class PriorityQueueDemo {
public static void main(String args[]) {
PriorityQueue<String> pq = new PriorityQueue<>();
pq.add("Welcome");
pq.add("To");
pq.add("Party");
System.out.println(pq);
}
}
Output:
[To, Welcome, Party]
Removing Elements From PriorityQueue
To remove the element from a PriorityQueue, we can use the delete()
method. If there are several such objects, the first instance of the object is removed. Additionally, it is also possible to use the poll()
method to take the head and then replace it.
import java.io.*;
import java.util.*;
public class PriorityQueueDemo {
public static void main(String args[]) {
PriorityQueue<String> pq = new PriorityQueue<>();
pq.add("Welcome");
pq.add("To");
pq.add("Party");
System.out.println("Initial PriorityQueue " + pq);
pq.remove("Geeks");
System.out.println("After Remove - " + pq);
System.out.println("Poll Method - " + pq.poll());
System.out.println("Final PriorityQueue - " + pq);
}
}
Output:
Initial PriorityQueue [To, Welcome, Party]
After Remove - [To, Welcome]
Poll Method - To
Final PriorityQueue - [Party]
Accessing PriorityQueue Elements
Because Queue
is based on its First In First Out principle, We can only access the head of the Queue
. To gain access to the Queue
elements that are a priority, you can employ the peek()
method.
Example:
// Java program to access elements
// from a PriorityQueue
import java.util.*;
class PriorityQueueDemo {
// Main Method
public static void main(String[] args) {
// Creating a priority queue
PriorityQueue<String> pq = new PriorityQueue<>();
pq.add("Welcome");
pq.add("To");
pq.add("Party");
System.out.println("PriorityQueue: " + pq);
// Using the peek() method
String element = pq.peek();
System.out.println("Accessed Element: " + element);
}
}
Output:
PriorityQueue: [To, Welcome, Party]
Accessed Element: To
Iterating the PriorityQueue
There are many ways to iterate over the PriorityQueue
. The most well-known method is to convert the queue
into an array and then traverse it using the for a loop. In addition, the queue
comes with an internal iterator that can be used to cycle across the queue
.
Example:
import java.util.*;
public class PriorityQueueDemo {
public static void main(String args[]) {
PriorityQueue<String> pq = new PriorityQueue<>();
pq.add("Welcome");
pq.add("To");
pq.add("Party");
Iterator iterator = pq.iterator();
while (iterator.hasNext()) {
System.out.print(iterator.next() + " ");
}
}
}
Output:
To Welcome Party