Enqueue and Dequeue in Java
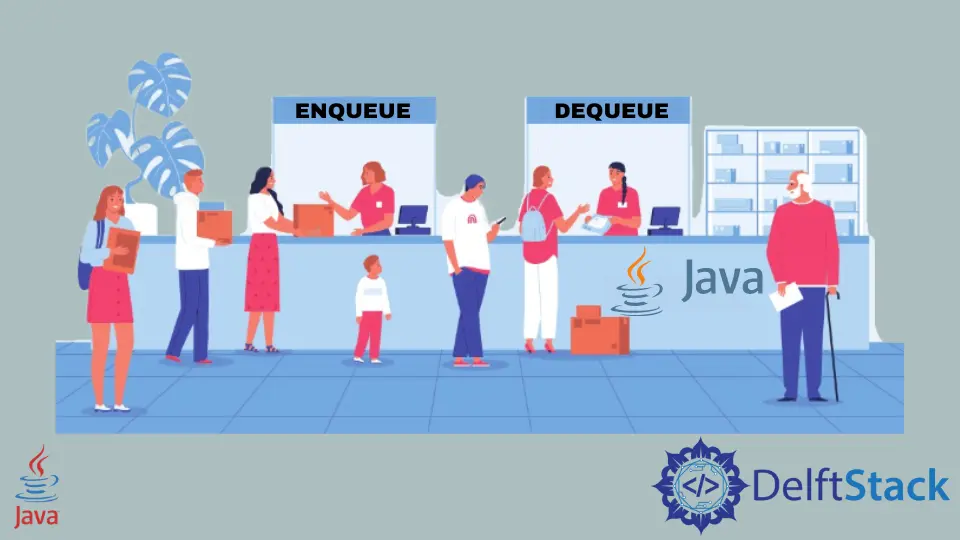
This article provides a concise exploration of queues in Java, encompassing their definition, enqueue and dequeue operations, key methods within the Queue interface, and the utilization of these methods in the LinkedList
class for effective data manipulation.
Queue
A queue in Java is a data structure that follows the First-In-First-Out (FIFO) principle, where elements are added to the rear and removed from the front. It ensures that the oldest element is processed first.
Queues are crucial in Java for managing tasks, scheduling processes, and handling asynchronous events. They facilitate orderly execution in scenarios like multi-threading, event-driven programming, and network communication.
By maintaining a predictable order of operations, queues enhance efficiency and coordination, making them essential for various applications, from handling background jobs to managing input/output requests in a systematic and organized manner.
Enqueue
Enqueue in Java refers to adding an element to the end of a data structure, like a queue. It’s crucial for maintaining order in a First-In-First-Out (FIFO) manner.
Enqueue operations are essential for tasks such as task scheduling, asynchronous event handling, and managing elements in a systematic way. In Java, enqueue is commonly used in conjunction with queues and other data structures where preserving the order of insertion is vital.
This operation ensures that elements are processed in the sequence they were added, playing a fundamental role in achieving organized and predictable behavior in various programming scenarios.
Dequeue
A dequeue (Double-Ended Queue) in Java is a versatile data structure that allows the insertion and removal of elements from both ends. It supports operations like enqueue and dequeue at both the front and rear.
Dequeues are crucial in Java for scenarios requiring flexibility in data manipulation, such as implementing efficient algorithms for sorting, sliding window problems, and managing collections where elements need to be added or removed from both ends. This versatility makes deques valuable in scenarios where a combination of queue and stack functionalities is necessary, providing a powerful tool for various programming tasks requiring dynamic data handling in a structured and adaptable manner.
So, when we say enqueue and dequeue in programming, we mean adding and removing an item, respectively. Take a look at the picture down below.
As you can see, addition (enqueue) in the queue will always be from the back, and removal (dequeue) will always be from the front. Now that you have a concrete understanding of the queue let’s take a look at the implementation of the queue in Java.
Methods for Enqueue and Dequeue
Queue Interface
Knowing the methods for enqueue and dequeue in the Java Queue interface is crucial for efficiently managing data in a first-in-first-out (FIFO) manner.
Enqueue Methods | Description |
---|---|
add(E e) |
Adds the specified element to the end of the queue. |
offer(E e) |
Inserts the specified element into the queue if it is possible to do so without violating capacity restrictions. |
Enqueue methods (add
and offer
) allow you to add elements to the end of the queue, while dequeue methods (remove
, poll
, element
, and peek
) enable you to retrieve and remove elements from the front of the queue.
Dequeue Methods | Description |
---|---|
remove() |
Retrieves and removes the head of the queue. Throws an exception if the queue is empty. |
poll() |
Retrieves and removes the head of the queue or returns null if the queue is empty. |
element() |
Retrieves, but does not remove, the head of the queue. Throws an exception if the queue is empty. |
peek() |
Retrieves, but does not remove, the head of the queue or returns null if the queue is empty. |
This is essential for implementing various algorithms and data structures where the order and timing of operations matter, such as task scheduling, event handling, and network communication. Understanding and using these methods properly helps ensure the correct and predictable behavior of your queue-based operations in Java.
LinkedList
Class
Understanding the enqueue and dequeue methods in the Java LinkedList
class is essential for the effective manipulation of data in a linked list structure.
Enqueue Methods | Description |
---|---|
add(E e) |
Adds the specified element to the end of the list. |
offer(E e) |
Inserts the specified element into the list if it is possible to do so without violating capacity restrictions. |
addLast(E e) |
Adds the specified element to the end of the list. |
Enqueue methods (add
, offer
, and addLast
) empower you to add elements to the end of the list. On the other hand, dequeue methods (remove
, poll
, removeFirst
, and pollFirst
) allow you to retrieve and remove elements from the front of the list.
Dequeue Methods | Description |
---|---|
remove() |
Retrieves and removes the head of the list. Throws an exception if the list is empty. |
poll() |
Retrieves and removes the head of the list or returns null if the list is empty. |
removeFirst() |
Removes and returns the first element of the list. Throws an exception if the list is empty. |
pollFirst() |
Removes and returns the first element of the list or returns null if the list is empty. |
This knowledge is crucial for implementing dynamic data structures and algorithms, facilitating efficient insertion and removal operations. Whether managing a queue or maintaining an ordered collection, mastering these methods in LinkedList
ensures the smooth and predictable handling of your data in Java.
Enqueue and Dequeue in Java
Queue
Interface
The Queue
interface in Java abstracts the enqueue and dequeue operations, providing a standardized way to handle queues. It allows for interchangeable implementations, offering flexibility in choosing data structures.
This abstraction is vital for writing modular and maintainable code, as it enables seamless transitions between different queue implementations, enhancing code adaptability and reducing dependencies on specific data structures like LinkedList
.
In this article, we will explore the code example below, breaking down each step to explain the nuances of methods such as add()
, offer()
, remove()
, poll()
, element()
, and peek()
.
Code Example:
import java.util.LinkedList;
import java.util.Queue;
public class QueueInterfaceExample {
public static void main(String[] args) {
// Creating a Queue using LinkedList
Queue<String> queue = new LinkedList<>();
// Enqueueing elements using various methods
queue.add("Element 1");
queue.offer("Element 2");
// Dequeueing elements using various methods
String dequeuedElement1 = queue.remove();
String dequeuedElement2 = queue.poll();
// Displaying dequeued elements
System.out.println("Dequeued Element 1: " + dequeuedElement1);
System.out.println("Dequeued Element 2: " + dequeuedElement2);
// Peeking at the head of the queue using peek()
String peekedElement = queue.peek();
// Displaying the element at the head without removing it
System.out.println("Peeked Element: " + peekedElement);
try {
// Attempting to use element() on an empty queue
String elementAtHead = queue.element();
System.out.println("Element at Head: " + elementAtHead);
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
}
}
Let’s dissect the code and comprehend the intricacies of each step, elucidating the significance of each method. Commencing with the creation of a Queue
utilizing a LinkedList
, a prevalent implementation of the Queue
interface in Java, we proceed to the enqueueing phase.
Employing methods such as add
and offer
, we add elements to the queue. Subsequently, dequeueing operations are executed through remove
and poll
methods, with displayed results.
Introducing non-destructive inspection, we use peek
to retrieve the head without removal. Finally, we attempt to use element
, akin to peek
, but with exception handling to prevent program termination when the queue is empty.
The output illustrates the successful execution of enqueue and dequeue operations, along with the outcomes of peeking and attempting to use element()
on an empty queue. This serves as a practical guide for developers looking to master queue operations in Java.
By navigating through this code example, we’ve explored the essence of enqueueing and dequeuing in Java using Queue
interface methods. Understanding these methods empowers developers to handle data systematically, ensuring efficient and predictable execution.
LinkedList
Using a LinkedList
for enqueueing and dequeueing in Java provides a flexible and efficient solution. Unlike the Queue
interface, LinkedList
allows for easy insertion and removal at both ends, enabling seamless implementation of enqueue and dequeue operations.
This flexibility is crucial for scenarios where dynamic manipulation of data is essential for optimizing code performance, such as efficiently managing a queue.
In this article, we will delve into the various methods available in Java for enqueueing and dequeuing, including add()
, offer()
, addLast()
, remove()
, poll()
, removeFirst()
, and pollFirst()
. Let’s explore these methods through a comprehensive example.
Code Example:
import java.util.LinkedList;
public class EnqueueDequeueExample {
public static void main(String[] args) {
// Creating a LinkedList to simulate a queue
LinkedList<String> queue = new LinkedList<>();
// Enqueueing elements using various methods
queue.add("Element 1"); // Equivalent to addLast()
queue.offer("Element 2");
queue.addLast("Element 3");
// Dequeueing elements using various methods
String dequeuedElement1 = queue.remove(); // Equivalent to removeFirst()
String dequeuedElement2 = queue.poll();
String dequeuedElement3 = queue.pollFirst(); // Equivalent to removeFirst()
// Displaying dequeued elements
System.out.println("Dequeued Element 1: " + dequeuedElement1);
System.out.println("Dequeued Element 2: " + dequeuedElement2);
System.out.println("Dequeued Element 3: " + dequeuedElement3);
}
}
Let’s delve into the code to grasp the intricacies of each step and understand the significance of the methods employed. Initially, we created a LinkedList
named queue
to serve as our simulated queue.
Moving on to the enqueueing phase, we utilize three distinct methods: add("Element 1")
to append Element 1
to the end of the queue, offer("Element 2")
for inserting Element 2
, and addLast("Element 3")
to add Element 3
to the queue’s tail. For dequeuing operations, we employ three different methods: remove()
to retrieve and eliminate the queue’s head (akin to removeFirst()
), poll()
to retrieve and remove the head while returning null if the queue is empty, and pollFirst()
to remove and return the first element of the queue (equivalent to removeFirst()
).
Finally, we display the elements that underwent dequeuing through the diverse methods employed.
The output demonstrates the successful enqueueing and dequeueing operations, showcasing the effectiveness of these methods in managing a simulated queue in Java.
Understanding the nuances of enqueueing and dequeuing in Java is crucial for effective data management. The versatility offered by methods like add()
, offer()
, addLast()
, remove()
, poll()
, removeFirst()
, and pollFirst()
allows developers to tailor their code to specific requirements.
By mastering these methods, you empower yourself to efficiently handle tasks that demand orderly processing of data, contributing to the creation of robust and responsive Java applications.
Conclusion
Queue in Java is a vital data structure adhering to the First-In-First-Out (FIFO) principle. Enqueueing involves adding elements to the end, while dequeueing is the removal of elements from the front.
The Queue
interface provides standardized methods like add
, offer
, remove
, poll
, element
, and peek
. Utilizing a LinkedList
for enqueueing and dequeueing offers flexibility, while the Queue
interface abstracts these operations, allowing for interchangeable implementations.
Mastering these methods ensures efficient and orderly data manipulation, providing developers with the tools to build responsive and well-structured Java applications.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn