FIFO Queue in Java
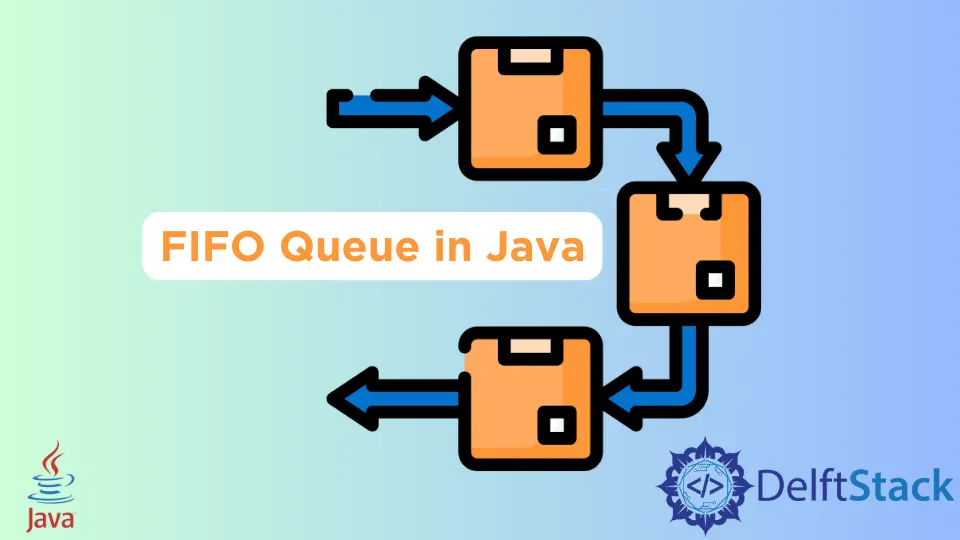
A queue is a linear data structure that implements the first-in-first-out concept. The concept means that the elements that get in first are the first to come out.
This article delves into the implementation of “First In, First Out” (FIFO) queues in Java, exploring different methods to create them. A FIFO queue is a fundamental data structure where the first element added is the first to be removed, which is crucial in scenarios where maintaining the order of elements is essential.
The methods discussed include utilizing specific classes like LinkedList
and ArrayDeque
and leveraging the Queue
interface. Each method has its advantages, offering developers flexibility, efficiency, or abstraction based on specific programming requirements.
FIFO Queue in Java
A FIFO Queue in Java stands for “First In, First Out”. It is a data structure that follows the principle that the first element added to the queue will be the first one to be removed.
This means that the order of elements in the queue is preserved, and when you add new elements, they are placed at the end of the queue. When you remove elements, they are taken from the front of the queue.
In simpler terms, imagine a queue of people waiting in line; the person who arrives first is the first to be served. Similarly, in a FIFO queue, the element that is enqueued first is the first to be dequeued.
This concept is essential for managing tasks, processes, or data in a sequential and ordered manner within a Java program.
Methods to Create FIFO Queue
Creating a FIFO queue is a fundamental task in programming, particularly when order matters in data processing. In Java, several methods can be employed to implement a FIFO queue, each with its own advantages and use cases.
These methods range from utilizing specific classes to offering flexibility and abstraction. Here are some common methods to create a FIFO queue in Java:
Approach | Description |
---|---|
LinkedList |
You can use the LinkedList class in Java, which provides methods for adding elements at the end and removing elements from the beginning, making it suitable for implementing a FIFO queue. |
ArrayDeque |
ArrayDeque is another class that can be used to implement a FIFO queue. It provides constant time complexity for adding and removing elements from both ends. |
Queue Interface |
The Queue interface adds a layer of abstraction, making your code more adaptable, consistent, and interoperable. It aligns with best practices in Java programming, enhancing encapsulation and preparing your code for potential future changes. |
Choose the implementation that best fits your requirements and the specific characteristics you need for your FIFO queue.
FIFO Queue in Java Using LinkedList
Using LinkedList
in creating a FIFO queue in Java provides flexibility and simplicity. Its dynamic structure allows for efficient insertion and deletion at both ends of the list, making it suitable for implementing a FIFO queue.
While not as fast as ArrayDeque
in certain scenarios, LinkedList
excels when there’s a mix of frequent insertions and removals. Its ease of use and straightforward implementation make it a practical choice for scenarios where the overhead of a doubly-linked list structure doesn’t significantly impact performance.
The simplicity and adaptability of LinkedList
make it a valuable option for implementing FIFO queues in Java.
Let’s dive into a complete working example that demonstrates the implementation of a FIFO queue using the LinkedList
class in Java. The example will cover adding elements to the queue, removing elements from the queue, and displaying the contents of the entire queue.
import java.util.LinkedList;
public class FIFOQueueExample {
public static void main(String[] args) {
// Creating a FIFO queue using LinkedList
LinkedList<String> fifoQueue = new LinkedList<>();
// Adding elements to the queue
fifoQueue.add("First");
fifoQueue.add("Second");
fifoQueue.add("Third");
// Displaying the initial queue
System.out.println("Initial Queue: " + fifoQueue);
// Removing the first element from the queue
String removedElement = fifoQueue.removeFirst();
System.out.println("Removed Element: " + removedElement);
// Displaying the updated queue
System.out.println("Updated Queue: " + fifoQueue);
}
}
In the process of understanding the code for implementing a FIFO queue in Java, let’s break down each crucial step. Initially, we create a Java class named FIFOQueueExample
, housing the main
method, which serves as the program’s entry point.
Following this, we instantiate a FIFO queue using the LinkedList
class, specifying that it will store elements of type String
. Subsequently, we proceed to add three elements (First
, Second
, and Third
) to the queue through the add
method of the LinkedList
class.
The initial state of the queue is then displayed on the console. Moving forward, we remove the first element from the queue utilizing the removeFirst
method, storing the removed element in a variable.
Finally, we print the updated contents of the queue after the removal operation. This sequential breakdown offers a comprehensive understanding of the code’s functionality.
The output of the program will be:
This output demonstrates the FIFO behavior, where the First
element added to the queue is the first one removed, resulting in an updated queue without the removed element.
Utilizing the LinkedList
class in Java to implement a FIFO queue provides a flexible and efficient solution for managing elements in a sequential manner. This example showcases the simplicity and effectiveness of this approach, making it suitable for various applications requiring ordered processing of data.
FIFO Queue in Java Using ArrayDeque
Using ArrayDeque
in creating a FIFO queue in Java offers efficiency and constant-time operations for both enqueue and dequeue, making it a superior choice. Unlike LinkedList
, ArrayDeque
doesn’t incur the same overhead for node structures, resulting in faster performance.
Additionally, it provides a dynamic array-based implementation, ensuring better memory utilization compared to a fixed-size array. The simplicity and speed of ArrayDeque
make it a preferred option for scenarios where fast and balanced enqueue and dequeue operations are crucial, making it a compelling choice for implementing efficient FIFO queues in Java.
Let’s delve into a complete working example that showcases the implementation of a FIFO queue using the ArrayDeque
class in Java. The example covers adding elements to the queue, removing elements from the queue, and displaying the entire contents of the queue.
import java.util.ArrayDeque;
public class FIFOQueueExample {
public static void main(String[] args) {
// Creating a FIFO queue using ArrayDeque
ArrayDeque<String> fifoQueue = new ArrayDeque<>();
// Adding elements to the queue
fifoQueue.add("First");
fifoQueue.add("Second");
fifoQueue.add("Third");
// Displaying the initial queue
System.out.println("Initial Queue: " + fifoQueue);
// Removing the first element from the queue
String removedElement = fifoQueue.poll();
System.out.println("Removed Element: " + removedElement);
// Displaying the updated queue
System.out.println("Updated Queue: " + fifoQueue);
}
}
In understanding the code for implementing a FIFO queue in Java, we take a step-by-step approach to elucidate each essential segment. The process initiates with the creation of a Java class named FIFOQueueExample
, featuring the main
method as the program’s entry point.
Subsequently, a FIFO queue is instantiated using the ArrayDeque
class, specifying that it will store elements of type String
. Following this, we proceed to add three elements (First
, Second
, and Third
) to the queue through the add
method of the ArrayDeque
class.
The initial state of the queue is then displayed on the console. Moving forward, we remove the first element from the queue utilizing the poll
method, storing the removed element in a variable.
Finally, we print the updated contents of the queue after the removal operation, providing a comprehensive comprehension of the code’s functionality.
The output of the program will be:
This output demonstrates the FIFO behavior, where the First
element added to the queue is the first one removed, resulting in an updated queue without the removed element.
The use of ArrayDeque
in Java to implement a FIFO queue provides an efficient and straightforward solution for managing elements in a sequential manner. This example illustrates the ease of use and effectiveness of this approach, making it an excellent choice for applications requiring ordered processing of data.
FIFO Queue in Java Using Queue
Interface
Using the Queue
interface in creating a FIFO queue in Java is crucial for abstraction and adaptability. It allows for a consistent and interchangeable approach to queuing, making it easier to switch between different implementations, such as LinkedList
or ArrayDeque
, without modifying the code that uses the queue.
This interface-based design promotes cleaner code and better encapsulation and aligns with Java’s standard practices. The use of Queue
enhances code readability and future-proofs the application, ensuring seamless integration with other Java libraries and facilitating maintenance and updates in the long run.
Let’s dive into a complete working example that illustrates the implementation of a FIFO queue using the Queue
interface in Java. The example covers adding elements to the queue, removing elements from the queue, and displaying the entire contents of the queue.
import java.util.LinkedList;
import java.util.Queue;
public class FIFOQueueExample {
public static void main(String[] args) {
// Creating a FIFO queue using the Queue interface with LinkedList implementation
Queue<String> fifoQueue = new LinkedList<>();
// Adding elements to the queue
fifoQueue.offer("First");
fifoQueue.offer("Second");
fifoQueue.offer("Third");
// Displaying the initial queue
System.out.println("Initial Queue: " + fifoQueue);
// Removing the first element from the queue
String removedElement = fifoQueue.poll();
System.out.println("Removed Element: " + removedElement);
// Displaying the updated queue
System.out.println("Updated Queue: " + fifoQueue);
}
}
In the explanation of the code for implementing a FIFO queue in Java, each segment is systematically elucidated. The initiation involves the creation of a Java class named FIFOQueueExample
, with the main
method serving as the program’s entry point.
Subsequently, a FIFO queue is created using the Queue
interface, with the underlying implementation chosen as LinkedList
. This queue is designated to store elements of type String
.
Following this, three elements (First
, Second
, and Third
) are added to the queue using the offer
method of the Queue
interface. The initial state of the queue is then displayed on the console.
Moving forward, we remove the first element from the queue using the poll
method, storing the removed element in a variable. Finally, we print the updated contents of the queue after the removal operation, offering a comprehensive understanding of the code’s functionality.
The output of the program will be:
This output exemplifies the FIFO behavior, where the First
element added to the queue is the first one removed. The resulting updated queue demonstrates the effectiveness of the Queue
interface in maintaining the order of elements.
In conclusion, leveraging the Queue
interface in Java to implement a FIFO queue offers a versatile and standardized solution. This example showcases the simplicity and efficiency of this approach, making it a valuable choice for applications requiring the ordered processing of data.
Conclusion
Implementing a First In, First Out (FIFO) queue in Java is a fundamental aspect of programming, particularly in scenarios where maintaining order is critical. Throughout the article, we explored various methods to create a FIFO queue, each with its unique advantages.
Using the LinkedList
class offers flexibility, while ArrayDeque
provides efficiency with constant-time operations. Leveraging the Queue
interface ensures adaptability and abstraction, allowing for seamless transitions between different implementations.
Whether simplicity, speed, or abstraction is prioritized, Java provides versatile tools to cater to diverse programming needs when it comes to FIFO queues. Choosing the appropriate method depends on specific requirements, offering developers the flexibility to optimize their code for various scenarios.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn