Queue offer vs add in Java
-
the
offer()
Method -
the
add()
Method -
Main Differences Between the
offer()
andadd()
Methods - Conclusion
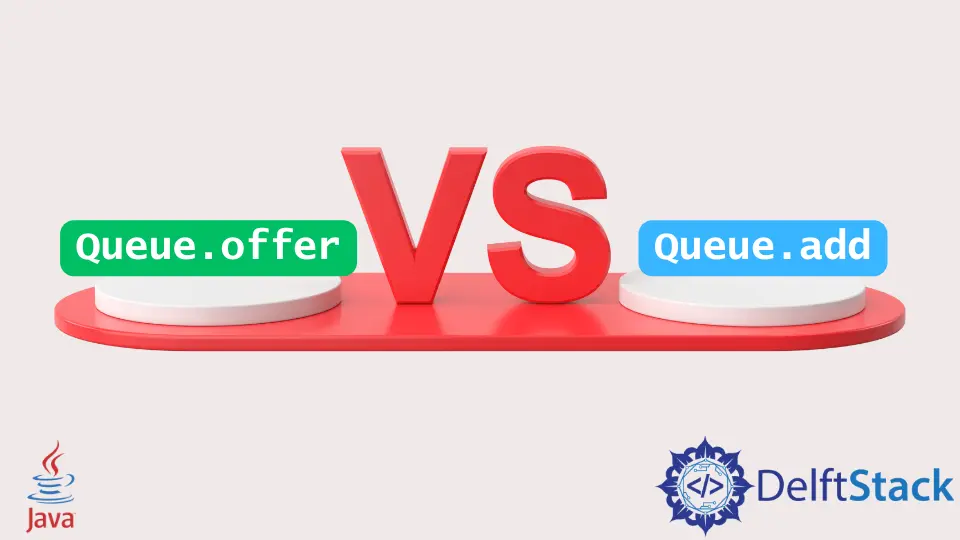
The article explores the functionalities of Java’s offer()
and add()
methods in the Queue
interface, emphasizing their shared purpose of adding elements and delineating key differences in their behaviors, offering developers foundational insights.
the offer()
Method
In Java, the offer()
method is part of the Queue
interface and is used to add an element to the queue. It attempts to add the specified element to the queue, returning true
if successful.
Suppose the queue is at its capacity and cannot accept more elements; offer()
returns false
without throwing an exception. This method provides a non-blocking way to add elements to the queue, making it suitable for scenarios where gracefully handling a full queue is preferred over throwing an exception.
the add()
Method
In Java, the add()
method is part of the Queue
interface and is used to add an element to the queue. It attempts to add the specified element to the queue and returns true
upon success.
If the queue is at its capacity and cannot accept more elements, it throws an IllegalStateException
. Unlike the offer()
method, add()
is more assertive and can be used when it is expected that the element should always be added successfully.
Main Differences Between the offer()
and add()
Methods
In Java, the Queue
interface is part of the Java Collections Framework and provides a way to represent a collection of elements in a specific order. Two methods commonly used in the Queue
interface are offer()
and add()
.
Here are the main differences between them:
Aspect | offer() |
add() |
---|---|---|
Return Type | Returns true if the element was successfully added to the queue and false if the queue is full or otherwise cannot accept the element at the moment. |
Throws an IllegalStateException if the element cannot be added at the moment, typically when the queue is full. |
Behavior on Capacity Exceeded | If the queue is at its capacity and cannot accept more elements, it returns false . |
If the queue is at its capacity and cannot accept more elements, it throws an IllegalStateException . |
Compatibility with Collection Interface |
Is part of the Queue interface and also inherited from the Collection interface. |
Is part of the Queue interface but is not directly inherited from the Collection interface. |
Exception Handling | Does not throw exceptions on failure; it simply returns false . |
Throws an IllegalStateException if the element cannot be added, for example, due to capacity restrictions. |
Use Case | Often used in scenarios where you want to attempt to add an element to the queue, and if the queue is full, you can handle the failure gracefully without throwing an exception. | Typically used when you expect that the operation of adding an element to the queue should always succeed, and an exception is appropriate if it does not. |
In the orchestration of Java programming, queues play a pivotal role in managing elements in a structured, first-in-first-out (FIFO) fashion. When dealing with queues of finite capacity, understanding the nuances between the offer()
and add()
methods becomes crucial.
This article delves into a practical example using a LinkedBlockingQueue
to showcase the distinct behaviors of these methods when the queue reaches its limit.
Code Example:
import java.util.concurrent.LinkedBlockingQueue;
public class QueueFullExample {
public static void main(String[] args) {
// Setting a fixed capacity for the queue
int capacity = 5;
LinkedBlockingQueue<String> queue = new LinkedBlockingQueue<>(capacity);
// Filling the queue to its capacity
for (int i = 0; i < capacity; i++) {
queue.offer("Element" + i);
}
// Attempting to add more elements with offer()
boolean offerResult = queue.offer("OverflowElement");
System.out.println("Offer Result: " + offerResult);
try {
// Attempting to add more elements with add()
queue.add("AnotherOverflowElement");
System.out.println("Add successful");
} catch (IllegalStateException e) {
System.out.println("Add failed: " + e.getMessage());
}
}
}
The Java program establishes a LinkedBlockingQueue
with a fixed capacity of 5 elements, laying the foundation for the subsequent actions. The queue is systematically populated with elements Element0
through Element4
using the offer()
method.
Subsequently, the offer()
method is employed to add OverflowElement
to the queue, signaling its success or failure through a boolean result. Transitioning to an alternate approach, the add()
method is introduced with the attempt to add AnotherOverflowElement
to the queue.
It is important to note that this method may trigger an IllegalStateException
if the queue is at its predefined capacity. The code execution concludes with the program’s output, explicitly demonstrating the divergent behaviors of offer()
and add()
when the queue reaches its specified limit.
The output succinctly communicates that offer()
gracefully returns false
upon reaching capacity, while add()
adopts a more assertive stance, prompting an IllegalStateException
with the message Queue full
.
Offer Result: false
Add failed: Queue full
The output demonstrates that offer()
gracefully handles the overflow by returning false
, while add()
throws an IllegalStateException
with the message Queue full
, showcasing the contrasting behaviors of these queue methods when the capacity is reached.
Conclusion
The offer()
and add()
methods in Java’s Queue
interface serve the common purpose of adding elements to a queue, but they exhibit key differences. While both methods attempt to add elements, offer()
gracefully returns false
if the queue is at capacity, providing a non-exceptional approach.
On the other hand, add()
is more assertive and throws an IllegalStateException
if the queue is full, making it suitable for scenarios where successful addition is expected. Understanding these distinctions enables developers to choose the appropriate method based on the desired handling of capacity constraints.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn