How to Implement Concurrent Queue in Java
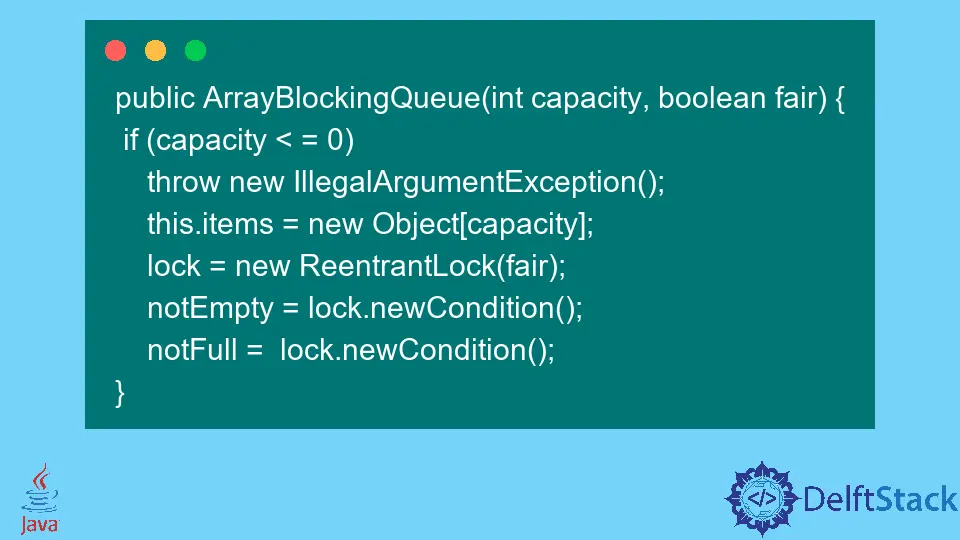
This article will discuss some of the most effective implementations of concurrent queues in Java and which ones need to be utilized.
Concurrent Queues in Java
First, let’s discuss all three queues.
Java ConcurrentLinkedQueue
No locking functionality is built into the ConcurrentLinkedQueue
. As a direct result of this, it provides a wait-free technique in which both add and poll are guaranteed to be thread-safe and to return promptly.
If our client ends up waiting in an eternal loop, regardless of which option we pick, we will need to choose a blocking queue as the best alternative. In addition, the wait-free method may be used without blocking other threads.
CAS or Compare-And-Swap is being utilized rather than locks in this queue. It is an excellent choice for modern reactive systems since these systems do not allow the usage of blocking data structures and would gain a great lot by using this method.
Java ArrayBlockingQueue
Internally, this queue makes use of an array. The result is a bounded queue whose size is predetermined.
The put
and take
operations in the ArrayBlockingQueue
share the same lock. The performance hit is worth the assurance that no entries will be overwritten.
Specifically, the queue pre-allocates the array before it is used. While this might increase throughout, it could also use too much memory.
One such use is a simple task queue. It is common to have a lot of staff to complete a small number of customers in this situation.
Since this queue’s size is capped, it provides a buffer zone in case of memory constraints. One example is the possibility of a long period during which a big capacity queue remains empty.
Fairness policy means the lock implementation will reasonably maintain thread order. If Thread A enters the lock acquisition phase first, and Thread B enters, Thread A will get the lock.
Without justice, the outcome is unclear. It’s likely to be scheduled after the following thread.
Java LinkedBlockingQueue
Each item in the LinkedBlockingQueue
is represented by a new node in the LinkedList variation used by the queue.
LinkedBlockingQueue
’s performance varies significantly from run to run. That is to say; we must constantly profile our circumstances to guarantee we are using the most appropriate data structure.
Best Queue to Implement in Java
Each time an item is added or withdrawn from the queue, LinkedBlockingQueue
must perform an allocation and deallocation of nodes. Therefore, an ArrayBlockingQueue
can be preferable if the queue increases and decreases rapidly.
The size of an ArrayBlockingQueue
is always the same. Adding the eleventh item with a capacity of 10 will cause the insert
statement to stall until an existing thread deletes an item.
When many threads attempt to add and delete items from the queue simultaneously—when the queue is unavailable—this causes a fairness problem. The first thread to request anything is always the first to get it, thanks to a fairness mechanism.
Without this, the wait time for one thread might be much longer than the wait time for another, leading to unexpected behavior. But the burden of managing fairness will reduce throughput.
The choice, though, relies on whether or not you need the blocking. It sounds like a situation where there are plenty of suppliers but just one buyer.
When there are many consumers but only one producer, the blocking behavior may not be necessary. In these cases, the consumers may check whether the queue is empty and proceed if so.
Implementation of ArrayBlockingQueue
in Java
The ArrayBlockingQueue
implementation utilizes the single-lock
double condition technique. Its constructor one has underlying data structures, Array and LinkedList, respectively.
The following is the constructor for the ArrayBlockingQueue
:
public ArrayBlockingQueue(int capacity, boolean fair) {
if (capacity < = 0)
throw new IllegalArgumentException();
this.items = new Object[capacity];
lock = new ReentrantLock(fair);
notEmpty = lock.newCondition();
notFull = lock.newCondition();
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn