Java 中的 FIFO 队列
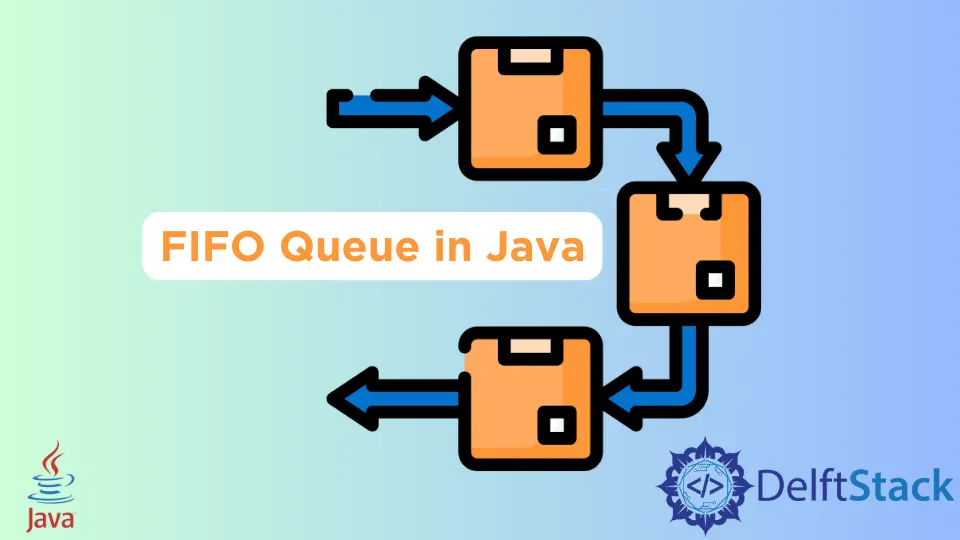
队列是实现先进先出概念的线性数据结构。这个概念意味着先进入的元素最先出来。
代表队列
的技术术语使用前端和后端。元素从后端添加到 Queue
并从前端取出。
这个概念的真实例子是售票柜台排,站在第一个位置的人最先拿到票。现在在 Java 中,概念或数据结构在 Queue
接口中实现。该接口存在于 java.util
包中。
下面是演示队列结构的代码块。
package F10;
import java.util.LinkedList;
import java.util.Queue;
public class Main {
public static void main(String[] args) {
Queue queue = new LinkedList<String>();
queue.add("First");
queue.add("Second");
queue.add("third");
System.out.println("Created Queue: " + queue);
String value = (String) queue.remove();
System.out.println("The element deleted from the head is: " + value);
System.out.println("The queue after deletion: " + queue);
String head = (String) queue.peek();
System.out.println("The head of the present queue is: " + head);
int size = queue.size();
System.out.println("The size of the queue is: " + size);
}
}
有多种方法可以在 Java 中实现 Queue
数据结构。在上面的代码块中,LinkedList
的使用实现了这个概念。
首先,使用 new LinkedList
语句实例化 Queue
引用。add
方法存在于 Queue
接口中,它将指定的元素插入到 Queue
中。附加值时,该函数返回布尔值 true
。
当由于大小限制而无法添加元素时,该方法可能会抛出 IllegalStateException
。当传递的值为空时,它也会抛出 NullPointerException
。当初始元素集被附加到列表中时,队列就会被打印出来。System.out.println
语句采用需要打印的字符串。
接下来,在 Queue
实例上调用 remove
函数。它将删除存在于队列头部的元素,因此返回头部元素。
有一个类似的 poll
函数也可以删除头部的元素。唯一的区别在于 remove
函数在 Queue 为空时抛出 NoSuchElementException
。删除的值存储在变量中并打印。打印剩余的队列以检查剩余的元素。
peek
函数检索队列中最顶层的元素并且不会删除它;它是一种检查队列头部元素的方法。当队列为空时,该函数返回头部值并抛出 NoSuchElementException
。size
函数存在于 Collection
界面中,并返回集合的大小。因此,此方法打印队列中剩余元素的大小。
输出:
Created Queue: [First, Second, third]
The element deleted from the head is: First
The Queue after deletion: [Second, third]
The head of the present Queue is: Second
The size of the Queue is: 2
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn