Comparable vs Comparator in Java
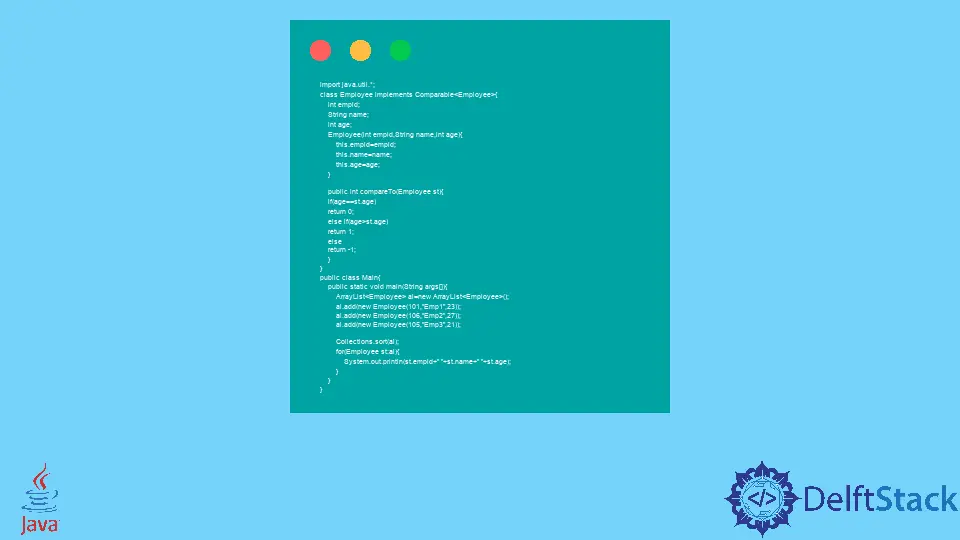
This article will discuss comparable and comparators and find the difference between their definitions and their use cases in Java.
Java Comparable
A Comparable
object in Java is used to compare itself with the other objects. We need to implement the java.lang.Comparable
interface in the class to use it and compare it with the instances. This interface has a single method called compareTo(object)
, which should be in line with the other methods of the object. This function also defines the natural order of the object.
We use Comparable
objects in Java when there is a single default comparison or only one implementation within the same class. This means that we can compare the two objects only based on single data.
We use <
, >
, and =
operators for comparison. These are used to compare the present object with the specified object.
- Positive, if the present object
>
the specified object. - Negative, if the present object
<
the specified object. - Zero, if the current object
=
the specified object.
For Example,
import java.util.*;
class Employee implements Comparable<Employee> {
int empid;
String name;
int age;
Employee(int empid, String name, int age) {
this.empid = empid;
this.name = name;
this.age = age;
}
public int compareTo(Employee st) {
if (age == st.age)
return 0;
else if (age > st.age)
return 1;
else
return -1;
}
}
public class Main {
public static void main(String args[]) {
ArrayList<Employee> al = new ArrayList<Employee>();
al.add(new Employee(101, "Emp1", 23));
al.add(new Employee(106, "Emp2", 27));
al.add(new Employee(105, "Emp3", 21));
Collections.sort(al);
for (Employee st : al) {
System.out.println(st.empid + " " + st.name + " " + st.age);
}
}
}
Output:
105 Emp3 21
101 Emp1 23
106 Emp2 27
Java Comparator
The Comparator
object is used for comparing two different objects either in the same class or two different classes with the help of the implementation of the java.lang.Comparator
interface.
We use comparators
when there is more than one way of comparing the two objects. To use the Comparator interface, the class has to implement the method compare()
. It can be used to compare two objects in a way that might not align with the natural order of the object.
For example,
import java.io.*;
import java.lang.*;
import java.util.*;
class Employee {
int eid;
String name, address;
public Employee(int eid, String name, String address) {
this.eid = eid;
this.name = name;
this.address = address;
}
public String toString() {
return this.eid + " " + this.name + " " + this.address;
}
}
class Sortbyeid implements Comparator<Employee> {
// Used for sorting in ascending order of
// roll number
public int compare(Employee a, Employee b) {
return a.eid - b.eid;
}
}
class Main {
public static void main(String[] args) {
ArrayList<Employee> a = new ArrayList<Employee>();
a.add(new Employee(111, "Emp1", "Delhi"));
a.add(new Employee(131, "Emp2", "Up"));
a.add(new Employee(121, "Emp3", "Jaipur"));
Collections.sort(a, new Sortbyeid());
System.out.println("Sorted: ");
for (int i = 0; i < a.size(); i++) System.out.println(a.get(i));
}
}
Output:
Sorted:
111 Emp1 Delhi
121 Emp3 Jaipur
131 Emp2 Up