How to Override the CompareTo Method in Java
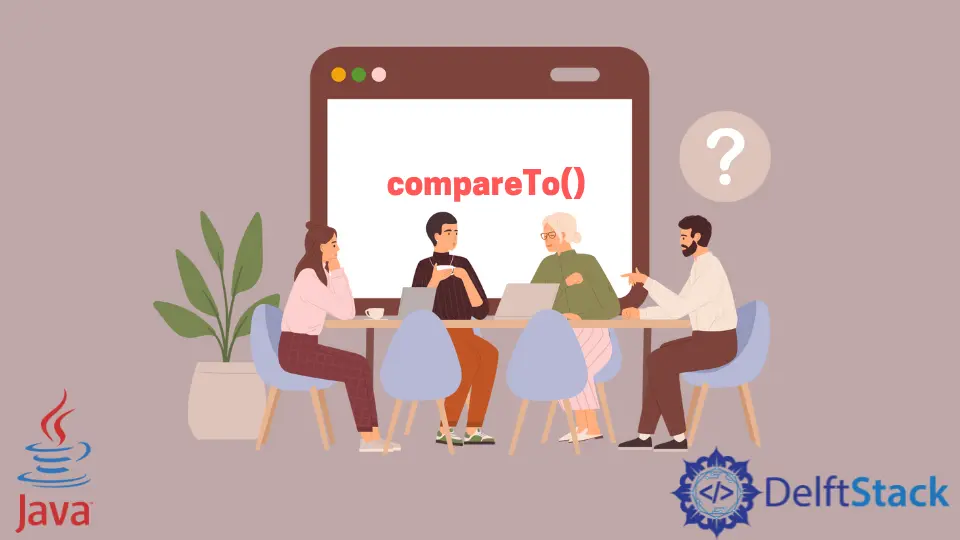
The compareTo()
method belongs to the Comparable
interface in Java. It is used to compare two objects.
It compares this object with the specified object for order. It returns a negative integer if this object is less than the specified object, zero if this object is equal to the specified object, a positive integer if this object is greater than the specified object.
Although, Java provides several other methods to sort values such as:
-
The
Arrays.sort()
method sorts the elements of an array in ascending order. -
The
Collections.sort()
method that works similar to theArrays.sort()
method. We can sort the arrays,queue
,linked list
, and many more using this method. -
The
Comparator interface
is used to sort an array or list of an object based on custom order sort. For example, for a list ofStudent objects
, the natural order may be ordered by students’ roll numbers.Still, in real-life applications, we may want to sort the list of students by their
last name
,first name
,date of birth
, or simply any other such criteria. In such conditions, we use acomparator
interface. -
The
Comparable
interface is used to order the user-defined class objects.The comparable interface provides a single sorting sequence only, i.e., we can only sort the elements based on a single data basis. For example, it may be
age
,roll number
,name
, or anything else.
To summarize, if we want to sort the objects based on the natural order, we use the comparable interface’s compareTo()
method.
Whereas, if we want to sort the object based on the attributes of different objects or customized sorting, we use compare()
of Comparator interface.
Override the compareTo()
Method in Java
To sort or compare objects according to the need of an operation, we first need to implement the Comparable
interface in the class and then override the compareTo()
method.
As we have to sort the array of objects, the traditional array.sort()
method will not work, so we call the Arrays.sort()
method and pass the object array.
It will search whether we have overridden the compareTo()
method or not. Since we have overridden the compareTo()
, objects will be compared using this compareTo()
method, based on age.
package javaexample;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
public class SimpleTesting implements Comparable<SimpleTesting> {
String name;
int age;
// Creating constructor
SimpleTesting(String name, int age) {
this.name = name;
this.age = age;
}
public int getage() {
return age;
}
public String getname() {
return name;
}
public static void main(String[] args) {
// Creating OverrideCompareto class object
SimpleTesting ob[] = new SimpleTesting[4];
// Inserting elements in the objects
ob[0] = new SimpleTesting("Virat", 71);
ob[1] = new SimpleTesting("Rohit", 20);
ob[2] = new SimpleTesting("Dhoni", 11);
ob[3] = new SimpleTesting("Jadeja", 18);
// sorting the array,using overriden method
Arrays.sort(ob);
for (SimpleTesting o : ob) {
// printing the sorted array objects name and age
System.out.println(o.name + " " + o.age);
}
// Creating a dynamic array by using an ArrayList
ArrayList<SimpleTesting> objects = new ArrayList<>();
// creating a new OverrideComparreto object
SimpleTesting newObject1 = new SimpleTesting("Shardul Thakur", 20);
objects.add(newObject1);
// creating a new GFG object
SimpleTesting newObject2 = new SimpleTesting("Jasprit Bumrah", 22);
// inserting the new object into the arraylist
objects.add(newObject2);
// using Collections to sort the arraylist
Collections.sort(objects);
for (SimpleTesting o : objects) {
// printing the sorted objects in arraylist by name and age
System.out.println(o.name + "" + o.age);
}
}
// Overriding compareTo() method
@Override
public int compareTo(SimpleTesting o) {
if (this.age > o.age) {
// if current object is greater --> return 1
return 1;
} else if (this.age < o.age) {
// if current object is greater --> return -1
return -1;
} else {
// if current object is equal to o --> return 0
return 0;
}
}
}
Output:
Dhoni 11
Jadeja 18
Rohit 20
Virat 71
Shardul Thakur20
Jasprit Bumrah22
Let’s see another example of the compareTo()
method to compare the ages of two objects.
package java example;
import java.util.*;
public class SimpleTesting implements Comparable<SimpleTesting> {
// Java Program which shows how to override the compareTo() method of comparable
// interface
// implementing Comparable interface
int age;
// Creating constructor
SimpleTesting(int age) {
this.age = age;
}
public static void main(String[] args) {
// Creating a dynamic array by using an arraylist
List<SimpleTesting> list = new ArrayList<>();
// Inserting elements in the objects
list.add(new SimpleTesting(56));
list.add(new SimpleTesting(66));
list.add(new SimpleTesting(21));
Collections.sort(list);
list.forEach(el -> System.out.println(el.age));
}
// Overriding compareTo() method
@Override
public int compareTo(SimpleTesting user) {
return this.age >= user.age ? -1 : 0;
}
}
Output:
66
56
21
In this tutorial, we saw the different types of techniques used for sorting in Java. We also understood how we could override the compareTo()
method in Java with the help of examples.