How to Reverse an Int Array in Java
-
Basic Java Program to Reverse an
int
Array - Java Program Performing Swap Operation to Reverse an Int Array
- Reverse an Int Array Using Java Collections
-
Reverse an Int Array Using Java
Collections.reverse(ArrayList)
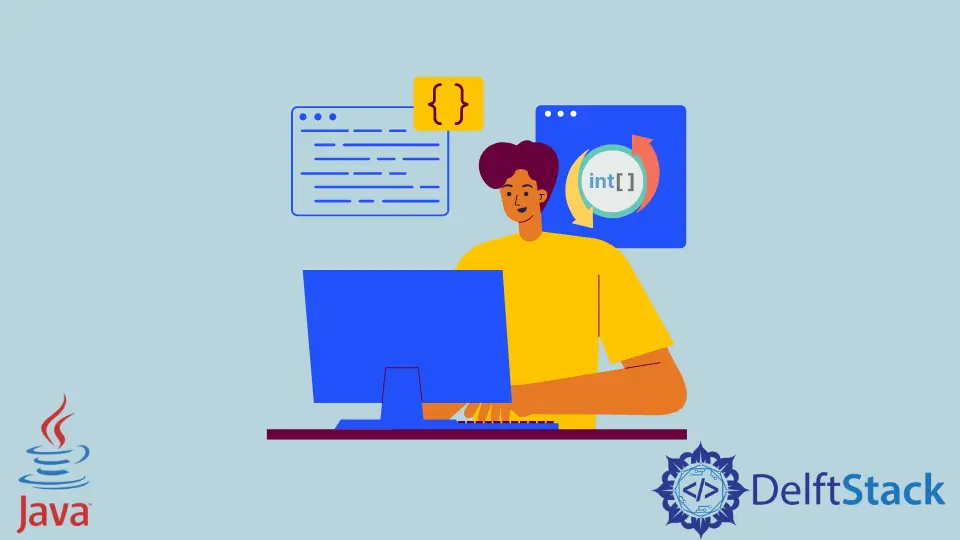
In this tutorial content, we will discuss how to reverse an int
array using Java. These structure of reversing an integer array ill need programming knowledge of Java Loop and Java Array. We can perform the reverse operation using three Java programs. Let us discuss each program implementations through examples.
Basic Java Program to Reverse an int
Array
In this first example, we take the size of array and the elements of array as input. We consider a function reverse
which takes the array (here array) and the size of an array as the parameters. Inside the function, we initialize a new array. The first array is iterated from the first element and each element of array is placed in the new declared array rom the rear position. In this way, we can reversely place the elements of array in the new array. Let us follow the below example.
import java.io.*;
import java.lang.*;
import java.util.*;
public class Example1 {
public static void reverse(int x[], int num) {
int[] y = new int[num];
int p = num;
for (int i = 0; i < num; i++) {
y[p - 1] = x[i];
p = p - 1;
}
System.out.println("The reversed array: \n");
for (int j = 0; j < num; j++) {
System.out.println(y[j]);
}
}
public static void main(String[] args) {
int[] array = {11, 12, 13, 14, 15};
reverse(array, array.length);
}
}
Output:
The reversed array:
15
14
13
12
11
Java Program Performing Swap Operation to Reverse an Int Array
In this second way out, we use the similar code for the including and printing of the array. Here, we swap the elements of the array without creating any new array. The first element of the array is swapped with the last element. The second element is swapped with the second-last element and so on. The below example will explain these in detail.
import java.io.*;
import java.lang.*;
import java.util.*;
public class Example2 {
public static void reverse(int x[], int num) {
int a, b, c;
for (a = 0; a < num / 2; a++) {
c = x[a];
x[a] = x[num - a - 1];
x[num - a - 1] = c;
}
System.out.println("The reversed array: \n");
for (b = 0; b < num; b++) {
System.out.println(x[b]);
}
}
public static void main(String[] args) {
int[] array = {11, 12, 13, 14, 15};
reverse(array, array.length);
}
}
Output:
The reversed array:
15
14
13
12
11
Reverse an Int Array Using Java Collections
The third method is to use the java.util.Collections.reverse(List list)
method. This method reverses the elements of an array in the specified list. First, we convert the array into a list by using java.util.Arraya.asList(array)
and then reverse the list in a specified manner. Let us discuss in the below example.
import java.util.*;
public class Example3 {
public static void reverse(Integer x[]) {
Collections.reverse(Arrays.asList(x));
System.out.println(Arrays.asList(x));
}
public static void main(String[] args) {
Integer[] arr = {15, 25, 35, 45, 55};
reverse(arr);
}
}
Output:
[55, 45, 35, 25, 15]
Reverse an Int Array Using Java Collections.reverse(ArrayList)
In this last example, we will learn reversing an int ArrayList by using Collections.reverse(ArrayList)
method.
import java.util.ArrayList;
import java.util.Collections;
public class Main {
public static void main(String[] args) {
ArrayList arrList = new ArrayList();
arrList.add("20");
arrList.add("30");
arrList.add("40");
arrList.add("50");
arrList.add("60");
System.out.println("Order Before Reverse: " + arrList);
Collections.reverse(arrList);
System.out.println("Order After Reverse: " + arrList);
}
}
Output:
Order Before Reverse: [20, 30, 40, 50, 60]
Order After Reverse: [60, 50, 40, 30, 20]