How to Reverse a String in Java
-
Reverse a String Using the
reverse()
Method in Java - Reverse a String Using Custom Code in Java
-
Reverse a String Using the
append()
Method in Java - Reverse a String Using Recursion in Java
-
Reverse a String Using
Stack
in Java
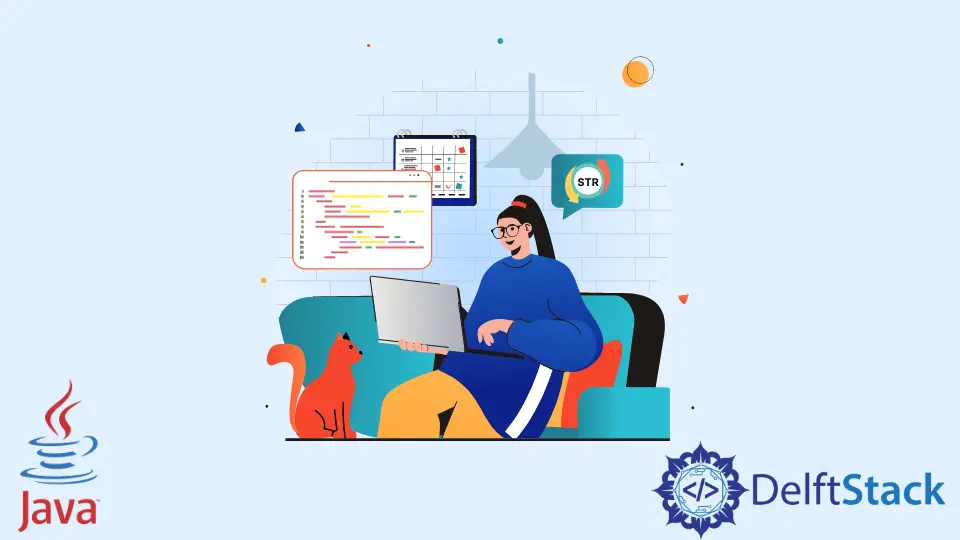
This tutorial introduces how to reverse a string in Java and lists some example codes to understand it.
There are several ways to reverse a string, like reverse()
, sorted()
, and parallelSort()
methods, etc. Let’s see the examples.
Reverse a String Using the reverse()
Method in Java
In this example, we use the reverse()
method of the StringBuilder
class to get the string in reverse order, and we use the toString()
method to get string object from the StringBuilder
object. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelfStack";
System.out.println(str);
StringBuilder strb = new StringBuilder(str);
str = strb.reverse().toString();
System.out.println(str);
}
}
Output:
DelfStack
kcatSfleD
Reverse a String Using Custom Code in Java
If you don’t want to use built-in methods, then use a custom code that will swap the string characters using a loop and collect them into a char array that further converted into a string. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelfStack";
System.out.println(str);
char[] ch = str.toCharArray();
int begin = 0;
int end = ch.length - 1;
char temp;
while (end > begin) {
temp = ch[begin];
ch[begin] = ch[end];
ch[end] = temp;
end--;
begin++;
}
str = new String(ch);
System.out.println(str);
}
}
Output:
DelfStack
kcatSfleD
Reverse a String Using the append()
Method in Java
This is another approach to reverse a string in Java. Here, we use charAt()
and append()
methods inside the for
loop to fetch and store chars into StringBuilder
object. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelfStack";
System.out.println(str);
StringBuilder dest = new StringBuilder();
for (int i = (str.length() - 1); i >= 0; i--) {
dest.append(str.charAt(i));
}
System.out.println(dest);
}
}
Output:
DelfStac
kcatSfleD
Reverse a String Using Recursion in Java
Recursion is a technique in which a function calls itself again and again till the base condition. We can use recursion to reverse the string, as in the below example.
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelfStack";
System.out.println(str);
String reverseStr = reverseIt(str, str.length() - 1);
System.out.println(reverseStr);
}
static String reverseIt(String str, int index) {
if (index == 0) {
return str.charAt(0) + "";
}
char letter = str.charAt(index);
return letter + reverseIt(str, index - 1);
}
}
Output:
DelfStack
kcatSfleD
Reverse a String Using Stack
in Java
We can use the Stack
class to reverse the string. We use the push()
method of Stack
to collect the characters into the stack and then use the pop()
method to fetch the stored characters in reverse order. See the example below.
import java.util.Stack;
public class SimpleTesting {
public static void main(String[] args) {
String str = "DelfStack";
System.out.println(str);
Stack<Character> stack = new Stack<>();
for (int i = 0; i < str.length(); i++) {
stack.push(str.charAt(i));
}
StringBuilder strb = new StringBuilder();
while (!stack.empty()) {
strb.append(stack.pop());
}
System.out.println(strb);
}
}
Output:
DelfStack
kcatSfleD