How to Remove Punctuation From String in Java
-
Remove Punctuation From a String Using the
replaceAll()
Method With Regular Expressions in Java - Remove Punctuation From a String Using ASCII Values in Java
- Remove Punctuation From a String Using a Custom Regular Expression in Java
- Conclusion
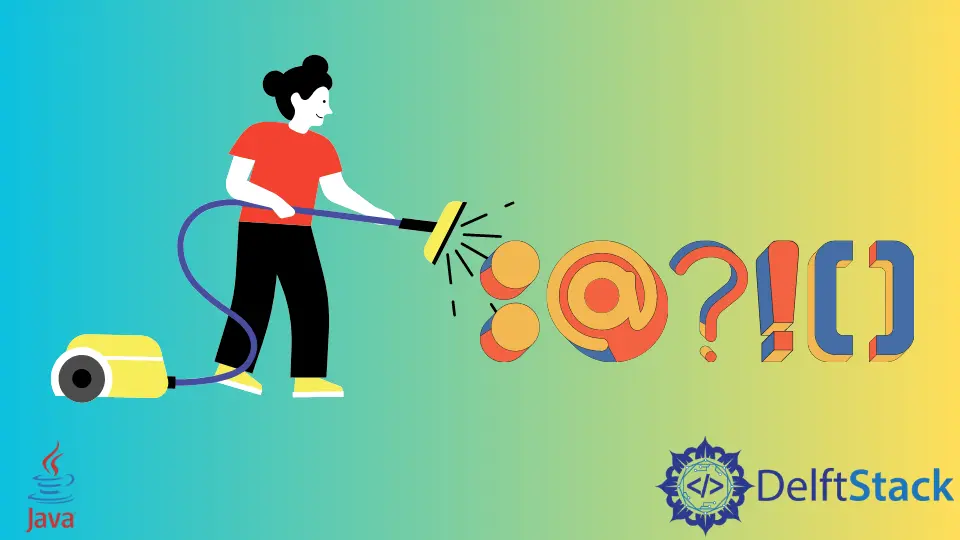
In Java, there are instances where you might want to process text and remove punctuation to extract meaningful information. This simple task is crucial for various applications, from text analysis to natural language processing.
This article will guide you through different methods to remove any punctuation from a string in Java.
Remove Punctuation From a String Using the replaceAll()
Method With Regular Expressions in Java
When working with strings in Java, it’s common to encounter scenarios where the removal of punctuation is necessary for further processing or analysis. The replaceAll
method, coupled with regular expressions, provides an elegant solution for achieving this task.
The replaceAll
method in Java is a part of the String
class and is designed to replace all occurrences of a specified regular expression with a given replacement. The syntax is as follows:
public String replaceAll(String regex, String replacement)
Here, regex
is the regular expression to be replaced, and replacement
is the string that will replace the matched part.
When dealing with punctuation removal, the regular expression \\p{Punct}
is particularly useful. This expression matches any punctuation character.
Now, let’s look at how to use this in practical code.
public class RemovePunctuation {
public static String removePunctuation(String input) {
return input.replaceAll("\\p{Punct}", "");
}
public static void main(String[] args) {
String input = "Hello, world! This is a test.";
String result = removePunctuation(input);
System.out.println("Original: " + input);
System.out.println("Without Punctuation: " + result);
}
}
Output:
Original: Hello, world! This is a test.
Without Punctuation: Hello world This is a test
In the RemovePunctuation
class, the removePunctuation
method takes a string input
as its parameter. The method then utilizes the replaceAll
method on the input string, with the regular expression \\p{Punct}
.
This expression matches any punctuation character, including symbols like commas, periods, exclamation marks, and more.
The replaceAll
method replaces all occurrences of punctuation in the given input string with an empty string, effectively removing them. The resulting string with no punctuation is then returned.
In the main
method, we demonstrate the functionality by initializing a string input
with a sample sentence. We then call the removePunctuation
method, pass the input string, and store the result in the result
variable.
Remove Punctuation From a String Using ASCII Values in Java
An alternative method to remove punctuation from a string involves leveraging ASCII values. This approach provides more granular control over character removal by considering the ASCII values of each character in the string.
The basic idea behind using ASCII values for punctuation removal is to iterate through each character in the string and retain only those that are letters, digits, or whitespace. The Character
class in Java provides methods such as isLetterOrDigit()
and isWhitespace()
that help in making these determinations.
Below is a code example of how to use ASCII values to remove punctuation.
public class RemovePunctuation {
public static String removePunctuation(String input) {
StringBuilder result = new StringBuilder();
for (int i = 0; i < input.length(); i++) {
char currentChar = input.charAt(i);
if (Character.isLetterOrDigit(currentChar) || Character.isWhitespace(currentChar)) {
result.append(currentChar);
}
}
return result.toString();
}
public static void main(String[] args) {
String input = "Hello, world! This is a test.";
String result = removePunctuation(input);
System.out.println("Original: " + input);
System.out.println("Without Punctuation: " + result);
}
}
Output:
Original: Hello, world! This is a test.
Without Punctuation: Hello world This is a test
In the RemovePunctuation
class, the removePunctuation
method is implemented to take a string input
and create a StringBuilder
named result
to store the characters without punctuation. The method then iterates through each character in the input string using a for
loop.
For each character, the code checks whether it is a letter, digit, or whitespace using the Character.isLetterOrDigit()
and Character.isWhitespace()
methods. If the character meets any of these criteria, it is appended to the result
StringBuilder.
After iterating through the entire string, the result
StringBuilder is converted back to a string using the toString()
method, and the modified string is returned.
In the main
method, we demonstrate the functionality by initializing a string input
with a sample sentence. We then call the removePunctuation
method, pass the input string, and store the result in the result
variable.
Remove Punctuation From a String Using a Custom Regular Expression in Java
Another effective method for removing punctuation from a string involves using a custom regular expression. This approach provides the flexibility to define a specific set of rules for punctuation removal, allowing for a more tailored solution.
To use a custom regular expression for removing punctuation, we can employ the replaceAll
method on the input string, similar to the approach with the built-in \p{Punct}
expression. However, with a custom regular expression, we have the freedom to define our own rules for which characters to keep and which to remove.
Here’s a complete working code example:
public class RemovePunctuation {
public static String removePunctuation(String input) {
return input.replaceAll("[\\p{Punct}&&[^']]", "");
}
public static void main(String[] args) {
String input = "Hello, world! Don't forget this test.";
String result = removePunctuation(input);
System.out.println("Original: " + input);
System.out.println("Without Punctuation: " + result);
}
}
Output:
Original: Hello, world! Don't forget this test.
Without Punctuation: Hello world Don't forget this test
In the RemovePunctuation
class, the removePunctuation
method is implemented to take a string input
. The key part of this method is the replaceAll
method, where we use a custom regular expression: [\\p{Punct}&&[^']]
.
Breaking down this regular expression:
\\p{Punct}
matches any punctuation character.[^']
is a negated character class that matches any character except the apostrophe ('
). This is added to the expression to retain apostrophes in the final result.
The replaceAll
method then replaces all occurrences of characters matching this custom regular expression with an empty string, effectively removing them.
In the main
method, we demonstrate the functionality by initializing a string input
with a sample sentence containing an apostrophe. We then call the removePunctuation
method, pass the input string, and store the result in the result
variable.
Using a custom regular expression allows for fine-tuning the punctuation removal process according to specific requirements. This method is particularly useful when you need to preserve certain characters while removing others based on custom rules.
Conclusion
In Java development, efficiently managing strings is essential. Removing punctuation is a common requirement that can significantly improve the accuracy of text processing applications.
In this article, we’ve covered three straightforward methods—regular expressions, ASCII values, and custom regular expressions. Whether you prefer the simplicity of regular expressions, the control of ASCII values, or the customization of a tailored regular expression, Java developers now have practical options to handle punctuation removal according to their specific needs.
With these tools, developers can streamline text processing tasks and create robust applications for handling and analyzing textual data.