How to Iterate Through HashMap in Java
-
Iterate Through
HashMap
UsingentrySet()
Method in Java -
Iterate Through
HashMap
Usingforeach
in Java -
Iterate Through
HashMap
UsingkeySet()
Method in Java -
Iterate Through
HashMap
UsingforEach()
Method in Java -
Iterate Through
HashMap
Usingstream
andforEach()
Method in Java
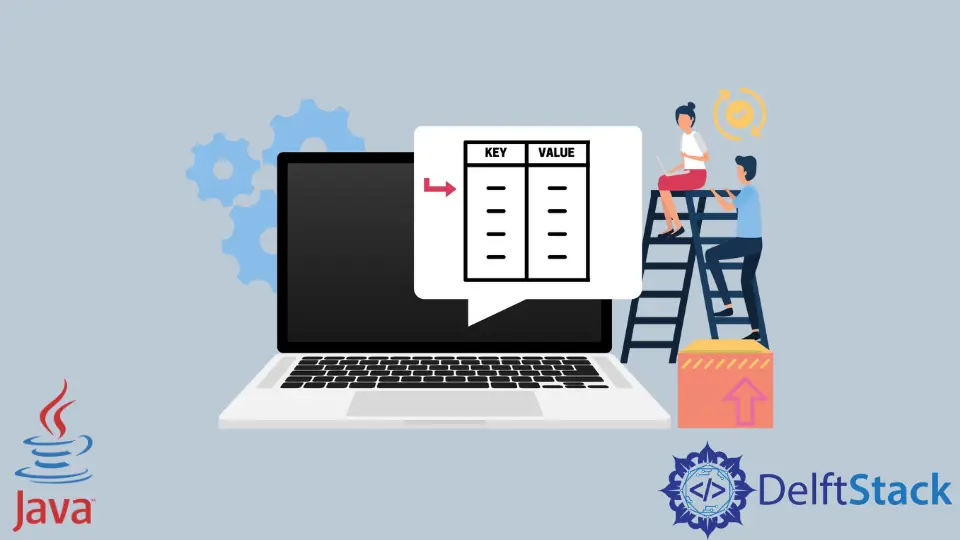
This tutorial introduces how to iterate through HashMap
in Java and lists some example codes to understand it.
There are several ways to iterate HashMap
, here we use keySet()
, entrySet()
, and forEach()
method, etc. Let’s see the examples.
Iterate Through HashMap
Using entrySet()
Method in Java
The entrySet()
method is used to get set a view of the mappings contained in the map. We collect the set view into the iterator and iterate using the while
loop. See the example below.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
Iterator it = map.entrySet().iterator();
while (it.hasNext()) {
Map.Entry<Integer, Integer> entry = (Map.Entry) it.next();
System.out.println(entry.getKey() + " = " + entry.getValue());
}
}
}
Output:
1 = 10
2 = 1020
3 = 300
5 = 500
Iterate Through HashMap
Using foreach
in Java
This is another solution to iterate the HasMap
. Here, we use a for-each
loop to iterate the elements of HashMap
. See the example below.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
for (Map.Entry<Integer, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + " = " + entry.getValue());
}
}
}
Output:
1 = 10
2 = 1020
3 = 300
5 = 500
Iterate Through HashMap
Using keySet()
Method in Java
The keySet()
method is used to collect all the keys and create a set that further could be used to iterate element of the HashMap
. See the example below.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
for (Integer key : map.keySet()) {
System.out.println(key + " = " + map.get(key));
}
}
}
Output:
1 = 10
2 = 1020
3 = 300
5 = 500
Iterate Through HashMap
Using forEach()
Method in Java
We can use the forEach()
method to iterate the elements of HashMap
. The forEach()
method is a new method introduced into Java 8 and available into Iteratable
and Stream
interfaces. See the example below.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
map.forEach((key, value) -> { System.out.println(key + " = " + value); });
}
}
Output:
1 = 10
2 = 1020
3 = 300
5 = 500
Iterate Through HashMap
Using stream
and forEach()
Method in Java
We can use the stream to iterate the elements. Here, we use entrySet()
to collect map elements that further traverse through the forEach()
method of the stream.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
map.entrySet().stream().forEach(System.out::println);
}
}
Output:
1 = 10
2 = 1020
3 = 300
5 = 500