Comment parcourir HashMap en Java
-
Itération par
HashMap
en utilisant la méthodeentrySet()
en Java -
Itération par
HashMap
en utilisantforeach
en Java -
Itération par
HashMap
en utilisant la méthodekeySet()
en Java -
Itération à travers le
HashMap
en utilisant la méthodeforEach()
en Java -
Itération par
HashMap
en utilisant les méthodesstream
etforEach()
en Java
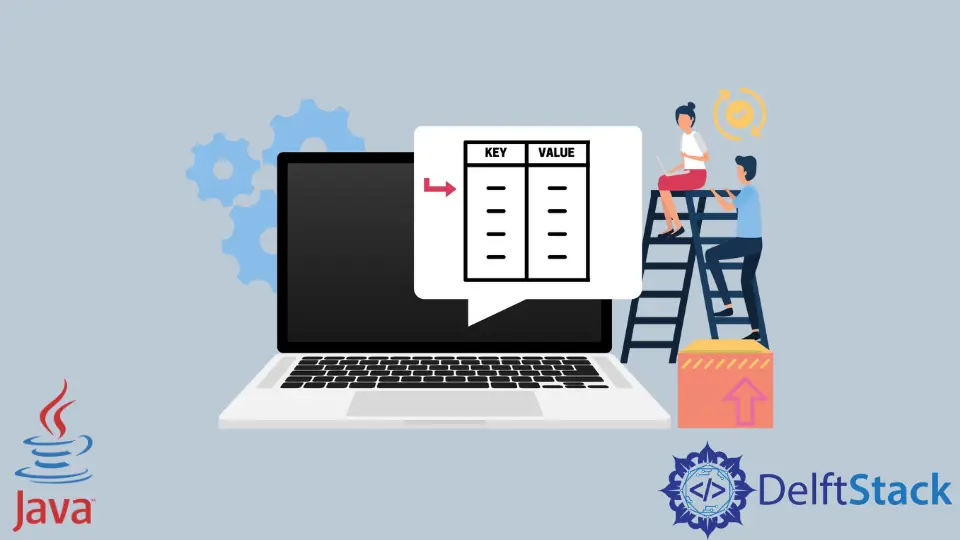
Ce tutoriel explique comment itérer à l’aide de HashaMap
en Java et donne quelques exemples de codes pour le comprendre.
Il y a plusieurs façons d’itérer HashMap
, ici nous utilisons les méthodes keySet()
, entrySet()
, et forEach()
, etc. Voyons les exemples.
Itération par HashMap
en utilisant la méthode entrySet()
en Java
La méthode entrySet()
est utilisée pour obtenir une vue des mappages contenus dans la carte. Nous collectons la vue définie dans l’itérateur et l’itérons en utilisant la boucle while
. Voir l’exemple ci-dessous.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
Iterator it = map.entrySet().iterator();
while (it.hasNext()) {
Map.Entry<Integer, Integer> entry = (Map.Entry) it.next();
System.out.println(entry.getKey() + " = " + entry.getValue());
}
}
}
Production:
1 = 10
2 = 1020
3 = 300
5 = 500
Itération par HashMap
en utilisant foreach
en Java
C’est une autre solution pour itérer le HashaMap
. Ici, nous utilisons une boucle for-each
pour itérer les éléments du HashaMap
. Voir l’exemple ci-dessous.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
for (Map.Entry<Integer, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + " = " + entry.getValue());
}
}
}
Production:
1 = 10
2 = 1020
3 = 300
5 = 500
Itération par HashMap
en utilisant la méthode keySet()
en Java
La méthode keySet()
est utilisée pour collecter toutes les clés et créer un ensemble qui pourrait être utilisé pour itérer des éléments du HashMap
. Voir l’exemple ci-dessous.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
for (Integer key : map.keySet()) {
System.out.println(key + " = " + map.get(key));
}
}
}
Production:
1 = 10
2 = 1020
3 = 300
5 = 500
Itération à travers le HashMap
en utilisant la méthode forEach()
en Java
Nous pouvons utiliser la méthode forEach()
pour itérer les éléments de HashMap
. La méthode forEach()
est une nouvelle méthode introduite dans Java 8 et disponible dans les interfaces Iteratable
et Stream
. Voir l’exemple ci-dessous.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, Integer> map = new HashMap<>();
map.put(2, 1020);
map.put(3, 300);
map.put(1, 100);
map.put(5, 500);
map.forEach((key, value) -> { System.out.println(key + " = " + value); });
}
}
Production:
1 = 10
2 = 1020
3 = 300
5 = 500
Itération par HashMap
en utilisant les méthodes stream
et forEach()
en Java
Nous pouvons utiliser le Stream pour itérer les éléments. Ici, nous utilisons entrySet()
pour collecter les éléments de la carte qui passent ensuite par la méthode forEach()
du Stream.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
map.entrySet().stream().forEach(System.out::println);
}
}
Production:
1 = 10
2 = 1020
3 = 300
5 = 500