How to Get the Current Date-Time in Java
-
LocalDateTime.now()
to Get the Current Date and Time in Java -
ZonedDateTime.now()
to Get the Current Date and Time With Time Zone in Java -
Calendar.getInstance()
to Get the Current Date and Time in Java
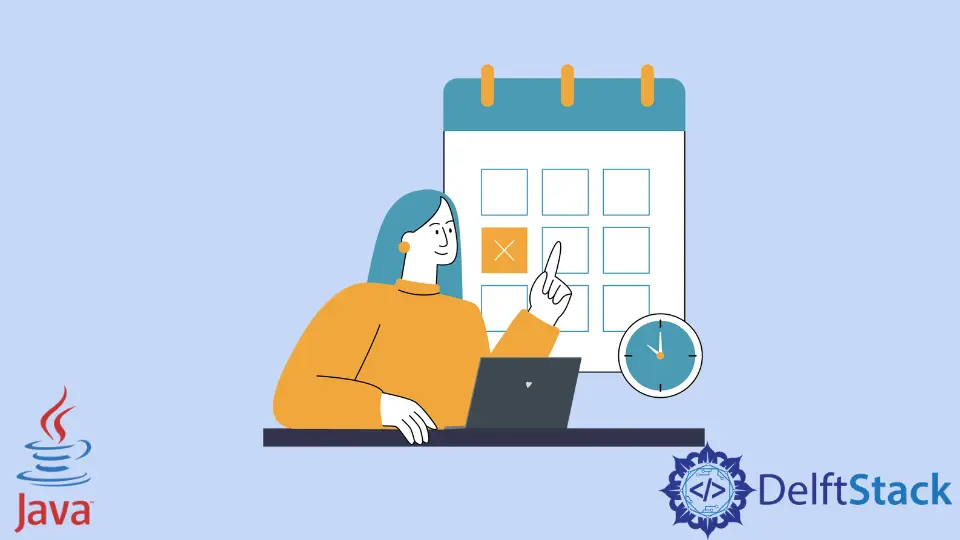
We will introduce all the various methods in Java that can get the current date and time. We will see detailed examples to understand things better.
LocalDateTime.now()
to Get the Current Date and Time in Java
We can get the current date-time using the LocaleDateTime
class with the method now()
. It returns the date and time in YYYY-MM-DD-hh-mm-ss.zzz
format that looks like 2020-09-22T14:39:33.889798
.
To make it more easily readable, we will use DateTimeFormatter.ofPattern(pattern)
that takes a date-time pattern which we can customize according to our needs.
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
DateTimeFormatter dtf = DateTimeFormatter.ofPattern("yyyy/MM/dd HH:mm:ss");
System.out.println("yyyy/MM/dd HH:mm:ss-> " + dtf.format(LocalDateTime.now()));
DateTimeFormatter dtf2 = DateTimeFormatter.ofPattern("yy/MM/dd HH:mm:ss");
System.out.println("yy/MM/dd HH:mm:ss-> " + dtf2.format(LocalDateTime.now()));
DateTimeFormatter dtf3 = DateTimeFormatter.ofPattern("yyyy/MMMM/dd HH:mm:ss");
System.out.println("yyyy/MMMM/dd HH:mm:ss-> " + dtf3.format(LocalDateTime.now()));
DateTimeFormatter dtf4 = DateTimeFormatter.ofPattern("yyyy/MM/dd HH:mm");
System.out.println("yyyy/MM/dd HH:mm-> " + dtf4.format(LocalDateTime.now()));
DateTimeFormatter dtf5 = DateTimeFormatter.ofPattern("yyyy/MM/dd hh:mm");
System.out.println("yyyy/MM/dd hh:mm:ss-> " + dtf5.format(LocalDateTime.now()));
}
}
Output:
yyyy/MM/dd HH:mm:ss-> 2020/09/22 15:07:01
yy/MM/dd HH:mm:ss-> 20/09/22 15:07:01
yyyy/MMMM/dd HH:mm:ss-> 2020/September/22 15:07:01
yyyy/MM/dd HH:mm-> 2020/09/22 15:07
yyyy/MM/dd hh:mm:ss-> 2020/09/22 03:07
ZonedDateTime.now()
to Get the Current Date and Time With Time Zone in Java
Time zone is an important part of date and time. We can get the date-time with the time zone using ZonedDateTime.now()
.
That’s not all, as we can get the time of every timezone by passing the ZoneId
arguments inside ZonedDateTime.now()
.
Check out the below example.
import java.time.ZonedDateTime;
public class Main {
public static void main(String[] args) {
System.out.println(ZonedDateTime.now());
System.out.println("Get current timezone " + ZonedDateTime.now().getZone());
System.out.println(
"Get time of different timezone: " + ZonedDateTime.now(ZoneId.of("America/New_York")));
}
}
Output:
2020-09-22T15:53:32.635585+05:30[Asia/Kolkata]
Get current timezone Asia/Kolkata
Get time of different timezone: 2020-09-22T06:23:32.643391-04:00[America/New_York]
Calendar.getInstance()
to Get the Current Date and Time in Java
Another way to get the current date/time is to use Calendar.getInstance()
returns a Calendar
object with the current date and time that can be converted in Date/Time
formate using the getTime()
method.
We can see the time is shown in the example.
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
String timeStamp =
new SimpleDateFormat("yyyy/MM/dd HH:mm:ss").format(Calendar.getInstance().getTime());
System.out.println(timeStamp);
}
}
Output:
2020/09/22 15:59:45
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn