How to Create a New List in Java
- Create an Empty New List in Java
- Create a Non-Empty New List in Java
- Create a Non-Empty List of Fixed Size in Java
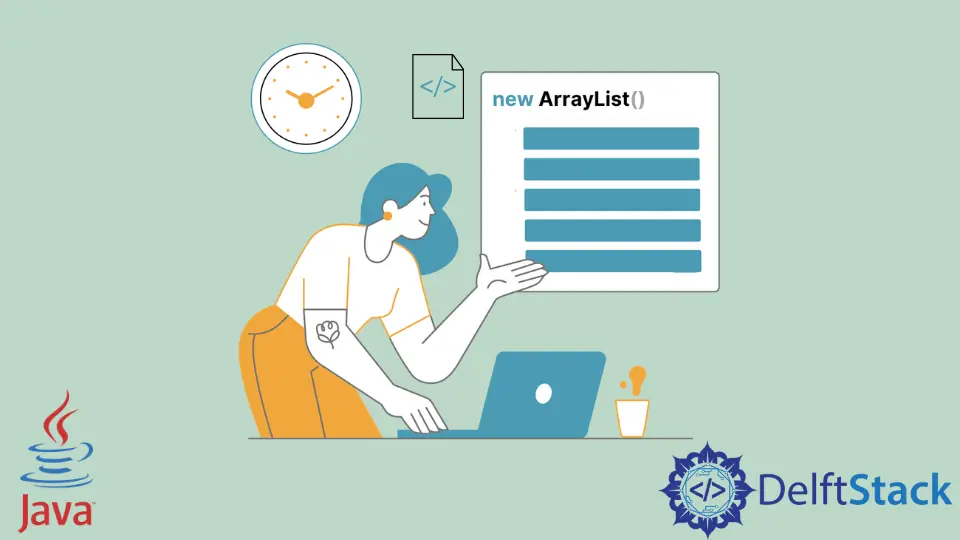
This tutorial will discuss methods to create different types of lists in Java.
List
in Java is an interface and is implemented by ArrayList
, LinkedList
, Vector
and Stack
. It provides an ordered collection of objects. The user has precise control over where in the list each element is inserted. The user can access elements by their integer index (position in the list) and search for elements in the list. Moreover, Lists also allow duplicate elements to be stored.
Create an Empty New List in Java
Since List
is an interface, we can not create a List object directly. However, we can create objects of the classes which implement the List interface: ArrayList
, LinkedList
, Vector
and Stack
. Here is a simple way:
List myArrayList = new ArrayList();
List myLinkedList = new LinkedList();
List myVector = new Vector();
List myStack = new Stack();
These types of Lists do not have a specific data type and can hold any type of objects and values in it. Let us try creating these in Java and adding some values to it.
import java.util.*;
public class myClass {
public static void main(String args[]) {
// ArrayList
List myArrayList = new ArrayList();
myArrayList.add(1);
myArrayList.add(2);
myArrayList.add("three");
System.out.println("ArrayList: " + myArrayList);
// LinkedList
List myLinkedList = new LinkedList();
myLinkedList.add(4);
myLinkedList.add(5);
myLinkedList.add("six");
System.out.println("LinkedList: " + myLinkedList);
// Stack
List myStack = new Stack();
myStack.add(7);
myStack.add(8);
myStack.add("nine");
System.out.println("Stack: " + myStack);
}
}
The above code outputs:
ArrayList: [1, 2, three]
LinkedList: [4, 5, six]
Stack: [7, 8, nine]
We can see that we added int
and String
values to the lists without any error.
It is possible to specify the data type such that only values of that particular type can be stored. Let us try another example.
import java.util.*;
public class myClass {
public static void main(String args[]) {
// ArrayList
List<Integer> myArrayList = new ArrayList<>();
myArrayList.add(1);
myArrayList.add(2);
myArrayList.add("three");
System.out.println("ArrayList: " + myArrayList);
}
}
The above code results in the following error.
> /myClass.java:9: error: incompatible types: String cannot be converted to Integer
> myArrayList.add("three");
It is because while creating the ArrayList
object, we specified the data type to be Integer
; therefore, it will not accept any other data type.
Create a Non-Empty New List in Java
We discussed how to create empty List objects and then add objects to the list. Now let us see another way to create a List with objects in it.
import java.util.*;
public class myClass {
public static void main(String args[]) {
List<String> list = new ArrayList<String>() {
{
add("a");
add("b");
}
};
System.out.println("ArrayList: " + list);
}
}
The above code outputs:
ArrayList: [a, b]
Create a Non-Empty List of Fixed Size in Java
We can also create a non-empty list of fixed size. If so, operations like add
, remove
will not be supported.
import java.util.*;
public class myClass {
public static void main(String args[]) {
List<Integer> list = Arrays.asList(1, 2);
System.out.println("ArrayList: " + list);
}
}
The above code outputs:
ArrayList: [1, 2]
Let us see what happens if we try adding another element to this list.
import java.util.*;
public class myClass {
public static void main(String args[]) {
List<Integer> list = Arrays.asList(1, 2);
list.add(3);
System.out.println("ArrayList: " + list);
}
}
The above code gives the following error since it does not allow operations like add
, remove
etc:
> Exception in thread "main" java.lang.UnsupportedOperationException
> at java.base/java.util.AbstractList.add(AbstractList.java:153)
> at java.base/java.util.AbstractList.add(AbstractList.java:111)
> at myClass.main(myClass.java:6)
However, you can change the value at any given position in this type of list.
import java.util.*;
public class myClass {
public static void main(String args[]) {
List<Integer> list = Arrays.asList(1, 2);
System.out.println("Initial ArrayList: " + list);
list.set(1, 3);
System.out.println("Changed ArrayList: " + list);
}
}
The above code outputs:
Initial ArrayList: [1, 2]
Changed ArrayList: [1, 3]
Now we know how to create different types of Lists in Java.