How to Convert String to Date in Java
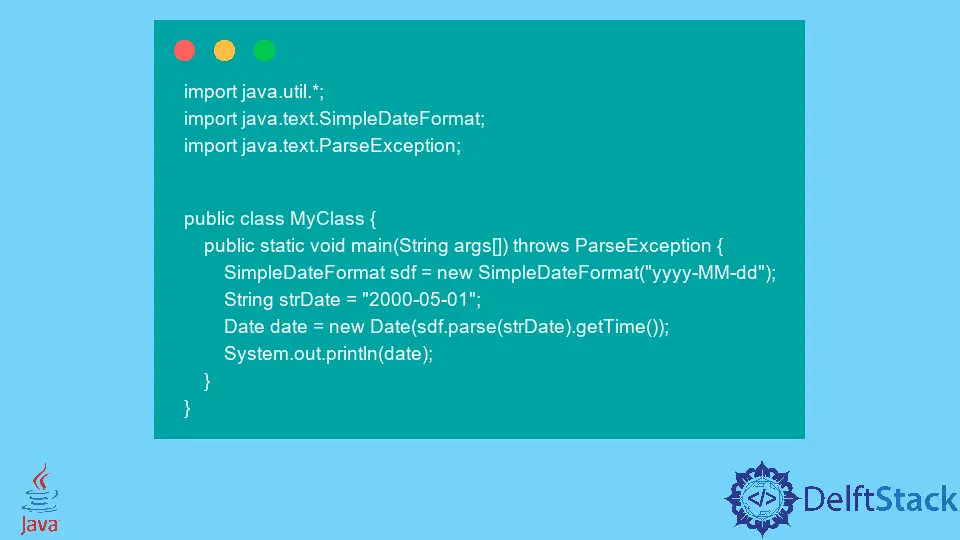
Converting a string to a date in Java is a common requirement that developers often face. Whether you’re parsing user input, reading from a file, or dealing with data from an API, understanding how to manipulate date formats is crucial. Java provides powerful classes like SimpleDateFormat
and LocalDate
that allow for efficient string-to-date conversions.
In this article, we’ll explore various methods to achieve this, complete with code examples and detailed explanations. By the end, you’ll have a solid grasp of how to seamlessly convert strings to dates in Java, enhancing your programming toolkit.
Using SimpleDateFormat
The SimpleDateFormat
class is one of the most traditional ways to convert strings to dates in Java. This class allows you to define a specific date format and parse a string accordingly. Here’s how you can do it:
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class StringToDateExample {
public static void main(String[] args) {
String dateString = "2023-10-15";
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");
Date date = null;
try {
date = formatter.parse(dateString);
} catch (ParseException e) {
e.printStackTrace();
}
System.out.println(date);
}
}
Output:
Sun Oct 15 00:00:00 UTC 2023
In this example, we first import the necessary classes. The dateString
variable holds the date in a string format. We then create an instance of SimpleDateFormat
, specifying the format we expect the string to be in. The parse
method attempts to convert the string into a Date
object. If the string does not match the specified format, a ParseException
is thrown, which we handle using a try-catch block. Finally, we print the resulting date.
Using LocalDate
In Java 8 and later, the LocalDate
class from the java.time
package is a more modern approach to date handling. It offers a more intuitive API and is immutable, making it thread-safe. Here’s how you can convert a string to a LocalDate
:
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class StringToLocalDateExample {
public static void main(String[] args) {
String dateString = "2023-10-15";
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
LocalDate localDate = LocalDate.parse(dateString, formatter);
System.out.println(localDate);
}
}
Output:
2023-10-15
In this code snippet, we use DateTimeFormatter
to define the expected date format. The LocalDate.parse
method takes the string and the formatter as arguments, converting the string into a LocalDate
object. This approach is cleaner and less error-prone than the older SimpleDateFormat
. The output is straightforward, displaying the date in the ISO-8601 format.
Conclusion
Converting strings to dates in Java is essential for effective date manipulation. Whether you choose to use SimpleDateFormat
for legacy applications or LocalDate
for modern projects, both methods provide reliable ways to achieve your goals. Understanding these techniques not only enhances your Java programming skills but also prepares you for handling various date formats in real-world applications. With the examples provided, you should feel confident in implementing these methods in your own projects.
FAQ
-
What is the difference between SimpleDateFormat and LocalDate?
SimpleDateFormat is part of the older Java date-time API, while LocalDate is part of the newer java.time package introduced in Java 8, which is more modern and thread-safe. -
Can I parse dates in different formats using SimpleDateFormat?
Yes, you can specify different patterns in SimpleDateFormat to parse various date formats as needed. -
What happens if the string does not match the expected date format?
If the string does not match the format specified, a ParseException will be thrown, indicating that the parsing failed. -
Is LocalDate mutable?
No, LocalDate is immutable, meaning its state cannot be changed after it is created, making it safer to use in concurrent applications.
- Can I format dates back to strings in Java?
Yes, both SimpleDateFormat and DateTimeFormatter provide methods to convert Date and LocalDate objects back to string representations.