How to Fix Exception in Thread AWT-EventQueue-0 java.lang.NullPointerException
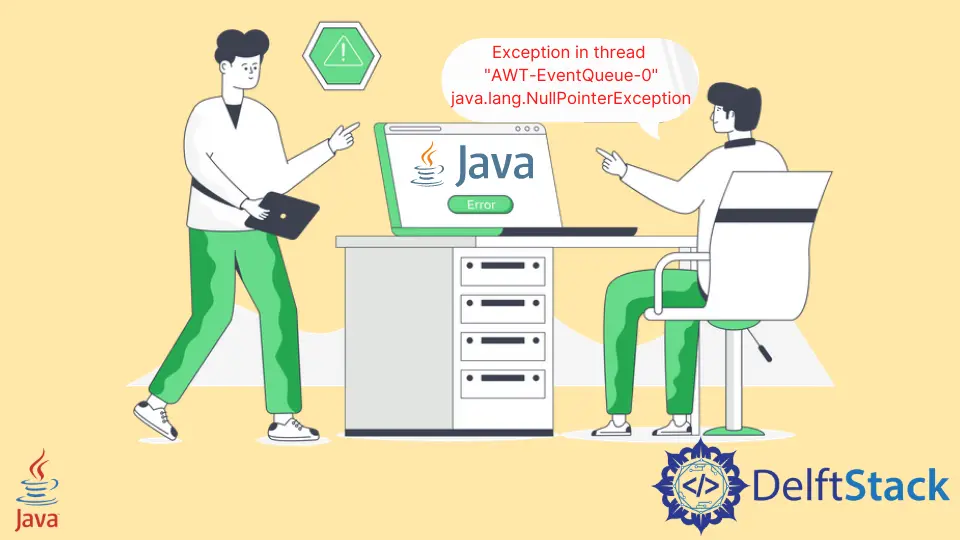
The "AWT-EventQueue-0" java.lang.NullPointerException
exception occurs when we work with Java AWT package methods, and a null
value is passed to any method. This tutorial demonstrates how to solve this NullPointerException
in Java.
Exception in thread "AWT-EventQueue-0" java.lang.NullPointerException
in Java
The "AWT-EventQueue-0" java.lang.NullPointerException
occurs when we pass a null value to the AWT package. The NullPointerException
exception is the most common in Java.
The NullPointerException
occurs when any of the following conditions meet.
- When accessing and modifying the
null
object field. - When we invoke a method from a
null
object. - When accessing and modifying the slots of the
null
object. - When taking the length of any
null
array. - When we try to synchronize over a
null
object. - When we are throwing a
null
value.
Let’s try an example that will throw the "AWT-EventQueue-0" java.lang.NullPointerException
in Java.
package delftstack;
import java.awt.*;
import java.awt.event.*;
import java.util.Timer;
import javax.swing.*;
@SuppressWarnings("serial")
public class Example extends JFrame implements ActionListener, KeyListener {
static Dimension Screen_Size = new Dimension(Toolkit.getDefaultToolkit().getScreenSize());
Insets Scan_Max = Toolkit.getDefaultToolkit().getScreenInsets(getGraphicsConfiguration());
int Task_Bar_Size = Scan_Max.bottom;
static JFrame Start_Screen = new JFrame("Start Screen");
static JFrame Game_Frame = new JFrame("Begin the Game!");
static JLabel Cow_Label = new JLabel();
static int Sky_Int = 1;
static JLabel Sky_Label = new JLabel();
static int SECONDS = 1;
static boolean IS_Pressed = false;
public static void main(String[] args) {
new Example();
}
public Example() {
JPanel Buttons_Panel = new JPanel();
Buttons_Panel.setLayout(null);
Start_Screen.setSize(new Dimension(
Screen_Size.width - getWidth(), Screen_Size.height - Task_Bar_Size - getHeight()));
Start_Screen.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Start_Screen.setVisible(true);
System.out.println(Start_Screen.getSize());
// buttons
JButton Start_Button = new JButton("Start");
Start_Button.addActionListener(this);
Start_Button.setSize(
(int) Start_Screen.getWidth() / 7, (int) (Start_Screen.getHeight() / 15.36));
Start_Button.setBounds((Start_Screen.getWidth() / 2) - Start_Button.getWidth() / 2,
((int) Start_Screen.getHeight() / 2) - Start_Button.getHeight(), Start_Button.getWidth(),
Start_Button.getHeight());
Start_Button.setActionCommand("Start");
Buttons_Panel.add(Start_Button);
Start_Screen.add(Buttons_Panel);
}
@Override
public void actionPerformed(ActionEvent Action_Event) {
Object CMD_Object = Action_Event.getActionCommand();
if (CMD_Object == "Start") {
Start_Screen.setVisible(false);
// getClass().getResource("/cow.png") and getClass().getResource("/grass.png") is giving null
// because there is no image in folder named cow.png or grass.png
ImageIcon Cow_Image = new ImageIcon(getClass().getResource("/cow.png"));
ImageIcon Grass_Image = new ImageIcon(getClass().getResource("/grass.png"));
Game_Frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Game_Frame.setSize(Start_Screen.getSize());
Game_Frame.setVisible(true);
JPanel Demo_Panel = new JPanel();
Demo_Panel.setBackground(Color.white);
Demo_Panel.setLayout(null);
Demo_Panel.setFocusable(true);
Game_Frame.add(Demo_Panel);
Demo_Panel.addKeyListener(this);
Cow_Label.setBounds(
(Start_Screen.getWidth() / 2) - 105, (Start_Screen.getHeight() / 2) - 55, 210, 111);
Cow_Label.setIcon(Cow_Image);
Demo_Panel.add(Cow_Label);
Demo_Panel.setVisible(true);
Cow_Label.setVisible(true);
JLabel Grass_Label = new JLabel();
System.out.println("grass");
// getClass().getResource("/Sky.png") will throw a nullpointerexception because there is no
// image in the folder
ImageIcon Sky1 = new ImageIcon(getClass().getResource("/Sky.png"));
Sky_Label.setIcon(Sky1);
Grass_Label.setIcon(Grass_Image);
Grass_Label.setBounds(0, (Start_Screen.getHeight() - 308), Start_Screen.getWidth(), 350);
System.out.println("mOooow");
Demo_Panel.add(Grass_Label);
Sky_Label.setBounds(1, 56, 1366, 364);
Demo_Panel.add(Sky_Label);
System.out.println("google");
}
}
@Override
public void keyPressed(KeyEvent Key_Event) {
int CMD_Int = Key_Event.getKeyCode();
// getClass().getResource("/cow moving.gif") will throw a nullpointerexception because there is
// no image in the folder
ImageIcon Moving_Cow = new ImageIcon(getClass().getResource("/cow moving.gif"));
System.out.println(CMD_Int);
IS_Pressed = true;
if (CMD_Int == 39) {
System.out.println("Key is Pressed");
Cow_Label.setIcon(Moving_Cow);
} else if (CMD_Int == 37) {
}
System.out.println("End");
while (IS_Pressed == true) {
Timer Wait_Please = new Timer("Wait Please");
try {
Wait_Please.wait(1000);
} catch (InterruptedException p) {
}
int SKY = 1;
SKY += 1;
String SKY_String = "/Sky" + String.valueOf(SKY) + ".png";
ImageIcon SKy = new ImageIcon(getClass().getResource(SKY_String));
Sky_Label.setIcon(SKy);
if (IS_Pressed == false) {
Wait_Please.cancel();
break;
}
}
}
@Override
public void keyReleased(KeyEvent Key_Event) {
// getClass().getResource("/cow.png") and getClass().getResource("/grass.png") is giving null
// because there is no image in folder named cow.png or grass.png
ImageIcon Cow_Image = new ImageIcon(getClass().getResource("/cow.png"));
int CMD_Int = Key_Event.getKeyCode();
IS_Pressed = false;
if (CMD_Int == 39) {
Cow_Label.setIcon(Cow_Image);
} else if (CMD_Int == 37) {
Cow_Label.setIcon(Cow_Image);
}
}
@Override
public void keyTyped(KeyEvent c) {
// TODO Auto-generated method stub
}
}
The code above is about a simple game with a cow standing, and the cow will start moving on pressing the button. It will throw the "AWT-EventQueue-0" java.lang.NullPointerException
because the AWT method new ImageIcon(getClass().getResource())
is getting a null
entry.
The output for this code is:
Exception in thread "AWT-EventQueue-0" java.lang.NullPointerException: Cannot invoke "java.net.URL.toExternalForm()" because "location" is null
at java.desktop/javax.swing.ImageIcon.<init>(ImageIcon.java:234)
at delftstack.Example.actionPerformed(Example.java:48)
at java.desktop/javax.swing.AbstractButton.fireActionPerformed(AbstractButton.java:1972)
at java.desktop/javax.swing.AbstractButton$Handler.actionPerformed(AbstractButton.java:2313)
...
We can solve this issue by moving the images to the class folder path. We can also remove the /
as Windows use \\
for path in Java.
And if it is still not working, we can give the full path to the images. Further explanations are commented-out in the code above.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook