The Dot (.) Operator in Java
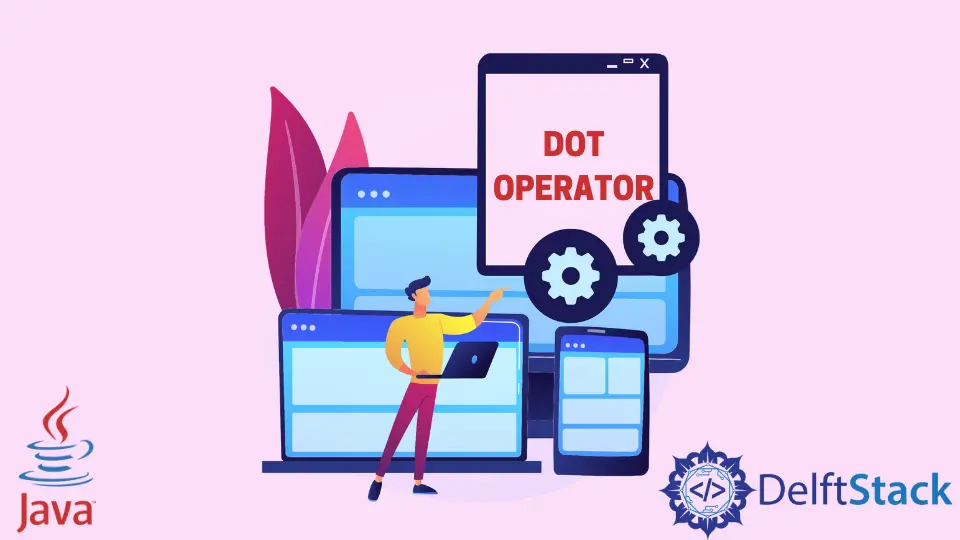
In Java language, the dot operator (.
) symbolizes the element or operator that works over the syntax. It is often known as a separator, dot, and period.
Simply, the dot operator acts as an access provider for objects and classes. The usage of the above operator is as below.
Introduction to the Dot (.
) Operator in Java
In Java, the dot operator (.
) serves as a fundamental syntactic element with a key role in accessing and manipulating class members. It is used to navigate through the structure of classes, objects, and their associated fields and methods.
The dot operator essentially signifies a connection between an object or class and its members, allowing for the retrieval of values from fields, invocation of methods, access to static members, and more. Understanding the usage of the dot operator is foundational for effective object-oriented programming in Java.
Ways to Use the Dot Operator in Java
The dot (.
) operator in Java serves multiple purposes, primarily facilitating the access of class members such as fields and methods.
In this article, we will explore the various ways the dot operator can be employed, using a comprehensive code example to illustrate its applications.
Concept | Description |
---|---|
Accessing Fields | The dot operator is utilized to retrieve values stored in fields within a class. |
Invoking Methods | Crucial for invoking methods on objects, the dot operator connects the object and the method call. |
Accessing Static Members | Employed to access static members, such as constants, directly connecting to class-level elements. |
Chaining Method Calls | Facilitates method chaining, enabling consecutive method calls on the same object using the dot operator. |
Accessing Members of Objects in Collections | Utilized to access elements within collections, connecting to objects stored in the collection. |
Accessing Members of Nested Classes | Necessary for accessing members of nested classes, connecting to the inner class using the dot operator. |
Separating Class and Package | Aids in organizing code by separating classes and packages. Importing involves using the dot operator to indicate the package structure. |
Separating Method and Class | The dot operator is used to separate the method from the class or object when invoking methods. |
Separating Variable and Reference Variable | When accessing fields of an object, the dot operator distinguishes the variable (reference variable) from the field. |
In the vast landscape of Java programming, mastering the dot operator is akin to unlocking the doors to an array of programming possibilities. This unassuming dot (.
) is a powerful tool that allows developers to access fields, invoke methods, and navigate complex class hierarchies.
Code Example:
public class DotOperatorExample {
// Static class with a field
static class MyClass { int myField = 10; }
// Static class with static members
static class Constants { static final double PI = 3.14159; }
// Static class with a method
static class MathOperations {
int add(int a, int b) {
return a + b;
}
}
// Static class for chaining method calls
static class StringManipulator {
String convertToUpperCase(String input) {
return input.toUpperCase();
}
}
// Static class for nested classes
static class Outer {
static class Inner {
void innerMethod() {
System.out.println("Inner method called");
}
}
}
public static void main(String[] args) {
// Accessing fields
DotOperatorExample.MyClass myObject = new DotOperatorExample.MyClass();
int fieldValue = myObject.myField;
System.out.println("Accessing Fields: " + fieldValue);
// Accessing static members
double piValue = DotOperatorExample.Constants.PI;
System.out.println("Accessing Static Members: " + piValue);
// Invoking methods
DotOperatorExample.MathOperations mathObject = new DotOperatorExample.MathOperations();
int sum = mathObject.add(5, 3);
System.out.println("Invoking Methods: " + sum);
// Chaining method calls
String originalString = "Hello, World!";
String resultString =
new DotOperatorExample.StringManipulator().convertToUpperCase(originalString);
System.out.println("Chaining Method Calls: " + resultString);
// Accessing members of nested classes
DotOperatorExample.Outer.Inner innerObj = new DotOperatorExample.Outer.Inner();
innerObj.innerMethod();
}
}
In the initial segment of our code, the dot operator takes center stage in the process of accessing fields. To exemplify this, we instantiate the static inner class MyClass
using the dot operator (DotOperatorExample.MyClass myObject = new DotOperatorExample.MyClass();
).
Subsequently, we employ the dot operator once more to access the field myField
: int fieldValue = myObject.myField;
. This dual application underscores how the dot operator seamlessly facilitates the retrieval of specific attributes within a class.
Transitioning to the realm of static members, we showcase the dot operator’s innate ability to provide direct access to these elements.
In the case of the Constants
class, the dot operator comes into play as we access the static constant PI
directly: double piValue = DotOperatorExample.Constants.PI;
. This demonstration underscores the dot operator’s pivotal role in accessing static elements within a class, offering a straightforward mechanism for such interactions.
The subsequent illustration delves into the dot operator’s significance in method invocation.
This is depicted by creating an instance of the MathOperations
class and subsequently utilizing the dot operator to call the add
method: int sum = mathObject.add(5, 3);
. Here, the dot operator serves as a bridge, seamlessly connecting the object and the method, thereby allowing for the invocation of methods in a smooth and coherent manner.
A notable aspect of the dot operator is its contribution to chaining method calls, enhancing code readability and conciseness.
In this context, we explore the elegance of method chaining by creating a new instance of StringManipulator
and immediately invoking the convertToUpperCase
method in a single line using the dot operator (new DotOperatorExample.StringManipulator().convertToUpperCase(originalString)
). This showcases the dot operator’s ability to streamline method calls, resulting in more readable and efficient code.
Finally, the dot operator’s prowess in navigating through nested classes is elucidated.
Within this section, we instantiate the outer class Outer
and subsequently employ the dot operator to access the nested class Inner
: DotOperatorExample.Outer.Inner innerObj = new DotOperatorExample.Outer.Inner();
. Following this, we invoke the method innerMethod()
using the dot operator (innerObj.innerMethod();
), exemplifying its utility in seamlessly navigating complex class structures.
Output:
In summary, this detailed exploration underscores the multifaceted role of the dot operator in Java programming, showcasing its efficacy in accessing fields, invoking methods, navigating static members, facilitating method chaining, and effortlessly traversing through nested classes. As we run the program, the output will affirm the effectiveness of the dot operator in orchestrating these diverse operations within the Java programming paradigm.
Conclusion
The dot operator in Java serves as a fundamental tool, facilitating the access of fields, invocation of methods, handling static members, chaining method calls, navigating nested classes, and distinguishing class, method, and variable references, contributing to the efficiency and organization of Java programming.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn