What is the /= Operator in Java
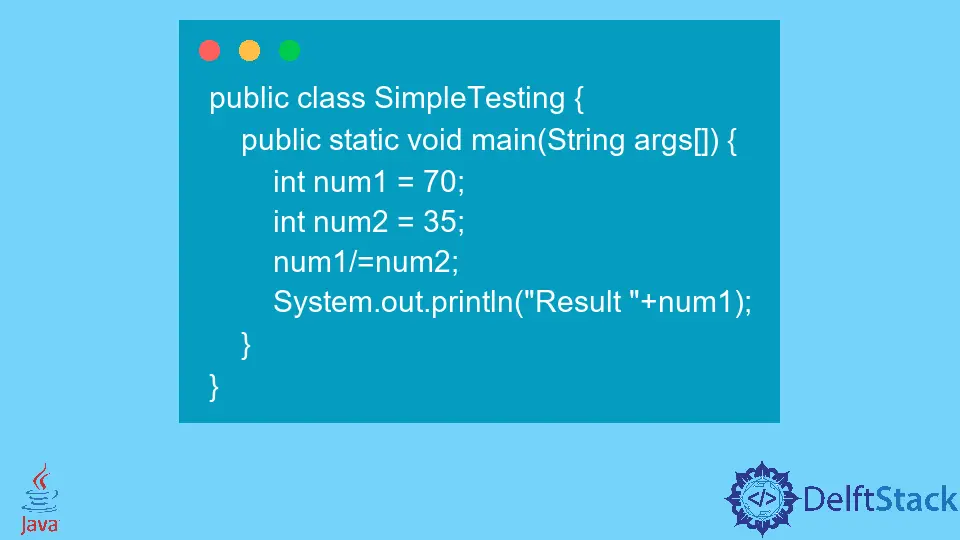
This tutorial introduces the /=
operator and its uses in Java.
Java provides us with several operators to manipulate data when required. It has arithmetic operators, relational operators, assignment operators, etc. Apart from these operators, Java supports combined operators such as +=
, -=
, *=
, /=
, etc.
This tutorial will look at what the /=
operator means. The /
stands for the division operator, and the =
stands for the assignment operator. Java provides a more concise way of using both these operators in a single statement.
The /=
operator is special to Java and will be used separately. Let’s understand with some examples.
Shorthand Divide and Assign /=
Operator in Java
This operator is a combination of division and assignment operators. It works by dividing the current value of the left-hand variable by the right-hand value and then assigning the result to the left-hand operand.
In other words, writing code would be like:
a /= b
It is equivalent to the below code.
a = a / b
Let’s take a code example to understand this concept better.
public class SimpleTesting {
public static void main(String args[]) {
int num1 = 70;
int num2 = 35;
num1 /= num2;
System.out.println("Result " + num1);
}
}
Output:
Result 2
In the above code, num1
is divided by num2
, and the result is stored in num1
.
This code is similar to the below code if we use a simplified operator. Both produce the same result. See the example below.
public class SimpleTesting {
public static void main(String args[]) {
int num1 = 70;
int num2 = 35;
num1 = num1 / num2;
System.out.println("Result " + num1);
}
}
Output:
Result 2
We can use it at any place, even with complex code statements. Let’s take another code example. Here, we used it with ternary operators.
public class SimpleTesting {
public static void main(String args[]) {
int num1 = 70;
int num2 = 35;
int result = ((num1 /= num2) >= 0 ? num1 : num1 + num2);
System.out.println("Result " + result);
}
}
Output:
Result 2
Suppose we have an array and want to divide each element by 2, then we can do this by using the shorthand operator with concise code. See the example below.
public class SimpleTesting {
public static void main(String args[]) {
int[] arr = {23, 32, 65, -54, 82};
for (int i = 0; i < arr.length; i++) {
arr[i] /= 2;
}
// print the array
for (int ele : arr) {
System.out.println(ele);
}
}
}
Output:
11
16
32
-27
41
Using Shorthand Operators in Java
Java supports compound assignment operators such as +=
, -=
, *=
, etc.
In this example, we used other shorthand operators to understand the use of these operators well. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int val = 120;
System.out.println("val = " + val);
val += 10; // addition
System.out.println("val = " + val);
val -= 10; // subtraction
System.out.println("val = " + val);
val *= 10; // multiplication
System.out.println("val = " + val);
val /= 10; // division
System.out.println("val = " + val);
val %= 10; // compound operator
System.out.println("val = " + val);
}
}
Output:
val = 120
val = 130
val = 120
val = 1200
val = 120
val = 0
Conclusion
This article taught us what Java’s /=
operator does. The /=
is a shorthand operator in Java. It enables us to combine the division and assignment operator in a clearer and concise format.