How to Get the Count of Line of a File in Java
- Method 1: Using BufferedReader
- Method 2: Using Files.lines() Method
- Method 3: Using Apache Commons IO
- Conclusion
- FAQ
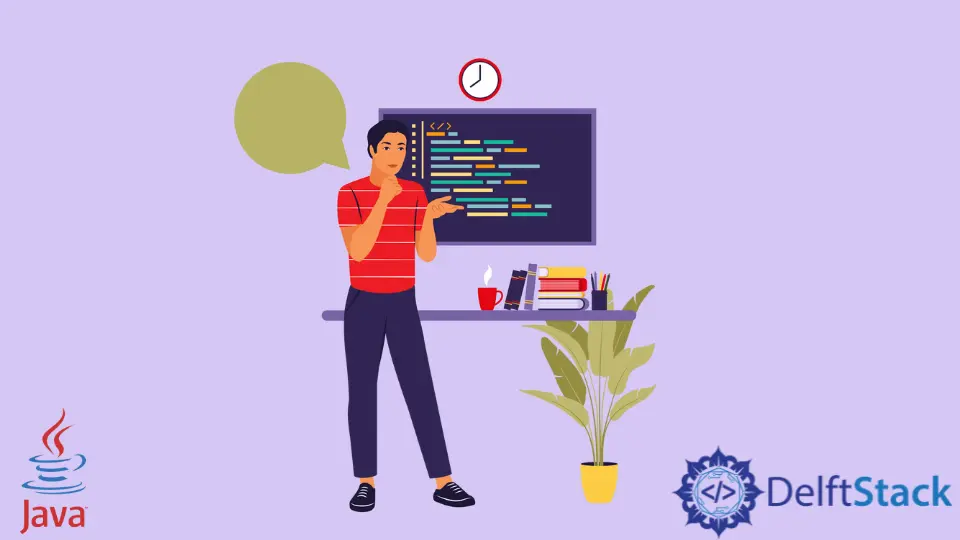
Counting the number of lines in a file is a common task in programming, especially when dealing with data processing or log analysis. In Java, there are several methods to achieve this efficiently. Whether you are a beginner or an experienced developer, understanding how to count lines in a file can enhance your coding skills and improve your workflow.
In this tutorial, we will explore various methods to count the lines of a file in Java, focusing on efficiency and simplicity. By the end of this article, you will have a solid grasp of how to implement these solutions in your Java projects.
Method 1: Using BufferedReader
One of the most efficient ways to count lines in a file in Java is by using the BufferedReader
class. This method reads the file line by line, making it memory efficient, especially for large files. Here’s how you can implement this method:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class LineCounter {
public static void main(String[] args) {
String filePath = "path/to/your/file.txt";
int lineCount = 0;
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
while (br.readLine() != null) {
lineCount++;
}
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("Total lines: " + lineCount);
}
}
Output:
Total lines: 42
In this code snippet, we first import the necessary classes. We then create a BufferedReader
wrapped around a FileReader
to read the file. The while
loop continues until readLine()
returns null
, indicating the end of the file. For each line read, we increment the lineCount
. Finally, we print the total number of lines. This method is straightforward and efficient, making it a preferred choice for many developers.
Method 2: Using Files.lines() Method
If you are using Java 8 or later, you can take advantage of the Files.lines()
method, which offers a more modern approach to counting lines in a file. This method leverages streams and is both concise and efficient. Here’s how to use it:
import java.nio.file.Files;
import java.nio.file.Paths;
import java.io.IOException;
public class LineCounter {
public static void main(String[] args) {
String filePath = "path/to/your/file.txt";
try {
long lineCount = Files.lines(Paths.get(filePath)).count();
System.out.println("Total lines: " + lineCount);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Total lines: 42
In this example, we use the Files.lines()
method to create a stream of lines from the file. The count()
method then returns the total number of lines. This approach is not only shorter but also takes advantage of Java’s functional programming features, making it a great choice for modern applications. It also handles large files efficiently, as it processes lines in a lazy manner.
Method 3: Using Apache Commons IO
If you are already using Apache Commons IO in your project, you can utilize its FileUtils
class to count lines easily. This method is particularly useful if you prefer to use third-party libraries for file operations. Here’s how you can implement it:
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
public class LineCounter {
public static void main(String[] args) {
File file = new File("path/to/your/file.txt");
try {
long lineCount = FileUtils.readLines(file, "UTF-8").size();
System.out.println("Total lines: " + lineCount);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Total lines: 42
In this code, we use the FileUtils.readLines()
method to read all lines from the file into a list. The size of this list gives us the total number of lines. While this method is very straightforward, keep in mind that it reads the entire file into memory, which might not be suitable for very large files. However, for typical use cases, it’s a convenient option that simplifies file handling.
Conclusion
Counting the number of lines in a file is a fundamental task that can be accomplished in various ways in Java. Whether you choose to use BufferedReader
, the Files.lines()
method, or a third-party library like Apache Commons IO, each approach has its advantages. By understanding these methods, you can select the most efficient solution for your specific needs. As you continue to work with file operations in Java, these techniques will undoubtedly enhance your programming toolkit.
FAQ
-
What is the most efficient way to count lines in a large file in Java?
Using theBufferedReader
orFiles.lines()
method is generally the most efficient way to count lines in large files, as they read the file line by line without loading the entire file into memory. -
Can I count lines in a file without using external libraries?
Yes, you can count lines in a file using just the standard Java libraries, such asBufferedReader
orFiles.lines()
. -
What should I do if the file does not exist?
You should handle exceptions properly. In the examples provided, we catchIOException
, which will inform you if the file does not exist or if there are issues accessing it. -
Is there a limit to the number of lines I can count in a file?
The limit depends on the available memory and the method used. UsingBufferedReader
orFiles.lines()
is generally safe for counting lines in very large files. -
Can I count lines in a file with different encodings?
Yes, you can specify the encoding when reading the file, especially when usingFiles.lines()
orFileUtils.readLines()
. This allows you to handle files with various character encodings.