How to Convert Collection to List in Java
- Use a Constructor to Convert Collection Into List in Java
- Use Built-In Functions to Convert Collection Into List in Java
-
Use the
for
Loop to Convert Collection Into List in Java -
Use
collect
to Convert Collection to List in Java
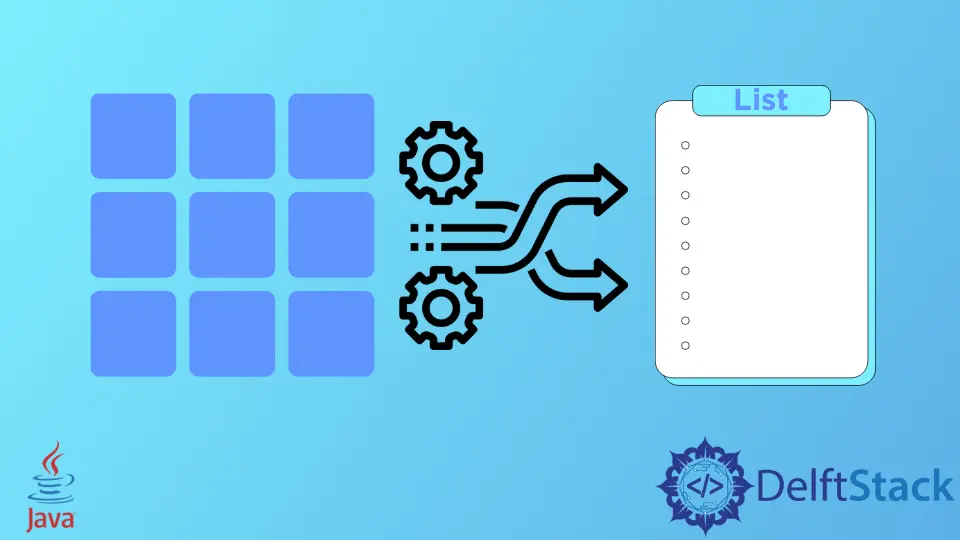
This article will introduce and discuss the different methods of converting a collection into a list in Java.
Use a Constructor to Convert Collection Into List in Java
We can use the constructor function and provide the collection we want to convert in the parameter.
Syntax:
List<Generic> listname = new ArrayList<>(collectionName);
Example:
import java.util.*;
public class ExpHStoLL {
public static void main(String[] args) {
Set<String> hs = new HashSet<String>();
// Adding names in hs
hs.add("Dhruv");
hs.add("Sahil");
hs.add("Ajay");
System.out.println("Hash Set Collection :" + hs);
// Using constructor of hs for linked list
List<String> MyList = new LinkedList<String>(hs);
System.out.println("\nLinked List after conversion: " + MyList);
}
}
Output:
Hash Set Collection :[Dhruv, Sahil, Ajay]
Linked List after conversion: [Dhruv, Sahil, Ajay]
Here, we have created a hash set of names and then used the constructor hs
to create a list from the hash set.
Use Built-In Functions to Convert Collection Into List in Java
There are two built-in methods to convert a collection into a list in the Array and Collections classes.
Use asList
to Convert Collection Into List in Java
The Array class provides the asList()
method to convert an array collection into a list.
Syntax:
List<Generic> MyList = Arrays.asList(ArrayName);
Example:
import java.util.*;
public class ExpArrtoL {
public static void main(String args[]) {
String[] MyArray = {"Dhruv", "Sahil", "Ajay"};
System.out.println("My Array: " + Arrays.toString(MyArray));
// Using asList function
List<String> MyList = Arrays.asList(MyArray);
System.out.println("List using asList(): " + MyList);
}
}
Output:
My Array: [Dhruv, Sahil, Ajay]
List using asList(): [Dhruv, Sahil, Ajay]
In the code above, we created an array and then used the asList()
function with the array name MyArray
as its argument to convert the collection into a list.
Use addAll
to Convert Collection Into List in Java
addAll()
is a method provided in the collections framework that we can use to convert a collection to a list. The elements from the collection can be specified one by one or as an array.
This is similar to the asList()
method, but this performs better, effectively improving time complexity. We must create both an array and a list before using this method since it requires two parameters to copy items from the collection to the list.
Syntax:
Collections.addAll(ListName, ArrayName);
Example:
import java.util.*;
public class ExpArrtoL {
public static void main(String args[]) {
String[] MyArray = {"Dhruv", "Sahil", "Ajay"};
System.out.println("My Array: " + Arrays.toString(MyArray));
List<String> MyList = new ArrayList<>();
// Using addAll function
Collections.addAll(MyList, MyArray);
System.out.println("List using addAll(): " + MyList);
}
}
Output:
My Array: [Dhruv, Sahil, Ajay]
List using addAll(): [Dhruv, Sahil, Ajay]
Use the for
Loop to Convert Collection Into List in Java
If we don’t want to use a constructor or in-built functions, the for-each
loop can help convert a collection into a list. This method is friendly for beginners as it provides an easy way of conversion.
Example:
import java.util.*;
public class ListUsingForLoop {
public static void main(String[] args) {
Set<String> hs = new HashSet<String>();
hs.add("Dhruv");
hs.add("Sahil");
hs.add("Ajay");
System.out.println("Our HashSet HS:" + hs);
// List of the same size as our HashSet hs
List<String> MyList = new ArrayList<String>(hs.size());
// The loop picks each string of HS and adds it to the List
for (String x : hs) MyList.add(x);
System.out.println("\nList made from HS:" + MyList);
}
}
Output:
Our HashSet HS:[Dhruv, Sahil, Ajay]
List made from HS:[Dhruv, Sahil, Ajay]
Here, we have created a hash set of names and used them for each loop to specify that for each string found in the hash set hs
, we add the string to our list MyList
.
Use collect
to Convert Collection to List in Java
We can also use the stream’s collect()
function to convert a collection into a list and display a result without editing our original collection.
The collect()
method gathers elements from data structures or collections and provides additional logic for manipulating them.
Syntax:
List<Generic> ListName = CollectionName.stream().collect(toList());
Example:
import java.util.*;
import java.util.stream.*;
public class ExpHStoLL {
public static void main(String[] args) {
Set<String> hs = new HashSet<String>();
// Adding names in hs
hs.add("Dhruv");
hs.add("Sahil");
hs.add("Ajay");
System.out.println("Hash Set Collection :" + hs);
List<String> MyList = hs.stream().collect(Collectors.toList());
System.out.println("\nConverted to List: " + MyList);
}
}
Output:
Hash Set Collection :[Dhruv, Sahil, Ajay]
Converted to List: [Dhruv, Sahil, Ajay]
While using the collect()
method of the stream API to convert collections into a list, the copying is done using references.
Since the list is a reference data type, i.e., it stores the reference address of the actual objects or elements, any changes to our list will also change our collection.
The copy list created from the original collection is a shallow copy. This means that removing the list will also remove the element from the original collection.
We can avoid this by creating a deep copy, where the copy of a collection to a list results in a new memory allocation for the list instead of just copying old references.
Related Article - Java List
- How to Split a List Into Chunks in Java
- How to Get First Element From List in Java
- How to Find the Index of an Element in a List Using Java
- How to Filter List in Java
- Differences Between List and Arraylist in Java
- List vs. Array in Java