How to Implement Key Value Pair in Java
- Importance of Implementing Key-Value Pairs in Java
- Methods in Implementing Key-Value Pairs
- Conclusion
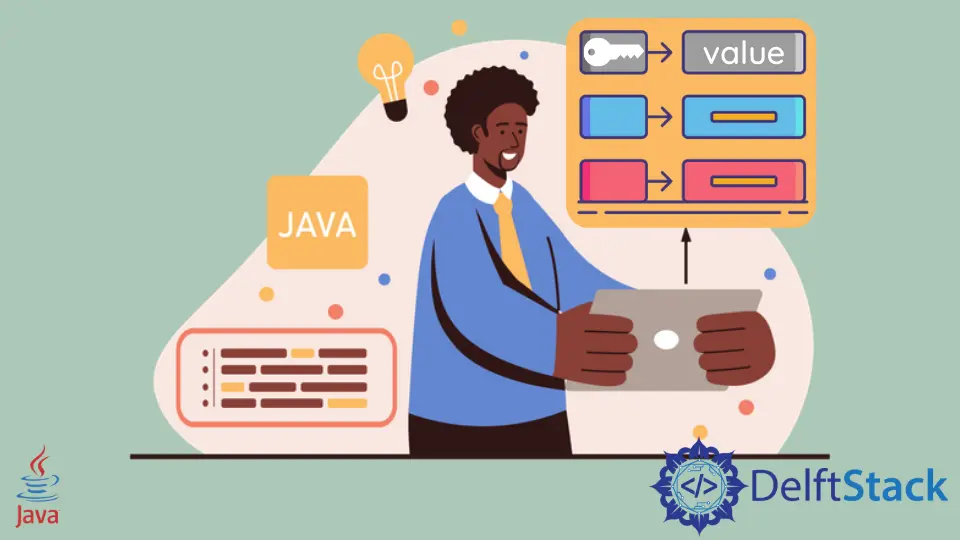
This tutorial introduces how to implement key-value pairs in Java.
In Java, to deal with the key-value pair, the Map
interface and its implementation classes are used. We can use classes such as HashMap
and TreeMap
to store data into the key-value pair.
Apart from these built-in classes, we can create our own class that can hold the key-value pair.
Importance of Implementing Key-Value Pairs in Java
Implementing key-value pairs in Java is crucial for efficient data organization and retrieval. It allows developers to associate a unique identifier (key) with a corresponding value, facilitating quick and direct access to specific pieces of data.
This concept is fundamental in various scenarios, such as managing configuration settings, storing and retrieving data in collections, and creating relationships between different entities in a program. The simplicity and effectiveness of key-value pairs streamline data manipulation and enhance the overall organization and accessibility of information in Java applications.
Methods in Implementing Key-Value Pairs
Using the HashMap
Method
The HashMap
method provides an efficient and flexible way to implement key-value pairs in Java. Its simplicity, combined with fast access times, makes it a powerful tool for managing associative data structures.
By grasping this concept, developers can enhance the organization and retrieval of information within their Java applications.
Code Example:
import java.util.HashMap;
import java.util.Map;
public class KeyValuePairExample {
public static void main(String[] args) {
// Creating a HashMap to store key-value pairs
Map<String, Integer> keyValueMap = new HashMap<>();
// Adding key-value pairs to the HashMap
keyValueMap.put("John", 28);
keyValueMap.put("Alice", 35);
keyValueMap.put("Bob", 42);
// Retrieving values using keys
int johnsAge = keyValueMap.get("John");
int alicesAge = keyValueMap.get("Alice");
// Displaying the results
System.out.println("John's Age: " + johnsAge);
System.out.println("Alice's Age: " + alicesAge);
}
}
Initially, we import the necessary packages, bringing in the HashMap
and Map
classes from the java.util
package, essential for efficient key-value pair implementation. Following that, we create a HashMap
named keyValueMap
, specifying that it will contain string keys and integer values.
We proceed to add key-value pairs to this map
, where each key serves as a unique identifier (in this case, names), and the associated values represent relevant information, such as ages. Utilizing the get
method, we retrieve specific values based on their corresponding keys, exemplified by fetching the ages of individuals named John
and Alice
.
Output:
We showcase the results by displaying the ages associated with the keys John
and Alice
on the console. As we’ve seen in our example, implementing key-value pairs using HashMap
is straightforward and offers a concise way to handle data relationships.
Using the TreeMap
Method
The TreeMap
method provides a sorted approach to implementing key-value pairs in Java. The TreeMap
class, part of the Java Collections Framework
, is a valuable tool that brings a sorted order to these pairs.
Its ability to maintain order based on keys makes it advantageous in scenarios where sorting is essential. By understanding and incorporating TreeMap
into your Java applications, you gain a powerful tool for managing and retrieving data in a sorted manner.
Code Example:
import java.util.Map;
import java.util.TreeMap;
public class TreeMapExample {
public static void main(String[] args) {
// Creating a TreeMap to store key-value pairs
TreeMap<String, Integer> keyValueMap = new TreeMap<>();
// Adding key-value pairs to the TreeMap
keyValueMap.put("John", 28);
keyValueMap.put("Alice", 35);
keyValueMap.put("Bob", 42);
// Retrieving values using keys
int johnsAge = keyValueMap.get("John");
int alicesAge = keyValueMap.get("Alice");
// Displaying the results
System.out.println("John's Age: " + johnsAge);
System.out.println("Alice's Age: " + alicesAge);
}
}
Firstly, by importing the java.util
package, we gain access to the TreeMap
class functionalities. This class, along with the Map
interface, forms the foundation for our key-value pair implementation.
Moving on, we create a TreeMap
named keyValueMap
, explicitly stating that it will contain String
keys and Integer
values within the angular brackets (< >
).
As we populate our TreeMap
with key-value pairs, it’s crucial to note that unlike HashMap
, a TreeMap
maintains a sorted order based on the natural ordering of its keys or a custom comparator if provided.
To retrieve values associated with specific keys, we employ the get
method. In this context, we exemplify this process by fetching the ages of individuals identified by the keys John
and Alice
.
Output:
The output will showcase the ages corresponding to the keys John
and Alice
, emphasizing the ordered nature of the TreeMap
. As demonstrated in our example, the TreeMap
method offers a reliable way to handle key-value pairs while ensuring a well-ordered data structure.
Using the LinkedHashMap
Method
The LinkedHashMap
method provides a reliable way to implement key-value pairs in Java, maintaining the order of insertion. This feature can be advantageous in scenarios where the sequence of elements is crucial.
By incorporating LinkedHashMap
into your Java applications, you gain a valuable tool for managing and retrieving data while preserving the order of insertion.
Code Example:
import java.util.LinkedHashMap;
import java.util.Map;
public class LinkedHashMapExample {
public static void main(String[] args) {
// Creating a LinkedHashMap to store key-value pairs
LinkedHashMap<String, Integer> keyValueMap = new LinkedHashMap<>();
// Adding key-value pairs to the LinkedHashMap
keyValueMap.put("John", 28);
keyValueMap.put("Alice", 35);
keyValueMap.put("Bob", 42);
// Retrieving values using keys
int johnsAge = keyValueMap.get("John");
int alicesAge = keyValueMap.get("Alice");
// Displaying the results
System.out.println("John's Age: " + johnsAge);
System.out.println("Alice's Age: " + alicesAge);
}
}
The LinkedHashMap
class, belonging to the java.util
package, is crucial for key-value pair implementation. By importing both Map
and LinkedHashMap
classes, we gain access to the essential functionalities required for this purpose.
In the code, we declare and instantiate a LinkedHashMap
named keyValueMap
with String
keys and Integer
values denoted by the angle brackets (< >
). The distinct feature of LinkedHashMap
lies in its ability to maintain the order of insertion, a valuable trait in scenarios where the sequence of elements holds significance.
Subsequently, we populate our LinkedHashMap
with key-value pairs, and using the get
method, we retrieve values associated with specific keys, exemplified by fetching the ages of John
and Alice
.
Output:
As exemplified in our code, the LinkedHashMap
method offers an effective solution for scenarios where maintaining insertion order is a key requirement.
Using the Hashtable
Method
The Hashtable
method provides a synchronized and thread-safe way to implement key-value pairs in Java. Its classic nature makes it suitable for scenarios where thread safety is crucial.
By incorporating Hashtable
into your Java applications, you gain a reliable tool for managing and retrieving data while ensuring thread safety.
Code Example:
import java.util.Hashtable;
import java.util.Map;
public class HashtableExample {
public static void main(String[] args) {
// Creating a Hashtable to store key-value pairs
Hashtable<String, Integer> keyValueTable = new Hashtable<>();
// Adding key-value pairs to the Hashtable
keyValueTable.put("John", 28);
keyValueTable.put("Alice", 35);
keyValueTable.put("Bob", 42);
// Retrieving values using keys
int johnsAge = keyValueTable.get("John");
int alicesAge = keyValueTable.get("Alice");
// Displaying the results
System.out.println("John's Age: " + johnsAge);
System.out.println("Alice's Age: " + alicesAge);
}
}
Initially, by importing the java.util
package and specifically the Hashtable
and Map
classes.
We declare and instantiate a Hashtable
named keyValueTable
, with explicit specifications that it will have String
keys and Integer
values. As we populate our Hashtable
with key-value pairs, it’s important to note that Hashtable
ensures the uniqueness of keys, and its synchronized nature makes it thread-safe.
Using the get
method, we retrieve values associated with specific keys, demonstrating this process by fetching the ages of individuals identified by the keys John
and Alice
.
Output:
The output will distinctly showcase the ages corresponding to the keys John
and Alice
, highlighting the unique and synchronized features of the Hashtable
method. As exemplified in our code, the Hashtable
method offers an effective solution for scenarios where synchronization and thread safety are paramount considerations.
Using the Properties
Method
The Properties
method provides a concise and effective way to implement key-value pairs in Java, particularly for configuration settings. Its simplicity makes it an excellent choice for scenarios where you need to store and retrieve key-value pairs without the complexity of other data structures.
It is often used for reading and writing configuration files, making it a versatile tool for storing and retrieving key-value pairs. By using the Properties
class, you gain a versatile tool for managing configuration settings and other key-value data in your Java applications.
Code Example:
import java.util.Properties;
public class PropertiesExample {
public static void main(String[] args) {
// Creating a Properties object to store key-value pairs
Properties properties = new Properties();
// Adding key-value pairs to the Properties object
properties.setProperty("Name", "John Doe");
properties.setProperty("Age", "30");
properties.setProperty("Location", "Cityville");
// Retrieving values using keys
String name = properties.getProperty("Name");
String age = properties.getProperty("Age");
// Displaying the results
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
Initially, by importing the Properties
class from the Java standard library, we gain access to its functionalities. Then we create a Properties
object, named properties
, which acts as our container for key-value pairs.
As we populate this object using the setProperty
method, each call adds or updates a key-value pair in a straightforward manner. To retrieve values associated with specific keys, we use the getProperty
method, exemplified here by fetching the name and age.
Output:
The output will distinctly showcase the values corresponding to the keys Name
and Age
, highlighting the simplicity and effectiveness of the Properties
method for managing key-value pairs. As demonstrated in our example, the Properties
method offers an efficient solution for scenarios where simplicity and ease of use are essential.
Using the EnumMap
Method
The EnumMap
method provides an efficient and type-safe way to implement key-value pairs in Java, especially when dealing with enums
. Its specialization for enums
makes it a precise and performant choice for scenarios where keys are predefined constants.
By utilizing EnumMap
, developers can create well-structured and type-safe mappings, enhancing the readability and reliability of their code.
Code Example:
import java.util.EnumMap;
public class EnumMapExample {
// Enum representing days of the week
enum DayOfWeek { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY }
public static void main(String[] args) {
// Creating an EnumMap to store key-value pairs with enum keys
EnumMap<DayOfWeek, String> dayOfWeekMap = new EnumMap<>(DayOfWeek.class);
// Adding key-value pairs to the EnumMap
dayOfWeekMap.put(DayOfWeek.MONDAY, "Start of the week");
dayOfWeekMap.put(DayOfWeek.TUESDAY, "Second day");
dayOfWeekMap.put(DayOfWeek.WEDNESDAY, "Midweek");
// Retrieving values using enum keys
String mondayDescription = dayOfWeekMap.get(DayOfWeek.MONDAY);
String wednesdayDescription = dayOfWeekMap.get(DayOfWeek.WEDNESDAY);
// Displaying the results
System.out.println("Monday: " + mondayDescription);
System.out.println("Wednesday: " + wednesdayDescription);
}
}
We define an enum
DayOfWeek
to represent the days of the week. Enums
provide a concise way to represent a fixed set of constants, offering clarity in code.
Next, we declare and instantiate an EnumMap
named dayOfWeekMap
, tailored to use DayOfWeek
enum
keys and String
values. The construction of the EnumMap
involves specifying the enum
class, ensuring a clear mapping.
As we populate our EnumMap
with key-value pairs, the enum
constants act as keys, and their corresponding descriptions as values, creating a structured association. Using enum
constants as keys, we retrieve values from the EnumMap
through the straightforward get
method, showcasing the simplicity of accessing values based on enum
keys.
Output:
The output will distinctly showcase the descriptions corresponding to the enum
keys MONDAY
and WEDNESDAY
, emphasizing the clarity and efficiency of this method in managing key-value pairs. As demonstrated in our example, the EnumMap
method offers an effective solution for scenarios where enums
are used as keys in key-value pair structures.
Using the Map.Entry
Method
The Map.Entry
method is a powerful way to interact with individual key-value pairs within a map. It allows developers to access, modify, or remove specific entries, providing fine-grained control over the map’s contents.
This concept is crucial for any Java developer working with maps, and mastering it enhances the ability to manipulate and understand data structures efficiently.
Code Example:
import java.util.HashMap;
import java.util.Map;
public class MapEntryExample {
public static void main(String[] args) {
// Creating a Map to store key-value pairs using Map.Entry
Map<String, Integer> keyValueMap = new HashMap<>();
// Adding key-value pairs using Map.Entry
keyValueMap.put("John", 28);
keyValueMap.put("Alice", 35);
keyValueMap.put("Bob", 42);
// Iterating through Map.Entry objects
for (Map.Entry<String, Integer> entry : keyValueMap.entrySet()) {
String key = entry.getKey();
int value = entry.getValue();
System.out.println(key + "'s Age: " + value);
}
}
}
To begin, we create a HashMap
named keyValueMap
designed to store key-value pairs with String
keys and Integer
values. We add key-value pairs to our map using the put
operation, ensuring that each key is uniquely associated with its corresponding value.
We employ a for-each
loop to iterate over the entrySet()
of our map. The entrySet()
method provides a set view of the mappings within the map.
Within each iteration, we retrieve the key and value from the Map.Entry
object, allowing us to print and manipulate these pairs effectively.
Output:
In our example, we showcased how to use Map.Entry
in conjunction with a HashMap
to iterate over key-value pairs and display their values.
Using the AbstractMap.SimpleEntry
Method
The AbstractMap.SimpleEntry
method provides a concise and convenient way to represent key-value pairs in Java. This concept is beneficial when you need to work with individual entries independently of a larger map structure.
By encapsulating key-value pairs into SimpleEntry
objects, developers gain a flexible and efficient mechanism for managing and manipulating data.
Code Example:
import java.util.AbstractMap;
import java.util.Map;
public class SimpleEntryExample {
public static void main(String[] args) {
// Creating key-value pairs using AbstractMap.SimpleEntry
Map.Entry<String, Integer> entry1 = new AbstractMap.SimpleEntry<>("John", 28);
Map.Entry<String, Integer> entry2 = new AbstractMap.SimpleEntry<>("Alice", 35);
// Displaying the results
System.out.println(entry1.getKey() + "'s Age: " + entry1.getValue());
System.out.println(entry2.getKey() + "'s Age: " + entry2.getValue());
}
}
In this implementation, we utilize instances of AbstractMap.SimpleEntry
to create key-value pairs. This class serves as a convenient container, encapsulating both a key and a value.
In the provided example, entry1
signifies the key-value pair for John
and his age of 28, while entry2
represents Alice
and her age of 35.
Moving forward, we proceed to display the results on the console. Leveraging the getKey()
and getValue()
methods from the Map.Entry
interface, we easily retrieve the key and value from each entry.
Output:
As demonstrated in our example, using AbstractMap.SimpleEntry
allows for clear and direct access to the key and value components of each pair, streamlining the handling of key-value data.
Using the AbstractMap.SimpleImmutableEntry
Method
The AbstractMap.SimpleImmutableEntry
method provides a straightforward and effective way to create immutable key-value pairs in Java. This concept is crucial when dealing with scenarios where certain values should remain constant throughout the program’s execution.
By encapsulating key-value pairs into SimpleImmutableEntry
objects, developers can ensure data integrity and prevent unintended modifications.
Code Example:
import java.util.AbstractMap;
import java.util.Map;
public class SimpleImmutableEntryExample {
public static void main(String[] args) {
// Creating immutable key-value pairs using AbstractMap.SimpleImmutableEntry
Map.Entry<String, Integer> immutableEntry = new AbstractMap.SimpleImmutableEntry<>("John", 28);
// Displaying the result
System.out.println(immutableEntry.getKey() + "'s Age: " + immutableEntry.getValue());
}
}
In this implementation, we initiate the creation of an immutable key-value pair by utilizing the AbstractMap.SimpleImmutableEntry
class. This specific class is designed to encapsulate both a key and a value, ensuring that the resulting key-value pair remains unalterable.
In the provided example, the immutableEntry
instance represents the key-value pair for John
and his age of 28. Subsequently, we proceed to showcase the result by printing it to the console.
Leveraging the getKey()
and getValue()
methods from the Map.Entry
interface, we retrieve the key and value from our immutable entry. This serves as a clear demonstration of the immutability feature, emphasizing that the values associated with this key cannot be modified.
Output:
As demonstrated in our example, using AbstractMap.SimpleImmutableEntry
guarantees that the values associated with the key remain immutable, offering a reliable solution for scenarios that demand unalterable key-value relationships.
Using the Map.immutableEntry
Method
The Map.immutableEntry
method provides a concise and efficient way to create immutable key-value pairs in Java. This concept is particularly useful when dealing with scenarios where certain values must remain constant throughout the program’s execution.
By using this method, developers can ensure data integrity and prevent unintended modifications to critical key-value relationships.
Code Example:
import java.util.Map;
public class ImmutableEntryExample {
public static void main(String[] args) {
// Creating an immutable key-value pair using Map.immutableEntry
Map.Entry<String, Integer> immutableEntry = Map.entry("John", 28);
// Displaying the result
System.out.println(immutableEntry.getKey() + "'s Age: " + immutableEntry.getValue());
}
}
The resulting immutableEntry
encapsulates the key-value pair for John
and his age of 28. To showcase the efficacy of the immutable key-value pair, we employ the getKey()
and getValue()
methods from the Map.Entry
interface.
The immutableEntry
object ensures that once set, these values remain unmodifiable.
Output:
This short process highlights the simplicity and reliability of creating and displaying immutable key-value pairs in Java. As illustrated in our example, the resulting immutableEntry
object ensures that the values associated with the key are immutable, making it a powerful tool for scenarios that demand unalterable data associations.
Conclusion
Implementing key-value pairs in Java is fundamental for efficient data organization and retrieval. The use of various methods, including HashMap
, TreeMap
, Hashtable
, Properties, EnumMap
, Map.entry
, AbstractMap.SimpleEntry
, AbstractMap.SimpleImmutableEntry
, and Map.immutableEntry
, provides developers with versatile tools to manage data effectively.
Whether it’s achieving flexibility with HashMap
, maintaining order with TreeMap
, ensuring thread-safety with Hashtable
, managing configurations with Properties
, or utilizing enum
constants with EnumMap
, these methods cater to diverse programming needs. The ability to create immutable key-value pairs further enhances data integrity.
Each method offers a unique approach, allowing developers to choose the most suitable solution based on the specific requirements of their Java applications.