How to Check if String Is Palindrome in Java
- Use Pointers to Check if a String Is a Palindrome in Java
- Reverse the String to Check if a String Is a Palindrome in Java
- Use Recursion to Check if a String Is a Palindrome in Java
- Conclusion
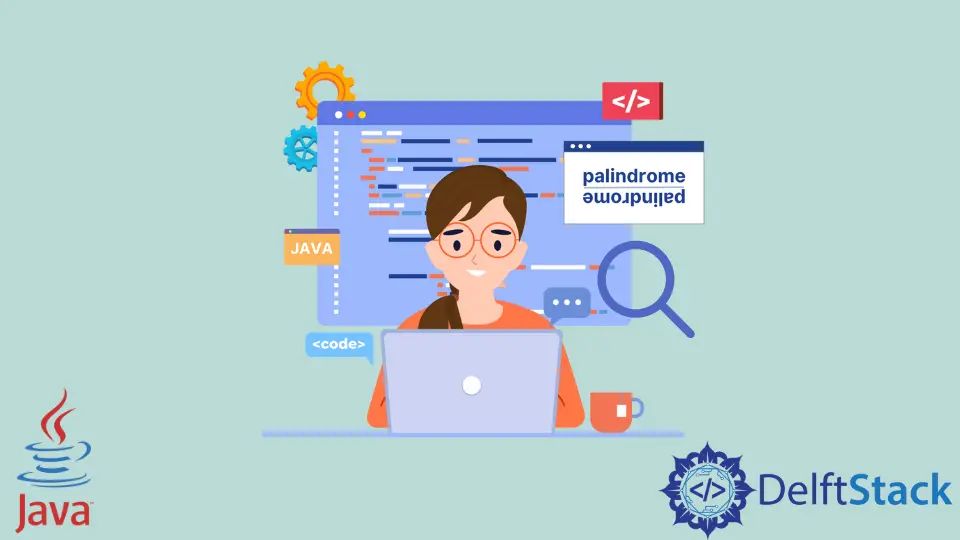
If the characters of a string from right to left are the same as the string’s characters from left to right, then we call it a palindrome
. Strings like ababa
, radar
, and ppqpp
are some examples.
Here, the first character is the same as the last character; the second character is the same as the second last character, etc. In this article, we will look at the various Java programs to check if a string is a palindrome or not.
Use Pointers to Check if a String Is a Palindrome in Java
A very simple idea to check if a string is a palindrome or not is to use two pointers; one point to the start of the string and the other points to the end of the string. Consider the following code.
public class PalProgram {
static boolean PalFunc(String s)
{
// Pointer i pointing to the start and j pointing to the end
int i = 0, j = s.length() - 1;
while (i < j) {
// Check if there is any mis-matching pair
if (s.charAt(i) != s.charAt(j))
return false;
// Update the pointers
i++;
j--;
}
// If no mismatch occurs
return true;
}
public static void main(String[] args) {
String s = "ava";
s = s.toLowerCase();
if (PalFunc(s))
System.out.print("Yes, it is a palindrome.");
else
System.out.print("No, it is not a palindrome.");
}
}
Output:
Yes, it is a palindrome.
Here, inside the PalFunc
function, the first pointer i
will point to the beginning of the string, and the second pointer j
will point to the end of the string, which we have to check if it is a palindrome or not.
Then, we will run a loop until i<j
. At each step, we check if the characters pointed by these two pointers, i
and j
, match or not.
Also, we simultaneously increment i
and decrement j
by one. If the characters don’t match at any step, we return false
notifying the user that the string is not a palindrome.
We are using the toLowerCase()
function in our example. The Java compiler compares two characters based on their ASCII
values.
It means that A == a
will evaluate false
. In this case, the string abA
will not be considered a palindrome in Java, which is not the actual scenario.
It is why we must first convert the string to either uppercase or lowercase before comparison in the for
loop. It is helpful when dealing with palindromes like AVva
, where the characters mix upper and lower cases.
Reverse the String to Check if a String Is a Palindrome in Java
Consider that we have the string aabcvbaa
. Let us first reverse the string. The resultant string will be aabvcbaa
.
The last character of the original string becomes the first character of the reversed string. The second last character of the original string becomes the second character of the reversed string, and so on.
Now, we can compare the two strings character by character to check if the string is a palindrome. If any mismatch occurs, the string is not a palindrome, and we can return false
, notifying the user that the string is not a palindrome.
But, if no mismatch occurs throughout, we can return true
, saying that the string is a palindrome. In this case, we are making a new reversed string instead of using two pointers in the same string (see the demonstration).
Sometimes, we are not allowed to use built-in functions provided in Java. Therefore, we will not use the reverse()
method from Java APIs.
We will write our function to reverse the string.
public class Solution {
static boolean Sol(String s)
{
// reverse the string
StringBuilder reversed_str = new StringBuilder();
char[] newArray = s.toCharArray();
for (int index = newArray.length - 1; index >= 0; index--) {
reversed_str.append(newArray[index]);
}
// comparing the original string with the reversed string
return (reversed_str.toString()).equals(s);
}
public static void main(String[] args) {
String s = "raceCAR";
// Convert the string to the lowercase
s = s.toLowerCase();
if (Sol(s))
System.out.print("Yes, this string is a palindrome.");
else
System.out.print("No, it isn't a palindrome.");
}
}
Output:
Yes, this string is a palindrome.
Let us quickly see what is happening inside the Sol
function. We first change the string to an array and then use this to reverse the string.
Then, we compare the reversed string with the original string letter by letter.
StringBuilder
class: The string class in Java creates immutable strings, meaning unchangeable strings. Here, we want to create a string,reversed_str
, which is mutable to append characters to it. TheStringBuilder
class in Java helps us to create mutable strings.toCharArray
method: Since we want to compare the original and the reversed string character by character, we use thetoCharArray()
method to convert the string to a series of characters. We store the result in the arraynewArray
.append()
method: After changing the original string to a character array, use it to make the reversed string. For this, we traverse the character array from the end and keep adding the characters in the stringreversed_str
using theappend()
method.toString()
method: We change it to a string again using thetoString()
method after making the reversed string. We do this because we can compare two strings simply using theequals()
method.
reversed_str
, and the equals()
method compares strings, not character sequences.equals()
method: At last, we compare the original strings
with the reversed stringreversed_str
. To do this, we can use theequals()
method, which returnstrue
if all characters of the string match.
We can achieve the same with ease if we use the reverse()
method from Java APIs - StringBuilder
and StringBuffer
, as shown below.
// Check if a string is a palindrome
// Java program
public class Solution {
static boolean Sol(String s)
{ // Using the stringbuilder API
StringBuilder newString = new StringBuilder(s);
StringBuilder rev_str = newString.reverse();
return (rev_str.toString()).equals(s);
}
public static void main(String[] args) {
String s = "raceCAR";
// Convert the string to the lowercase
s = s.toLowerCase();
if (Sol(s))
System.out.print("Yes, it is a palindrome.");
else
System.out.print("No, it is not a palindrome.");
}
}
Output:
Yes, it is a palindrome.
Note that when we use the StringBuilder
API, we need not create character arrays or reverse the string using a for
loop. This method is clean and simple.
To know more about the StringBuilder
class, refer to this documentation.
We can also use the StringBuilder
API, as shown below.
public class CheckPalindrome {
static boolean Sol(String s)
{ // Using the stringbuffer API
StringBuffer str = new StringBuffer(s);
StringBuffer rev_str = str.reverse();
return (rev_str.toString()).equals(s);
}
public static void main(String[] args) {
String s = "raceCAR";
// Convert the string to the lowercase
s = s.toLowerCase();
if (Sol(s))
System.out.print("Yes, it is a palindrome.");
else
System.out.print("No, it is not a palindrome.");
}
}
Output:
Yes, it is a palindrome.
You might wonder what makes the StringBuilder
and StringBuffer
classes different because the code looks identical.
The StringBuffer
class allows only one thread to call this method at a time. It is synchronized.
On the other hand, the StringBuilder
method can be called by more than a single thread simultaneously. It is non-synchronized.
However, the StringBuilder
class is more efficient than the StringBuffer
class. To know more about the StringBuffer
class, refer to this documentation.
Use Recursion to Check if a String Is a Palindrome in Java
We can recursively call the Sol
function to check if a string is a palindrome. The basic idea is to use recursion for iterating over the string.
public class Solution {
static boolean Sol(String s)
{
s = s.toLowerCase();
return RecursePal(s, 0, s.length() - 1);
}
static boolean RecursePal(String s, int f, int b) {
if (f == b) {
return true;
}
if ((s.charAt(f)) != (s.charAt(b))) {
return false;
}
if (f < b + 1) {
return RecursePal(s, f + 1, b - 1);
}
return true;
}
public static void main(String[] args) {
String s = "raceCAR";
// Convert the string to the lowercase
s = s.toLowerCase();
if (Sol(s))
System.out.print("Yes");
else
System.out.print("No");
}
}
Output:
Yes
Here, we define a function, RecursePal
. We pass the string s
, the index of the first character as f
and the index of the last character as b
as arguments.
Then, we check if the character at f
is the same as at b
. If yes, we return true
.
Otherwise, we return false
. Lastly, we again call the RecursePal
function to repeat this process for the entire string.
Each time we recursively call this function, we increment the f
index and decrement the b
index by one.
Conclusion
In this tutorial, we saw the different ways in Java to check if a string is a Palindrome or not.
We learned how to use two-pointers to loop through the string both ways. We also saw how to check if a string is a palindrome by reversing the string and using recursion in Java.