How to Check if String Contains Numbers in Java
- Using the Regular Expressions Method to Check if String Contains Numbers in Java
-
Using
Character.isDigit()
to Check if String Contains Numbers in Java -
Using the
Stream API
Method to Check if String Contains Numbers in Java -
Using the
Pattern
andMatcher
Methods to Check if String Contains Numbers in Java -
Using
replaceAll()
to Check if String Contains Numbers in Java - Conclusion
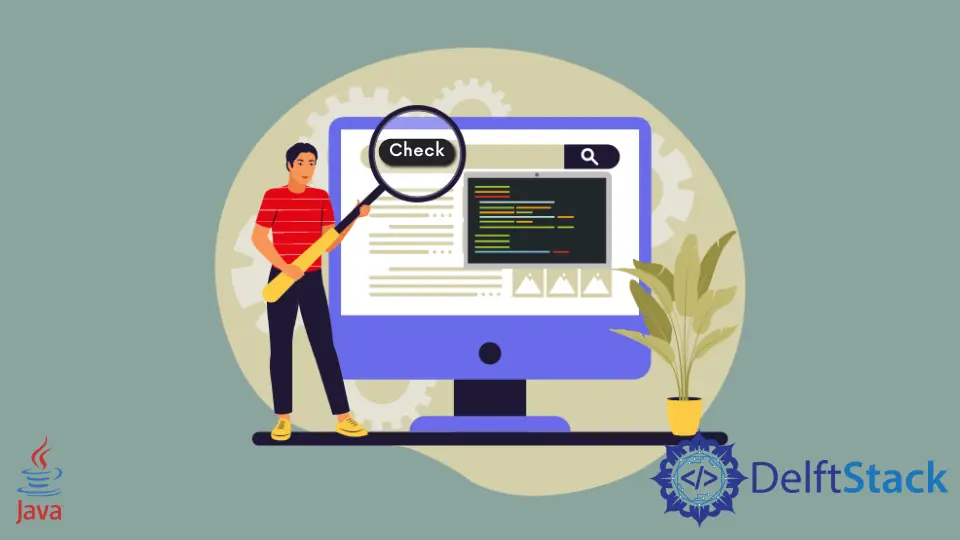
This article discusses the various ways to check if string contains a number in Java.
Checking if a string contains specific elements is crucial in Java for various tasks. This verification enables programmers to validate user inputs, ensuring they meet expected formats or constraints.
It plays a pivotal role in data validation, where applications need to confirm the presence of certain patterns, such as numeric values or specific characters. String containment checks are fundamental for parsing and extracting relevant information from strings, enhancing data processing capabilities.
Additionally, these checks are essential in decision-making processes, allowing developers to take appropriate actions based on the content of strings.
Using the Regular Expressions Method to Check if String Contains Numbers in Java
Using regular expressions to check if a string contains a number in Java offers unparalleled flexibility and conciseness.
Regular expressions provide a powerful and expressive way to define complex patterns, making it ideal for nuanced numeric checks. Unlike other methods, regex allows for precise customization, accommodating variations in number formats or positions within the string.
It simplifies code, offering a compact solution that adapts to diverse scenarios. Regular expressions enhance readability, reduce code length, and efficiently handle intricate numeric identification tasks, making them a preferred choice for robust and adaptable string analysis in Java.
Let’s dive into a comprehensive Java code example that employs regular expressions to address this challenge:
public class StringContainsNumbersExample {
public static boolean containsNumbersRegex(String input) {
return input.matches(".*\\d.*");
}
public static void main(String[] args) {
String sampleString = "Hello123World";
if (containsNumbersRegex(sampleString)) {
System.out.println("The string contains numbers.");
} else {
System.out.println("No numbers found in the string.");
}
}
}
In our solution, the pivotal component is the containsNumbersRegex
method, designed to assess whether a given string harbors any numeric digits. The method leverages a regular expression, .*\\d.*
, to achieve this evaluation.
Breaking down this expression, .*
matches any character (excluding newline) zero or more times, \\d
matches any digit (0-9
), and .*
follows, matching any character zero or more times again.
The matches
method, integral to our approach, is invoked on the input string, subjecting it to a comparison against the specified regular expression. Its return value is true
if the entire string aligns with the pattern, signifying the presence of numeric digits.
In the main
method, we demonstrate the practical application of our solution. By initializing a sample string, sampleString
, containing a mixture of alphabetic characters and numbers, we invoke the containsNumbersRegex
method on this string.
If the method returns true
, we communicate the existence of numbers in the string; otherwise, we convey their absence. This methodological breakdown provides a clear understanding of how the code operates and underscores its efficiency in discerning numeric content within a string.
For the given sampleString
(Hello123World
), the output of the program would be:
The string contains numbers.
The beauty of this approach lies in its simplicity and conciseness, providing an elegant solution to a common programming challenge.
Using Character.isDigit()
to Check if String Contains Numbers in Java
Utilizing the Character.isDigit()
method in Java to check if a string contains a number offers a direct, lightweight, and efficient approach. It simplifies code, providing a clear and concise way to verify the presence of numeric digits without the need for complex iterations or regular expressions.
This method enhances code readability, making it an ideal choice for straightforward numeric checks within strings. Its simplicity and minimal overhead make it a pragmatic choice for scenarios where a quick and precise determination of numeric content is required, contributing to efficient and concise Java programming.
Let’s dive into a complete Java code example that employs the Character.isDigit()
method to check if a string contains numeric digits:
public class StringContainsNumbersExample {
public static boolean containsNumbersIsDigit(String input) {
for (char c : input.toCharArray()) {
if (Character.isDigit(c)) {
return true;
}
}
return false;
}
public static void main(String[] args) {
String sampleString = "Hello123World";
if (containsNumbersIsDigit(sampleString)) {
System.out.println("The string contains numbers.");
} else {
System.out.println("No numbers found in the string.");
}
}
}
The essence of our solution lies in the containsNumbersIsDigit
method, which utilizes the Character.isDigit()
method to inspect each character in the input string.
In this method, we employ a for-each
loop to iterate over each character in the string. For each character (c
), we utilize the Character.isDigit()
method to determine if it represents a numeric digit.
If at least one character is a digit, the method returns true
, indicating the presence of numeric digits. Otherwise, if no digit is found after iterating through the entire string, the method returns false
.
In the main
method, we demonstrate the practical application of our solution by creating a sample string (sampleString
) containing a mix of alphabetic characters and numbers. The containsNumbersIsDigit
method is then invoked, and based on its result, we print a message indicating the presence or absence of numeric digits.
For the given sampleString
(Hello123World
), the output of the program would be:
The string contains numbers.
This method provides a clear and concise way to achieve our goal, showcasing the elegance of straightforward solutions in Java programming. As you navigate the intricacies of string manipulation, incorporating methods like Character.isDigit()
can enhance both the readability and effectiveness of your code.
Using the Stream API
Method to Check if String Contains Numbers in Java
Leveraging the Stream API to check if a string contains a number in Java introduces a declarative and functional paradigm.
The Stream API provides a concise and expressive approach, enhancing code readability and maintainability. It encapsulates the complexity of iteration, filtering, and matching, promoting a streamlined solution.
The fluent and declarative nature of the Stream API aligns with modern Java programming practices, offering an elegant alternative to imperative loop-based methods. This simplifies the code structure, promotes better code organization, and seamlessly integrates with other stream operations, making it a preferred choice for efficient and expressive numeric checks within strings.
Let’s dive into a complete Java code example that harnesses the power of the Stream API to address the task at hand:
import java.util.stream.IntStream;
public class StringContainsNumbersExample {
public static boolean containsNumbersStream(String input) {
return input.chars().anyMatch(Character::isDigit);
}
public static void main(String[] args) {
String sampleString = "Hello123World";
if (containsNumbersStream(sampleString)) {
System.out.println("The string contains numbers.");
} else {
System.out.println("No numbers found in the string.");
}
}
}
The central component is the containsNumbersStream
method, leveraging the Stream API to assess whether a string contains numeric digits.
This method employs the chars()
method on the input string, returning an IntStream
of the character values. We then utilize the anyMatch
function to determine if any character is a digit.
In the main
method, a sample string (sampleString
) comprising both alphabetic characters and numbers is created. The containsNumbersStream
method is then invoked on this string.
The resulting boolean
value determines whether the string contains numeric digits.
This approach is streamlined and expressive. The chars()
method efficiently converts the string into an IntStream
, and the anyMatch
function succinctly checks for the presence of numeric digits.
It showcases the elegance of the Stream API in handling complex operations on collections.
For the given sampleString
(Hello123World
), the output of the program would be:
The string contains numbers.
In this exploration, we’ve witnessed the power of the Stream API in addressing the task of identifying numeric digits in a string. The concise and expressive nature of the code demonstrates the effectiveness of leveraging modern Java features.
Using the Pattern
and Matcher
Methods to Check if String Contains Numbers in Java
Employing the Pattern
and Matcher
methods to check if a string contains a number in Java delivers a robust and customizable solution. It harnesses the power of regular expressions, offering a versatile approach to pattern matching within strings.
In Java, working with regular expressions involves two main components: Pattern
and Matcher
.
To create a Pattern
, you use the following syntax:
import java.util.regex.Pattern;
Pattern pattern = Pattern.compile(String regex);
Subsequently, you use a Matcher
to apply the pattern to a specific input using the following syntax:
import java.util.regex.Matcher;
// Create a Matcher for the pattern and the input string
Matcher matcher = pattern.matcher(CharSequence input);
The resulting Matcher
object provides various methods such as find()
, matches()
, and group()
to perform matching operations and retrieve matched portions.
Pattern
defines the regular expression, while Matcher
applies this pattern to a given input, facilitating versatile matching operations in Java.
This method allows for intricate pattern definitions, accommodating various numeric formats and positions. The Pattern
and Matcher
combination promotes code modularity and reusability, facilitating the creation of complex matching conditions.
Its adaptability and precision make it superior for scenarios where specific numeric patterns need validation, providing a comprehensive and efficient means of identifying numbers in strings compared to simpler methods.
Let’s delve into a complete Java code example that utilizes the Pattern
and Matcher
classes to ascertain the presence of numeric digits within a string:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class StringContainsNumbersExample {
public static boolean containsNumbersPatternMatcher(String input) {
Pattern pattern = Pattern.compile(".*\\d.*");
Matcher matcher = pattern.matcher(input);
return matcher.matches();
}
public static void main(String[] args) {
String sampleString = "Hello123World";
if (containsNumbersPatternMatcher(sampleString)) {
System.out.println("The string contains numbers.");
} else {
System.out.println("No numbers found in the string.");
}
}
}
The crux of our solution lies in the containsNumbersPatternMatcher
method, which utilizes the Pattern
and Matcher
classes to identify numeric digits within a string.
In this method, we begin by creating a Pattern
object using the compile
method.
The regular expression .*\\d.*
is specified, where .*
matches any character (except for a newline character) zero or more times, and \\d
matches any digit (0-9
). The Pattern
now represents the pattern we want to search for in the input string.
Next, we create a Matcher
object by invoking the matcher
method on the Pattern
instance. This Matcher
is then used to apply the pattern to the input string, and we check if the entire string matches the specified pattern using the matches
method.
In the main
method, we demonstrate the usage of our solution by creating a sample string (sampleString
) containing a mix of alphabetic characters and numbers. The containsNumbersPatternMatcher
method is then invoked, and based on its result, we print a message indicating the presence or absence of numeric digits.
For the given sampleString
(Hello123World
), the output of the program would be:
The string contains numbers.
This approach provides flexibility in defining custom patterns, making it a versatile tool for various string analysis tasks.
Using replaceAll()
to Check if String Contains Numbers in Java
Employing the replaceAll()
method in Java to check if a string contains a number simplifies the identification process. By leveraging regular expressions to replace non-numeric characters with an empty string, it provides a concise and readable solution.
The replaceAll()
method in Java is used to replace all occurrences of a specified substring or match with another string. Here’s the syntax:
String newString = originalString.replaceAll(String regex, String replacement);
In the replaceAll()
method syntax, originalString
refers to the string where replacements occur. The regex
parameter is a regular expression pattern identifying the substring or match to replace, and replacement
is the string that substitutes each matched occurrence.
This method efficiently updates strings by replacing all instances of the specified pattern with the designated replacement string. Understanding these parameters allows developers to tailor string transformations based on defined patterns, enhancing flexibility and precision in Java programming.
This method streamlines code, offering a straightforward way to focus on numeric patterns within strings. The replaceAll()
approach is particularly useful when aiming for simplicity and when the emphasis is on pattern manipulation, making it a convenient choice for scenarios where a clean and efficient numeric check is paramount in Java programming.
Let’s delve into a complete Java code example that utilizes the replaceAll()
method to check if a string contains numeric digits:
public class StringContainsNumbersExample {
public static boolean containsNumbersReplaceAll(String input) {
return !input.replaceAll("\\D", "").isEmpty();
}
public static void main(String[] args) {
String sampleString = "Hello123World";
if (containsNumbersReplaceAll(sampleString)) {
System.out.println("The string contains numbers.");
} else {
System.out.println("No numbers found in the string.");
}
}
}
The core of our solution lies in the containsNumbersReplaceAll
method, which utilizes the replaceAll()
method to efficiently identify numeric digits within a string.
In this method, we invoke replaceAll("\\D", "")
on the input string.
The regular expression \D
targets any non-digit character in the string and replaces it with an empty string. Consequently, what remains after this operation are only the numeric digits.
The result of the replaceAll()
operation is then checked for emptiness using the isEmpty()
method.
If there are no numeric digits in the string, the method returns true
, indicating the absence of numbers. Conversely, if numeric digits are present, the method returns false
.
In the main
method, we showcase the functionality of our solution by creating a sample string (sampleString
) containing a mix of alphabetic characters and numbers. The containsNumbersReplaceAll
method is then invoked, and based on its result, we print a message indicating the presence or absence of numeric digits.
For the given sampleString
(Hello123World
), the output of the program would be:
The string contains numbers.
In this exploration, we’ve harnessed the power of the replaceAll()
method to efficiently identify numeric digits in a Java string. This approach demonstrates the elegance of leveraging regular expressions to streamline complex string manipulations.
Conclusion
In conclusion, determining if a string contains numbers in Java can be approached through various methods, each serving specific needs.
Regular expressions offer flexibility and precision, accommodating complex patterns. The Stream API provides a modern, concise, and functional approach, enhancing readability.
Pattern
and Matcher
bring versatility, especially in intricate pattern-matching scenarios. The Character.isDigit()
method offers simplicity for straightforward numeric checks.
replaceAll()
provides a clean way to manipulate strings based on defined patterns.
The choice among these methods depends on the specific requirements, emphasizing the importance of selecting the most suitable approach for efficient and effective string analysis in Java programming.